Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial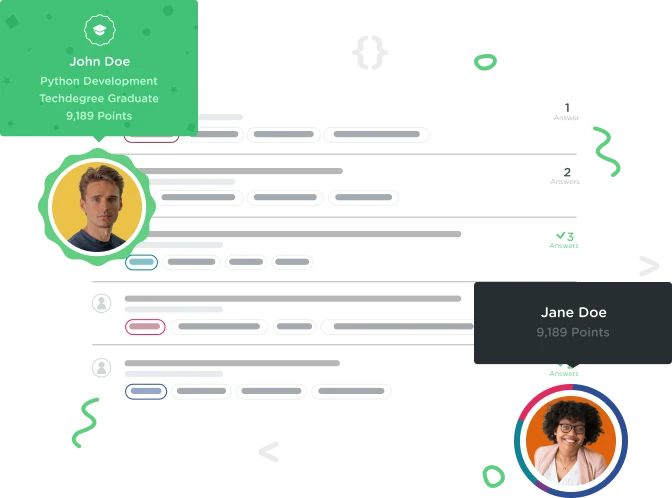
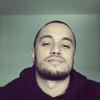
Luis Felipe Lino
Full Stack JavaScript Techdegree Student 15,707 PointsJquery function with or without parentheses
Hi folks, hope you are fine. Well my doubt is:
Why when I call a method within a conditional or within a function I must use parentheses after it, for example:
function confirmPassword(){
//hide hint if valid
if(arePasswordsMatching()){
$confirm_password.next().hide();
//else show hint
}else{
$confirm_password.next().show();
}
};
And when I call a method within a jquery event like focus or keyup I don't need to use paretheses, for example:
$password.focus(passwordEvent).keyup(passwordEvent).keyup(confirmPassword).keyup(enableSubmitForm);
Someone knows the answer?
Sorry about any english mistake ;)
3 Answers
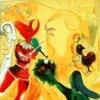
davidschreiter
15,005 PointsThe () will execute the function right then and there.
Without it you are just referring to the function not what it returns.
Sometimes you can pass a function another function for it to use later or you can pass a function the result of another function.
Some functions may be able to handle either and it doesn't matter since it might execute it right away anyways.
The if statement just wants a conditional statement (i.e. true/false) which is what arePasswordsMatching returns.
This is just my understanding of it. I could be off on a few things (or all of it). I'm positive it can be explained better than that. I'm still a bit of a Billy when it comes to programming. Cheers. Good luck.

Dean Wagman
21,591 PointsFunctions always have parentheses. Those functions you call in jQuery are functions with parentheses. They just have what we call parameters inside of them. Parameters are optional variables you can send to a function, that the function will take and use inside of itself.
For Example:
function startCar(name) {
console.log("The " + name + " is now on!");
}
startCar("Ferrari");
This code will print out "The Ferrari is now on!"
That is because our function takes a parameter, a variable we send to it, to do things; in this case print its name.
The jQuery functions are accepting parameters that they will use. In this case, we're passing the function another function to run.

Kelvin Zhao
10,418 PointsI don't think that answers the question that was asked thou. For e.g. in the question above,
$password.focus(passwordEvent);
if I were to use
$password.focus(passwordEvent());
the code wouldn't work. When do we know when to use parentheses, and when we couldn't and should just pass the function name inside?
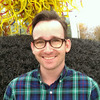
Max Pedersen
7,196 PointsDavid's answer was helpful, though the top answer on this Stack Overflow answer solidified things for me.
If you used the code $password.focus(passwordEvent()), then you are running the passwordEvent() function first (remember that JavaScript processes code from inside parentheses outward), which returns 'undefined.' Running $password.focus(undefined) is not what we're after.
Instead, we want $password.focus() to run the passwordEvent function itself--which, in this case, either hides or shows the hint, instead of returning a value. Hope that helps!
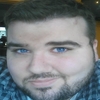
Marcus Parsons
15,719 PointsMax, the reason it is returning undefined is not because the function is being called. It will return undefined regardless of whether it is called immediately or in the future, because there is no return value listed in the function. Anytime a return value isn't specified in the function, it always returns undefined.
To prove that, run these two functions in your console separately and see the return values for each one:
function sayHi(name) {
alert("Hey there " + name + "!");
}
sayHi("Max");
function sayHiReturn(name) {
alert("Hey there " + name + "!");
return "awesome";
}
sayHiReturn("Max");
The first one will return undefined, but the second one will return "awesome" or whatever value you specify to return.
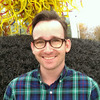
Max Pedersen
7,196 PointsHi Marcus,
Thanks for your reply. I agree with everything you've said--I think I was just a bit vague in my original comment. I should have said that in this specific lesson scenario, passwordEvent() will return 'undefined', because, as you said, that specific function in this lesson does not return anything--not that all functions will always return 'undefined'.
Though if that is also incorrect, I'd love to know, as I'm pretty new to this :).
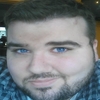
Marcus Parsons
15,719 PointsAny function that does not have a defined return statement will return undefined because return is undefined for that function. This is true for any function. The best way to see this is to construct some of your own functions that either return something or have no return statement like I just did and then copy them into the web console of Chrome or Firefox and see the return value. The return value always comes after everything executes in the code you run. For example, when running the function I posted "sayHiReturn", it will show the return value after the code.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsMarked as best answer
Robert Mews
11,540 PointsRobert Mews
11,540 PointsHey David,
By saying "The () will execute the function right there" are you saying the "()" at the end of the function name executes the function without having to return?
return functionName();
davidschreiter
15,005 Pointsdavidschreiter
15,005 PointsHi Robert,
I'm a little confused by your question. I think you may have the return statement mixed up a bit.
The return statement should be inside the function itself. Not all functions have to return something, but any return statement would have to be inside a function. In your example the code would have to exist inside another function. i.e.
If that's what your referring too, then anotherFunction would return what ever functionName would return.
If functionName didn't have the "()" at the end of it, anotherFunction would just return functionName itself and not execute the code inside functionName.
Then if you stored that result in another variable you could execute the code by using "()" after that variable's name. i.e.
This is the same as saying yetAnotherFunction equals functionName. Now at this point or any point later we can execute whatever code exists inside functionName by writing "yetAnotherFunction()" or "functionName()".
I hope i helped clear some of it up and not make it even more confusing. Good luck.