Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial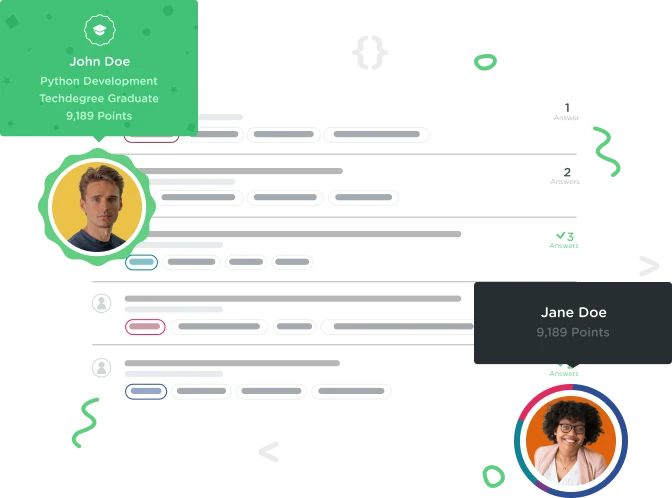

Anthony Renwick
3,609 Pointsjava.util.NoSuchElementException: No line found (Look around Scanner.java line 1540)
I have been having issues trying to work around this issue involving scanner & .nextLine(). But to no avail. I have looked in the Community Forum but I have not found something that could help me towards the right direction. This task has been quite a doozy so far so, any advice would be helpful. Thank you.
package com.teamtreehouse;
import java.io.IOException;
public class Main {
public static void main(String[] args) {
Prompter prompter = new Prompter();
String story = prompter.newPrompt();
Template tmpl = new Template(story);
try {
prompter.promptForWord(story);
} catch (IOException e) {
e.printStackTrace();
}
prompter.run(tmpl);
}
}
package com.teamtreehouse;
import java.io.IOException;
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Scanner;
import java.util.Set;
public class Prompter {
private BufferedReader mReader;
private Set<String> mCensoredWords;
public Prompter() {
mReader = new BufferedReader(new InputStreamReader(System.in));
loadCensoredWords();
}
private void loadCensoredWords() {
mCensoredWords = new HashSet<String>();
Path file = Paths.get("resources", "censored_words.txt");
List<String> words = null;
try {
words = Files.readAllLines(file);
} catch (IOException e) {
System.out.println("Couldn't load censored words");
e.printStackTrace();
}
mCensoredWords.addAll(words);
}
public void run(Template tmpl) {
List<String> results = null;
try {
results = promptForWords(tmpl);
} catch (IOException e) {
System.out.println("There was a problem...");
e.printStackTrace();
System.exit(0);
}
System.out.printf("%nYour TreeStory: %n%s", tmpl.render(results));
}
public String newPrompt() {
Scanner scanner = new Scanner(System.in);
if(scanner.hasNextLine()){
String story = scanner.nextLine();
}
System.out.printf("Enter a prompt: %n");
return scanner.nextLine();
}
public List<String> promptForWords(Template tmpl) throws IOException {
List<String> words = new ArrayList<String>();
for (String phrase : tmpl.getPlaceHolders()) {
String word = promptForWord(phrase);
words.add(word);
}
return words;
}
public String promptForWord(String phrase) throws IOException {
boolean isCensored;
String userInput;
do {
isCensored = false;
Scanner in = new Scanner(System.in);
if(in.hasNextLine()){
String story = in.nextLine();
}
System.out.printf("Enter a word that is a(n) %s%n", phrase);
userInput = in.nextLine();
for (String censored : mCensoredWords) {
if (userInput.equalsIgnoreCase(censored)) {
isCensored = true;
System.out.println("Try a less offensive word");
}
}
} while (isCensored);
return userInput;
}
}
# This is essentially what I am testing
1. The user is prompted for a new string template (the one with the double underscores in it).
a. The prompter class has a new method that prompts for the story template, and that method is called.
2. The user is then prompted for each word that has been double underscored.
a. The answer is checked to see if it is contained in the censored words.
User is continually prompted until they enter a valid word
3. The user is presented with the completed story
1 Answer
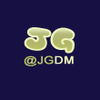
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Anthony,
Apologies noone has answered your query so far. I know how frustrating it can be hitting roadblocks with your code. Thanks for reaching out. :)
It's been some time since I worked on this project, but I'm getting the sense it involves pasting code you've been working on in your IDE, right?
It's difficult therefore for me to provide a solution. Did the responses on your stack overflow thread work out for you?
Anthony Renwick
3,609 PointsAnthony Renwick
3,609 PointsHey Jonathan,
First off, thank you for responding. I really do appreciate it.
Well, to answer your first question, it involves code that we got from the Downloads (it's the code in the 'TreeStory' folder, that is inside the local-development-environments folder). I can work on it in my IDE, but it has to output correctly in my 'Finishing TreeStory' objective.
And as far as your second question is concerned, I have gotten a few. But I am still trying to work through them to see which one sticks.
Anthony Renwick
Anthony Renwick
3,609 PointsAnthony Renwick
3,609 PointsHey Jonathan, I did work through what a solution given to me on Stack Overflow. It corrected my stated issue. But now I have a new issue (listed below after my corrected code).
Code (Main.java):
Code (Prompter.java):
New Error:
Any ideas on what may be causing this issue?
Anthony Renwick
3,609 PointsAnthony Renwick
3,609 PointsI made the neccesary correction by deleting 'throws IOException' and adding a try/catch in Main.java. But now I'm getting this error:
I tried to add catch to newPrompt but that didn't work. And even when I add in the 'throws IOException' in newPrompt, I get additional errors. But thankfully, I'm getting close to taking care of this assignment.
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsHi Anthony,
I'm sorry I can't be more helpful than I have been. I'm afraid I'm just not that experienced in Java, but I'm glad you're getting further to completing the code challenge!
Anthony Renwick
3,609 PointsAnthony Renwick
3,609 PointsWell, thanks for responding Jonathan. It's good to know that I'm not alone in this. Do you by any chance know anyone else on Treehouse who may be able to help me?