Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial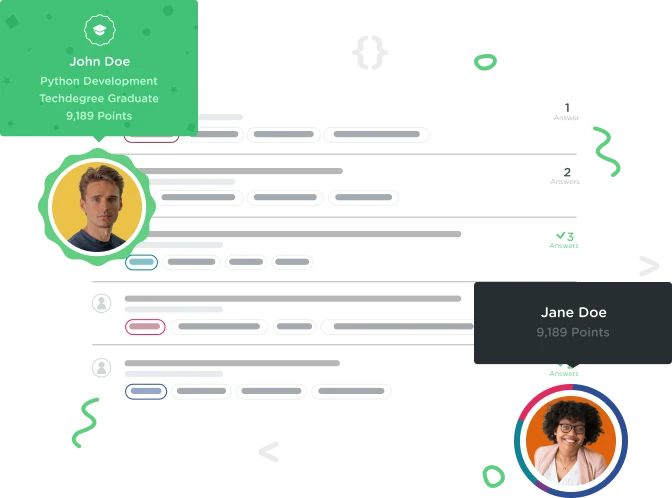
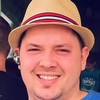
Allen Lamphear
Courses Plus Student 6,660 Points(jquery ajax) checkbox in a table - send post
I got a quick question. I have a table that generates a list of employees.
[] Has Work | John Doe | Employee Id
[] Has Work | Jane Doe | Employee Id
The table looks like that.
What I am trying to do is when you check the "Has work" it will post to a DB with the table row. ie. has work, name, employee id.
And if I uncheck it then posts again (delete that row in the db).
I just cant figure out how to get the other columns, ie. name and employee id. I know I can grab the checkbox value but I need the others.
$(function(){
$('#text').change(function(){
var date = $('#text').val();
$.ajax({
url: "testing.php",
type: 'GET',
data: 'date=' + date,
success: function(data) {
$('#driverStuff').html(data);
},
error: function(xhr, desc, err) {
console.log(xhr);
console.log("Details: " + desc + "\nError:" + error);
}
});
});
$('#haswork').change(function(){
var hw = $('#haswork').val();
$.ajax({
url: "hasWork.php",
type: 'POST',
data: 'cHW=' + hw,
success: function(data) {
$('#alert').html(data);
},
error: function(xhr, desc, err) {
console.log(xhr);
console.log("Details: " + desc + "\nError:" + error);
}
});
});
});
testing.php - http://pastebin.com/0M45BVEe
index.php - http://pastebin.com/uk49fD83
2 Answers

Geoff Parsons
11,679 PointsYou've got a couple of options. You could include data- attributes on the checkbox with the other info you need to submit. This can feel a little redundant though:
<input type="checkbox" name="hasWork" data-employee-id="1234" data-name="John Doe">
or you could do some DOM walking to find the row and then subsequently the required table cells to submit. This will be more fragile as changes to the DOM could potentially break your submissions:
$('input.hasWork').change(function(event){
var name = $(this).parents('tr').find('td.name').text();
var name = $(this).parents('tr').find('td.employeeId').text();
});
or you could even have each row be a form (double check that's valid markup) and submit on change.
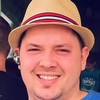
Allen Lamphear
Courses Plus Student 6,660 PointsGeoff Parsons - Thanks for the feedback. I am actually just starting out with javascript so I am working on improving and by using this site and feedback by people I am learning a lot. I did change my checkbox id's to classes and actually have them actually all different by what they are used for. ie. hasWorkAv (Available) , hasWorkNA (Not Available), etc.
Here I have a post of what it looks like so you can see a visual of what I am trying to do.
http://i.imgur.com/bVipFpg.png
I'll try out what you said about the couple of options to see how it works. Anymore feedback is much appreciated.
Geoff Parsons
11,679 PointsGeoff Parsons
11,679 PointsJust wanted to point out that it looks like you're outputting multiple elements with the same id, you should switch those over to classes:
echo '<td><input type="checkbox" name="hasWork" class="hasWork"/> Has Work</td>';
$('input.hasWork').change(...);