Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial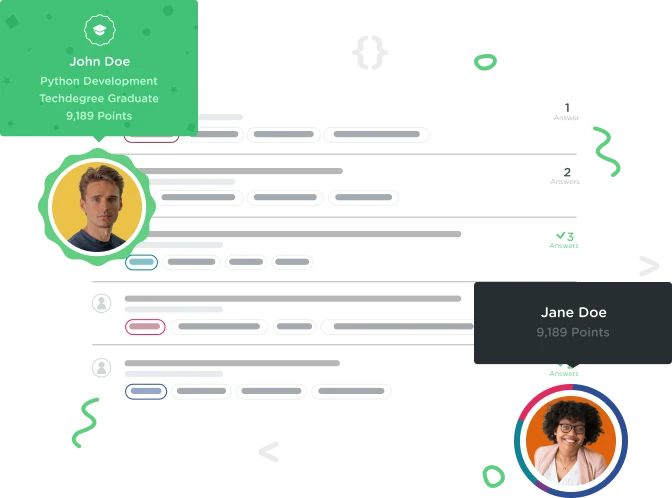
Maurits Pallesen
9,770 PointsJquery: If(function=true && function=true && function=true) {}
Hello everyone, i am working on making my own signup confirmation webpage, where i want to use jquery to make make the confirmations. The problem i'm stuck on now, is making the "submit" button disabled when the form is filled out incorrectly and then enable it when the form is filled correctly. I made it work once, but i don't think it was the optimal way of doing it. Here os my html:
<form>
<p>
<label for="username">Username</label>
<input type="text" id="username" name="username" maxlength="16" pattern=".{10,}" required title="Please use 8 letters and 2 numbers!">
<span id="username-hint">Enter a username with at least 8 letters and 2 numbers</span>
<p id="checkmark" class="checkmark"></p>
</p>
<p>
<label for="password">Password</label>
<input type="password" id="password" name="password" maxlength="16" pattern=".{8,}" required title="Your password is not 8 characters long!">
<span id="password-hint">Enter a password between 8 and 16 characters.</span>
<p id="checkmark1" class="checkmark"></p>
</p>
<p>
<label for="confirm_password">Confirm password</label>
<input type="password" id="confirm_password" name="confirm_password" maxlength="16" pattern=".{8,}" required title="Please confirm your password correctly!">
<span id="confirm-password-hint">Confirm your password please</span>
<p id="checkmark2" class="checkmark"></p>
</p>
<input type="submit" value="Submit" id="submit" disabled="disabled">
</form>
Here is my succesful attempt:
function validateSubmitButton () {
if ($username.val().length > 9 && $password.val().length > 7 && $confirmPassword.val().length > 7 && $password.val() === $confirmPassword.val()) {
$('#submit').removeAttr('disabled');
}
}
What i would really like to do, is in let the "if" statement check wether or not all the 3 validation functions (username, password and confirm password) i've written are true, and if they are, execute the function and remove the attribute. Something like this:
function validateSubmitButton () {
if (isUsernameValid=true && validatePassword=true && validatePasswordMatch=true) {
$('#submit').removeAttr('disabled');
}
}
In advance, thank you for your time and (hopefully ;) ) good answers :)
P.S. sry for the code links' sidescrolling, this was the first time i linked code.
1 Answer
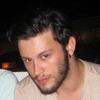
Lorenzo Pieri
19,772 PointsWell, you are actually just adding code into working code. Checking your function validateSubmitButton() I can tell you for sure that you are just implementing it the wrong way.
Using the last if statement you are checking if the values are validated the way you want them validated, which is correct because if the validation works fine, the value returned will be TRUE, as you ask.
The problem comes with the value return method. To return a TRUE in this case, you just have to stick with the first way you used (the one that worked) and than pass the returned values (true) to the last function validateSubmitButton. For me that's just a waste of space, but it's good to waste some space because that's the way things are meant to be done : clean.
function checkSubmit () {
if ($username.val().length > 9 &&
$password.val().length > 7 &&
$confirmPassword.val().length > 7 &&
$password.val() === $confirmPassword.val()) {
return true; //exiting the function
}
function validateSubmitButton (chSubmit) {
if(chSubmit === true){
$('#submit').removeAttr('disabled');
}
}
validateSubmitButton(checkSubmit()); //Passing a function as a parameter.
Long? Yeah. But the code is re-usable, it follows good practice and its more understandable!
Good day friend!