Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial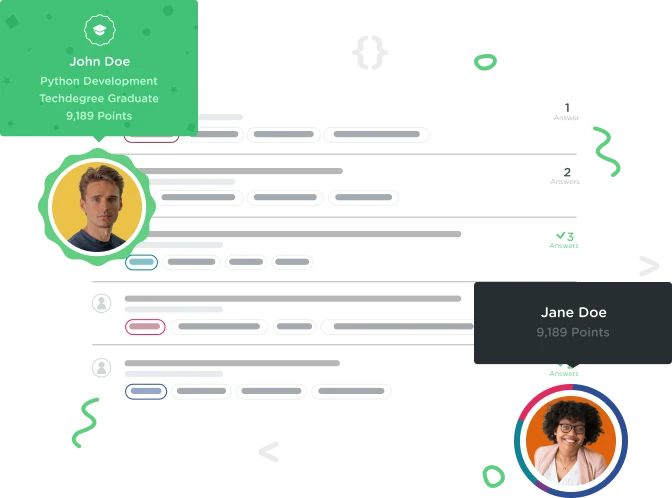
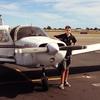
Eliot Winchell
8,341 PointsjQuery Email Validation - need help :)
Hi there! So I'm following along with the jQuery tutorial of the password confirmation box and I'm trying to do a similar thing except validate an email and check if the value in the email box is the same with the confirm email box. Right now in the javascript console I get an error in the second function that says "Uncaught SyntaxError: Unexpected token { " Also, the problem pretty much is that the email validation doesn't work and the side notes that say to enter an email and confirm it are always there and it doesn't work at all. Been changing a bunch of things but maybe it's something obvious I'm missing out on.
<!DOCTYPE html>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=utf-8"/>
<title>Winchell Associates</title>
<link rel="stylesheet" href="css/normalize.css"/>
<link href='http://fonts.googleapis.com/css?family=Open+Sans:400,700,600,300,800' rel='stylesheet' type='text/css'/>
<link rel="stylesheet" href="css/main.css"/>
<script src="https://maps.googleapis.com/maps/api/js?sensor=false"></script>
</head>
<body>
<section class="sides">
<header>
<a href="index.html" id="logo"><img src="img/logosmall.png" alt="logo"></a>
<nav>
<ul>
<li><a href="index.html">HOME</a></li>
<li><a href="about.html">ABOUT</a></li>
<li><a href="portfolio.html">PORTFOLIO</a></li>
<li><a href="services.html">SERVICES</a></li>
<li><a href="contact.html" class="selected">CONTACT</a></li>
</ul>
</nav>
</header>
<h2 class="contactheader">Contact Us!</h2>
<div id="map_canvas"></div>
<form action="#" method="post">
<h1>Contact Form
<h2>Please fill all the texts in the fields.</h2>
</h1>
<p>
<label for="name">Your Name</label>
<input id="name" name="name" type="text">
</p>
<p>
<label for="email">Your Email</label>
<input id="email" name="email" type="email">
<span>Please enter a valid Email Address</span>
</p>
<p>
<label for="confirm_email">Confirm Your Email</label>
<input id="confirm_email" name="confirm_email" type="email">
<span>Please confirm your Email Address</span>
</p>
<p>
<label for="message">Message</label>
<textarea id="message" name="message"></textarea>
</p>
<p>
<input type="submit" value="SUBMIT" id="submit">
</p>
</form>
<footer class="footerabout">
<a href="http://www.twitter.com/WinchellAssociates"><img src="img/twitter.png" alt="Twitter Logo"></a>
<a href="http://www.facebook.com/WinchellAssociates"><img src="img/facebook.png" alt="Facebook Logo"></a>
<a href="mailto:bruce@winchellassociates.com"><img src="img/mail.png" alt="Email Logo"></a>
<p>© 2014 Winchell Associates</p>
</footer>
</section>
<script src="http://code.jquery.com/jquery-1.11.0.min.js"></script>
<script src="js/app.js"></script>
</body>
</html>
var $email = $("#email");
var $confirmEmail = $("#confirm_email");
//hide hints
$("form span").hide();
function validateEmail($email){
var emailReg = new RegExp(/^(("[\w-\s]+")|([\w-]+(?:\.[\w-]+)*)|("[\w-\s]+")([\w-]+(?:\.[\w-]+)*))(@((?:[\w-]+\.)*\w[\w-]{0,66})\.([a-z]{2,6}(?:\.[a-z]{2})?)$)|(@\[?((25[0-5]\.|2[0-4][0-9]\.|1[0-9]{2}\.|[0-9]{1,2}\.))((25[0-5]|2[0-4][0-9]|1[0-9]{2}|[0-9]{1,2})\.){2}(25[0-5]|2[0-4][0-9]|1[0-9]{2}|[0-9]{1,2})\]?$)/i);
var valid = emailReg.test(email);
if(!valid) {
return false;
} else {
return true;
}
}
function confirmEmailEvent() {
//find out if password and confirmation match
if($email.val() === $confirmEmail.val() {
//hide hint if matched
$confirmEmail.next().hide();
} else {
//else show hint
$confirmEmail.next().show();
}
}
//when event happens on password input
$email.focus(emailEvent).keyup(emailEvent).focus(confirmEmailEvent).keyup(confirmEmailEvent);
//when event happenson confirmation input
$confirmEmail.focus(confirmEmailEvent).keyup(confirmEmailEvent);
1 Answer
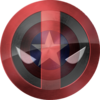
J Scott Erickson
11,883 Pointsfunction confirmEmailEvent() {
//find out if password and confirmation match
if($email.val() === $confirmEmail.val()) {
//hide hint if matched
$confirmEmail.next().hide();
} else {
//else show hint
$confirmEmail.next().show();
}
}
You were just missing the closing parenthesis. Syntax problem! Everything else looks good.
edit: the closing parenthesis in the if statement to be specific!