Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial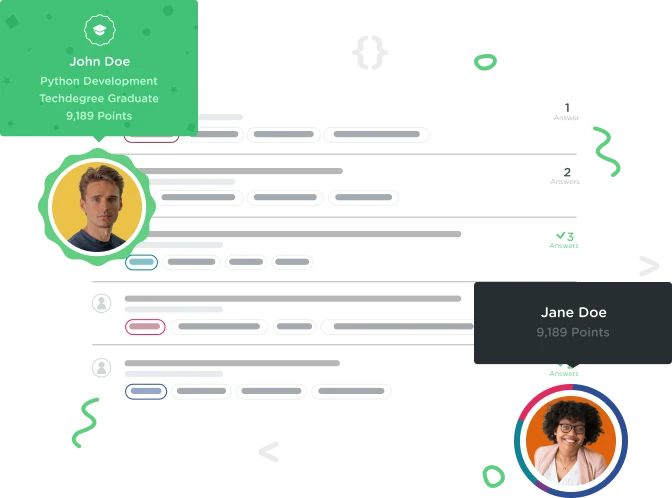
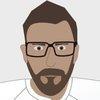
Joe Bruno
35,909 PointsjQuery Ajax Calling PHP Function
Hello,
I have a button, which functions as a shopping cart "add to cart" button. When clicked, it fires an AJAX call to the server that sends the value to the server and processes a php function.
This part is working - no problems; however, part of the php function is to add items to an array. I want to fire a second ajax call that reloads a section on the page (the shopping cart).
Below is what I am trying to accomplish:
(function ($) {
$(".addToCart").click(function(e){
e.preventDefault();
var cartData = $(this).val();
$.ajax({
type: 'POST',
dataType: 'json',
url: ajaxurl,
data: { action: 'get_cart_item', post_id: cartData },
})
.done($.ajax({
type: "POST",
url: ajaxurl,
data: { action: 'build_shopping_cart_html'},
success: alert("Cart Updated"),
}));
});
}(jQuery));
The alert executes properly, but the php function build_shopping_cart_html does not reload on the page with the added item - it doesn't seem to reload at all. Suggestions?
3 Answers

Thomas Jaede
Courses Plus Student 7,633 PointsHi Joe,
Let's assume your PHP file is returning the proper markup when you call the build_shopping_cart_html action. Once that markup is returned, you need to tell the page that you'd like to replace the old cart html with the new cart html
For example: Lets assume you have the following HTML before making the call to add_to_cart.
<ul id="cart">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
</ul>
Now you call "add to cart" with Item 4.
Correct me if I'm wrong, but your build_shopping_cart_html action is returning something like
<ul id="cart">
<li>Item 1</li>
<li>Item 2</li>
<li>Item 3</li>
<li>Item 4</li>
</ul>
If this is the case, you need to remove the Old HTML from the page, and add the new HTML. Something like....
var cartData = $(this).val();
$.ajax({
type: 'POST',
dataType: 'json',
url: ajaxurl,
data: { action: 'get_cart_item', post_id: cartData },
})
.done($.ajax({
type: "POST",
url: ajaxurl,
data: { action: 'build_shopping_cart_html'},
success: function(responseData) {
$("cart").remove();
$("cartParentElement").append(responseData);
}
}));
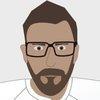
Joe Bruno
35,909 PointsThanks for the reply Thomas.
Something is still not quite right. I believe the problem is with my php function. The ajax call is successful, but only returns a "0."
My html is simply:
<div id="cart-parent">
<?php echo build_shopping_cart_html(); ?>
</div>
My PHP function is as follows:
function build_shopping_cart_html() {
$user_ID = get_current_user_id();
$userCartMeta = get_user_meta($user_ID, '_ifm_cart_items');
//var_dump($userCartMeta[0]);
echo "<ul id='cart'>";
if (empty($userCartMeta[0])) {
echo "Your Cart is empty!";
} else {
foreach($userCartMeta[0] as $activeCartItem => $cartItemStatus) {
if ($cartItemStatus == "active" || $cartItemStatus == 'dual') {
$cartItemData = get_post($activeCartItem);
$cartItemMeta = get_post_meta($activeCartItem);
$cartItemTitle = $cartItemData->post_title;
$cartItemContent = $cartItemData->post_content;
$cartItemImage = $cartItemMeta["_cmb_book_image"];
$cartItemPrice = $cartItemMeta["_cmb_book_price"];
$cartItemPublisher = $cartItemMeta["_cmb_publisher"];
$cartItemPubYear = $cartItemMeta["_cmb_year_published"];
$cartItemAuthor = $cartItemMeta["_cmb_book_author"];
$cartItemCoAuthor = $cartItemMeta["_cmb_book_coauthor"];
$cartItemPagesNum = $cartItemMeta["_cmb_total_pages"];
$cartItemISBN = $cartItemMeta["_cmb_isbn"];
$cartItemReview1 = $cartItemMeta["review_1"];
$cartItemReview2 = $cartItemMeta["review_2"];
echo "<li>" . $cartItemTitle . "</li>";
error_log($cartItemTitle);
}
}
}
echo "</ul><br>";
}
I simply am trying to get the ajax call to return the titles. The function loads initially on the original page as desired, but with the ajax jQuery function, the content is remove (correctly), but is replaced with only a "0". Any further suggestions?
Ajax function:
(function ($) {
$(".addToCart").click(function(e){
e.preventDefault();
var cartData = $(this).val();
$.ajax({
type: 'POST',
dataType: 'json',
url: ajaxurl,
data: { action: 'get_cart_item', post_id: cartData },
})
.done($.ajax({
type: "POST",
url: ajaxurl,
data: { action: 'build_shopping_cart_html'},
success: function(responseData) {
console.log("success"); // works. outputs to console success
$("#cart").remove();
$("#cart-parent").append(responseData);
}
}));
});
}(jQuery));
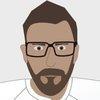
Joe Bruno
35,909 PointsGot it.
I had forgotten the add_action WordPress hook for the SECOND ajax call.
add_action( 'wp_ajax_build_shopping_cart_html', 'build_shopping_cart_html' );