Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial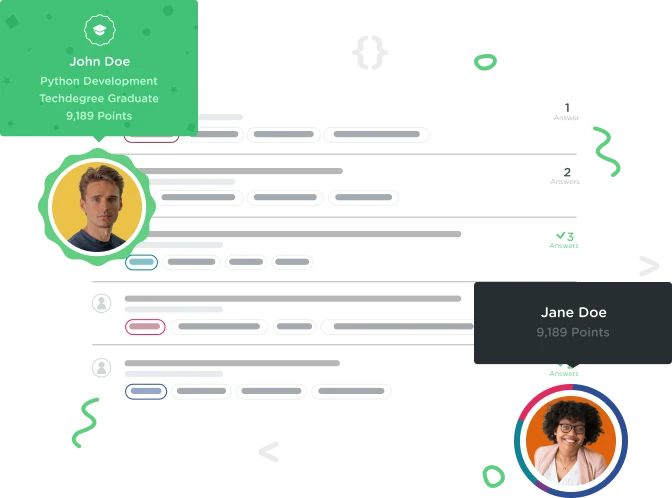

Christina Pagano
15,676 PointsJQuery Basics: Creating a Password Confirmation Form - Submit button remains disabled when passwords are valid...
var $password = $("#password");
var $confirmPassword = $("#confirm_password");
// Hide hints
$("form span").hide();
function isPasswordValid() {
return $password.val().length > 8;
}
function arePasswordsMatching() {
return $password.val() === $confirmPassword.val();
}
function canSubmit() {
return isPasswordValid() && arePasswordsMatching();
}
function passwordEvent(){
// Find out if password is valid
if(isPasswordValid()) {
//Hide hint if valid
$password.next().hide();
} else {
//Else show hint
$password.next().show();
}
}
function confirmPasswordEvent() {
//Find out if password and confirmation match
if(arePasswordsMatching()) {
//Hide hint if match
$confirmPassword.next().hide();
} else {
//Else show hint
$confirmPassword.next().show();
}
}
function enableSubmitEvent() {
$("#submit").prop("disabled", !canSubmit());
}
//When event happens on password input
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent());
//When event hapens on confirmation input
$confirmPassword.focus(confirmPasswordEvent).keyup(confirmPasswordEvent).keyup(enableSubmitEvent());
enableSubmitEvent();
So, the password hints show up and disappear just fine at the appropriate times, and when the password and confirm password are both valid the submit button should enable, but remains disabled. Can anyone see why? Don't know if I'm missing something obvious. Lol.
3 Answers

Jason Anello
Courses Plus Student 94,610 PointsHi Christina,
Didn't spot this the first time around but in your last keyup event for both inputs, you're trying to call the enableSubmitEvent
function rather than passing in just the name of the function.
keyup(enableSubmitEvent());
You need to remove the parenthesis after the function name.

Jay Laiche
8,612 PointsStill learning jQuery myself, but try removing the "not equal to" operator from the enableSubmitEvent function.
function enableSubmitEvent() {
$("#submit").prop("disabled", canSubmit());
}

Christina Pagano
15,676 PointsWell, I want it to only be disabled when I can NOT submit, so when canSubmit() is false. I did try this though and it enabled the submit button to work when the passwords were valid as well as invalid, so there's something going on with it not being able to pick up isPasswordValid and arePasswordsMatching, although the functions to show/hide the hint labels do... argggh. Thank you for taking the time to read and respond though. (:

Jason Anello
Courses Plus Student 94,610 PointsDo you have the id
set on your submit button?

Christina Pagano
15,676 PointsYes! Haha. I rewatched and followed everything Andrew did, still not working right, might just have to let this one go. Maybe a bug with my browser or workspaces. Merp.
Christina Pagano
15,676 PointsChristina Pagano
15,676 PointsAhhhhh! You're right! Thank you so much!
Jason Anello
Courses Plus Student 94,610 PointsJason Anello
Courses Plus Student 94,610 PointsYou're welcome