Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial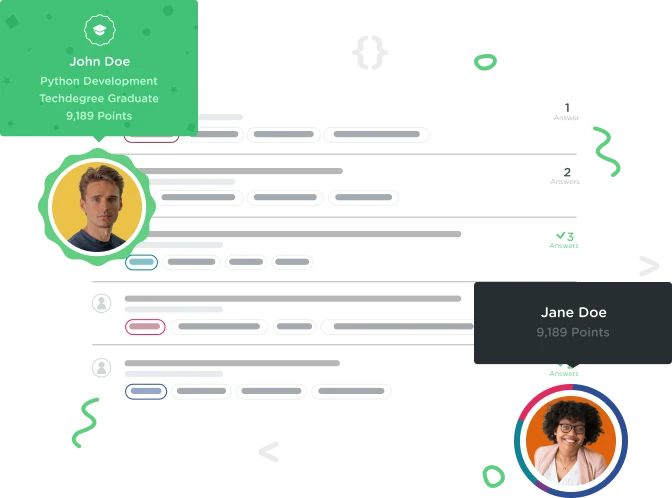
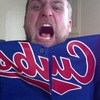
Paul Jackson
8,943 PointsJQuery buttons trouble?
I have a task manager with three task buttons and a Submit button. The Submit button's text should change to "Schedule," "Queue," or "Save" depending on which of the three task buttons you've clicked on. If you've clicked off of them or haven't yet clicked on any, the Submit button should read "Send."
The way I've approached it is creating 4 Submit buttons total and I'm trying to get them to show/hide at the appropriate times, while only showing one at a time, again, depending on if you've clicked a task button and which one.
So far, I've been able to conquer getting the three task buttons to show and hide properly, but the Send button either always stays shown or hidden, or there are more than one button shown in the task manager. I've tried if/else statements, but I seem to be getting nowhere. My code is shown below. Any ideas?
$(document).ready(function(){
var showSend = $(".submitButton, .queueButton, .scheduleButton").show();
//drag the task manager
$(".taskManager").draggable();
//hide & add buttons & extensions
$(".calendarContent, .queueContent, .saveContent, .scheduleButton, .queueButton, .saveButton").hide();
//slide the individual button extensions down
$('#calendar').click(function(){
$(".calendarContent, .scheduleButton").toggle();
$(".queueContent, .saveContent").hide()
if (showSend === true) {
$(".submitButton").hide();}
else {$(".submitButton").show();}
});
$('#queue').click(function(){
$(".queueContent, .queueButton").toggle();
$(".calendarContent, .saveContent").hide();
});
$('#save').click(function(){
$(".calendarContent, .queueContent").hide();
});
});
<body>
<form>
<div class="taskManager">
<textarea class="taskInfo"></textarea>
<div class='buttons'>
<input type="button" class="task" id="calendar">
<input type="button" class="task" id="queue">
<input type="button" class="task" id="save">
<button class="submitButton">Send</button>
<button class="saveButton">Save</button>
<button class="queueButton">Queue</button>
<button class="scheduleButton">Schedule</button>
</div>
<div class="calendarContent">
</div>
<div class="queueContent">
</div>
</div>
</form>
</body>
1 Answer
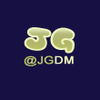
Jonathan Grieve
Treehouse Moderator 91,253 PointsIf you're using a boolean as a condition to test for the "send" button, don't you need to add a false declaration show jquery knows which text to show and task to perform? The the value doesn't change then the condition will always remain the same!.
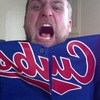
Paul Jackson
8,943 PointsI thought that's what the 'else' was doing?
If showSend is true, hide the submitButton. Otherwise, show it.
Am I incorrect?
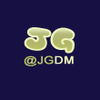
Jonathan Grieve
Treehouse Moderator 91,253 PointsThere's no true Boolean value to test.
I'd suggest that you add a showSend = true; under your first instance of showSend so your condition has a value to compare.. And then a false in your else condition!
That should theoretically allow you to break the true condition and show the right text
Theoretically :)
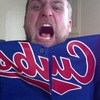
Paul Jackson
8,943 PointsI'm confused...
The way I see it is upon click, the .scheduleButton gets toggled and is therefore shown. That should make the showSend condition true and hide the .submitButton. When it's clicked again it's toggled off and therefore hidden. That should make the condition false. I moved some things around. Here's my updated code:
//slide the individual button extensions down
$('#calendar').click(function(){
$(".calendarContent, .scheduleButton").toggle();
$(".queueContent, .saveContent, .queueButton, .saveButton").hide();
var showSched = $(".scheduleButton").show();
if (showSched = true) {
$(".submitButton").hide();}
else {
$(".submitButton").show();
}
});
^ Shouldn't that make the submitButton hide and show depending on whether or not the .scheduleButton is?
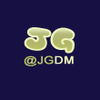
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Paul,
I'm sorry I've confused you :)
Let me show you what I mean with this code.
$(document).ready(function(){
var showSend = $(".submitButton, .queueButton, .scheduleButton").show();
**showsend=true;**
//drag the task manager
$(".taskManager").draggable();
//hide & add buttons & extensions
$(".calendarContent, .queueContent, .saveContent, .scheduleButton, .queueButton, .saveButton").hide();
//slide the individual button extensions down
$('#calendar').click(function(){
$(".calendarContent, .scheduleButton").toggle();
$(".queueContent, .saveContent").hide()
if (showSend === true) {
$(".submitButton").hide();
showSend=false;
}
else {$(".submitButton").show();
showSend=true;
}
});
$('#queue').click(function(){
$(".queueContent, .queueButton").toggle();
$(".calendarContent, .saveContent").hide();
});
$('#save').click(function(){
$(".calendarContent, .queueContent").hide();
});
});
I'm just wondering if the boolean is the way to "flag" a button state, that is let the condition know what button state to show based on the value of the showSend boolean variable.
Or maybe it's sometihing that can be solved with a condition that goes inside a while loop! Sorry I can't be more helpful than that! :-)
Paul Jackson
8,943 PointsPaul Jackson
8,943 PointsSorry for the delayed response and I do appreciate your efforts to help. I'm kinda new to this so I'm just playing around with this stuff. Still trying to figure this one out. It's a head-scratcher ha :)