Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial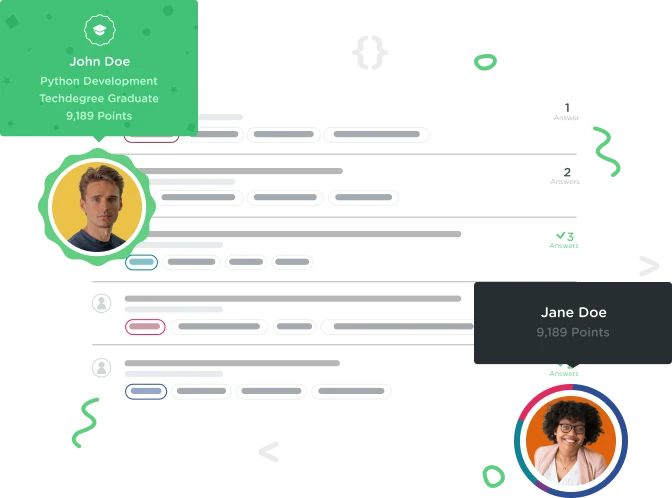

chazel
Courses Plus Student 6,961 PointsjQuery - Select current element and next element together
Let's say I have the following code structure:
<ul class="tab-nav">...</ul>
<div class="tab-content">...</div>
And I am using the modular app approach that checks for the existence of each .tab-nav on a page, making the initiating element:
$el = $('.tab-nav');
Starting with these variables below, I am able to get the correct elements.
$tabNav = $el;
$tabContent = $el.next('.tab-content');
However, if I need to wrap both elements in a div, for example, I cannot even simply achieve selecting both as a group. Here are some tries at creating a new selection based on the two above:
$tabGroup = $($tabNav, $tabContent); // returns only $tabNav
$tabGroup = $tabNav.next('.tab-content').andSelf(); // returns only $tabNav
$tabGroup = $tabNav.andSelf().next('.tab-content'); // returns only .tab-content
$tabGroup = $tabNav.next('.tab-content').addBack(); // returns only $tabNav
$tabGroup = $tabNav.addBack().next('.tab-content'); // returns only .tab-content
$tabGroup = $.extend({},$tabNav,$tabContent); // returns only $tabContent
$tabNav.before('<div class="tab-group">');
$tabContent.after('</div>');
// this works in code inspection, but it cannot be selected because--I suspect--it's not part of the DOM.
Being limited to starting from the .tab-nav element in the DOM, how can I create a new element that includes the pre-existing one ($el) along with the following node of .tab-content so that I can use the .wrap() method?
I also do not want to use any clone methods because those tend to break events and other functionality that is initiated within the content of each of those elements.
Thanks for the help in advance!
Note: I am restricted to jQuery 1.11.2 due to a CMS limitation. :(
1 Answer
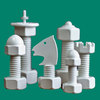
Steven Parker
231,269 PointsI'm not aware of a form of the jQuery function that will take more than one element argument, but there is one that will take an element array, so you could do this:
$tabGroup = $([$tabNav[0], $tabContent[0]]);