Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial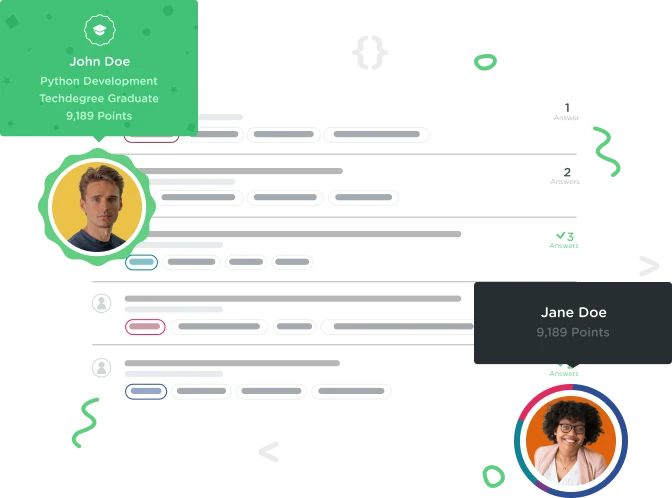

David Sampimon
12,026 Pointsjava.util.NoSuchElementException: No line found (Look around Scanner.java line 1540)
While I can succesfully run my code and pass the 'pseudo-tests' in IntelliJ locally, I receive the below error when checking the work in code challenge:
Bummer! java.util.NoSuchElementException: No line found (Look around Scanner.java line 1540)
It seems to be related to using scanner for user input. Who can please explain to me why my code runs locally and not when checking the code challenge. Also, suggestions for how to fix are more than welcome.
Thanks!
package com.teamtreehouse;
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
// write your code here
Prompter prompter = new Prompter();
Scanner scan = new Scanner(System.in);
System.out.println("Please enter the story, format placeholders with surrounding double underscore: __example__ ");
String story = scan.nextLine();
Template tmpl = new Template(story);
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.*;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Scanner;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
/* // Fake results used for testing
List<String> fakeResults = Arrays.asList(
"friend",
"talented",
"java programmer",
"high five");
*/
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem prompting for words");
e.printStackTrace();
System.exit(0);
}
// System.out.println(tmpl.render(results));
System.out.printf("Your TreeStory:%n%n%s", tmpl.render(results));
}
/**
* Prompts user for each of the blanks
*
* @param tmpl The compiled template
* @return
* @throws IOException
*/
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
/**
* Prompts the user for the answer to the fill in the blank. Value is guaranteed to be not in the censored words list.
*
* @param phrase The word that the user should be prompted. eg: adjective, proper noun, name
* @return What the user responded
*/
public String promptForWord(String phrase) {
String word;
do {
System.out.printf("Please enter %s: ",
phrase);
Scanner scan = new Scanner(System.in);
word = scan.nextLine();
} while (mCensoredWords.contains(word) == true);
return word;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
4 Answers
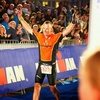
Steve Hunter
57,712 PointsHi David,
The error, java.util.NoSuchElementException
is often thrown in the context of a Scanner
when you call nextLine()
and there's no next line. You can cover this by checking for a next line before asking for it. Try something like:
if(scan.hasNextLine()){
String story = scan.nextLine();
}
That might cover off the eventuality of a null next line. The issue may not be this, but I have come across this a number of times.
Let me know what worked in the end.
Steve.

David Sampimon
12,026 PointsThank you for your suggestion Steve! Unfortunally I couldnt resolve the code challenge by using the scanner class. What I ended up doing was ditching the scanner and switching to a "BufferedReader(new InputStreamReader(System.in))" to poll for user input. With this approach I could finish the code challenge.
So unfortunatly it is still unclear for me why I could finish the pseudo tests locally and not in the browser.
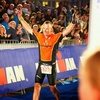
Steve Hunter
57,712 PointsHi David,
I got your code to 'work', i.e. not throw the error this thread relates to.
Two tweaks needed.
If you chase the error, you can fix the code. Your detailed error, and program output, is:
Please enter the story, format placeholders with surrounding double underscore: __example__
Please enter first prompt: java.util.NoSuchElementException: No line found
at java.util.Scanner.nextLine(Scanner.java:1540)
at com.teamtreehouse.Prompter.promptForWord(Prompter.java:89)
at com.teamtreehouse.Prompter.promptForWords(Prompter.java:69)
at com.teamtreehouse.Prompter.run(Prompter.java:49)
at com.teamtreehouse.Main.main(Main.java:15)
at JavaTester.run(JavaTester.java:77)
at JavaTester.main(JavaTester.java:39)
The stuff about Scanner.java:1540
can be ignored - I don't fancy rewriting that lot!! However, the earlier errors are in your code. The trace goes right back to the start of execution, in reverse order, so start with the first reference to your code, i.e. Prompter.java:89
. That's around the use of scan.nextLine()
I referred to in my earlier answer.
Protect that in the way I suggested, using an if
to test for hasNextLine()
. That throws another problem, as it's possible that word
will never be assigned anything as it is hidden within the if
conditional, so initialize that to a blank string where you declare it. The whole method looks like:
public String promptForWord(String phrase) {
String word = "";
do {
System.out.printf("Please enter %s: ",
phrase);
Scanner scan = new Scanner(System.in);
if(scan.hasNextLine()){
word = scan.nextLine();
}
} while (mCensoredWords.contains(word)); // no need for == true
return word;
}
As I said, this fixes the error you saw and enables the code to work within this environment using the Scanner
class. The code doesn't satisfy the code challenge for whatever reason but I'm sure you can figure that out. It doesn't like how your story is output/presented, for some reason.
I hope that helps,
Steve.

David Sampimon
12,026 PointsThanks Steve! Now I am puzzled why it doesnt like my output, looks good in IntelliJ! I'll dive into it.
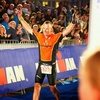
Steve Hunter
57,712 PointsOK. Looking at your IDE and code isn't the issue. Look at the output from your code in the challenge. Use the Preview button to see what it is doing. That may lead you to understanding where your code isn't behaving itself. It may be that the challenge tests are expecting the code to use a stream reader rather than a scanner. Their outputs may be slightly different which is throwing the tests out - I don't know what those tests are, unfortunately.
However, if I had £10 for every post that states "it works fine in the IDE- the challenge must be wrong", I'd be a rich man! I'm not, unfortunately!
I do think that the expected use of the stream reader is part of the issue you're facing. You can't use the console object in the IDE, easily, (you can, but it's a chore - I wrote a long post about it somewhere within these pages) so switching to a scanner makes sense. But the reverse process may cause the problem; I don't know.
Let me know how you get on - I'd be interested to get to the bottom of what's causing the compiler to work differently than expected. Chances are, it isn't, but the difference needs articulating, for sure.
Steve.