Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial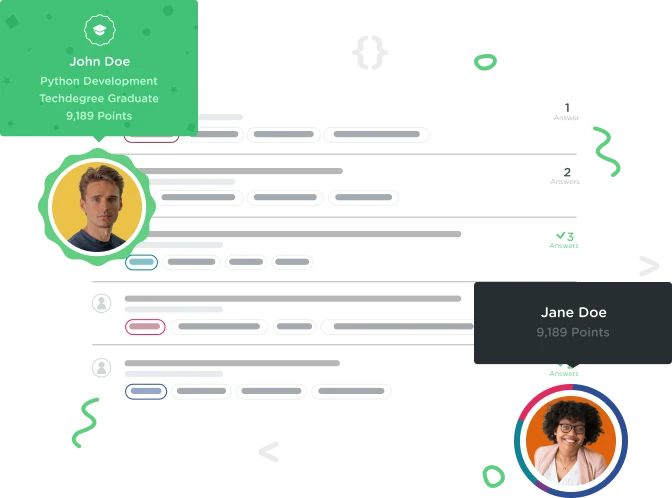

Chapman Cheng
PHP Development Techdegree Graduate 46,936 PointsjQuery selector cannot select both element and id?
In this practice, I encountered a problem in question 6, like this: HTML:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<link rel="stylesheet" type="text/css" href="styles.css">
<link rel="stylesheet" type="text/css" href="styles.css">
<title>Practice Basic jQuery Methods</title>
</head>
<body>
<h1><span>Yap!</span> World-Famous Restaurant Reviews</h1>
<div class="section">
<h2>Recent Reviews</h2>
<div class="review">
<h3>Dat Dog</h3>
<p>Best gator sausage New Orleans has to offer!</p>
</div>
<div id="newRestaurant">
<h3></h3>
<p></p>
</div>
</div>
<div class="section">
<h2>Add a Review</h2>
<p id="flashMessage">Write a review in the next 24 hours and receive a 10% off coupon!</p>
<label>Restaurant Title: <input type="text" id="restaurantNameInput"></label>
<label>Restaurant Review: <textarea id="restaurantReviewInput"></textarea></label>
<button id="submitBtn">Submit</button> <!--I use jQuery to select this element-->
</div>
<script src="js/jquery-3.2.1.min.js"></script>
<script src="js/app.js"></script>
</body>
</html>
jQuery:
$('button').click(function(){console.log("button element ok")}); //test 1
$('#submitBtn').click(function(){console.log("button id ok")}); //test2
$('button #submitBtn').click(function(){console.log("button element and id ok")}); //test3
only test 3 cannot pass the click function onto console? so why can I not specifically select the element and id at the same time?
4 Answers

andren
28,558 PointsYou seem to have misunderstood my comment slightly, I did not post a descendant selector, I was explaining that the code you originally wrote resulted in a descendant selector. The code I embedded (with commas) will select both button
elements and elements with a submitBtn
id. Which is what I though you wanted.
If you wanted a selector that checks for both element type and id simultaneously however then you simply combine the selectors without spaces or commas. Like this:
$('button#submitBtn').click(function(){console.log("button element and id ok")});
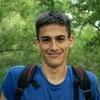
Anastasios Poursaitedes
10,491 PointsHey Chapman, if I'm not mistaken , you're asking if you can select one element which contains an id. In your case your button doesn't contain an element with an id. Your button is the id, Meaning that the button can identify itself with the button tag name but also with the id. Because you gave the button an id this means that you don't have to select it like this 'button#submitBtn' although you can but it's itself unique(becuase of the id) enough to call it just with the id. It's good to keep the specificity low.

Chapman Cheng
PHP Development Techdegree Graduate 46,936 PointsHey Anastasios, thanks for reply. You are totally right, what I asked previously, is how the jQuery selector works. Back then, I didn't understand the difference of
$(button#submitBtn)
selecting the Button element with id #submitBtn
and
$(button #submitBtn)
selecting an element id #submitBtn, which is nested in a button element
of course you are totally correct that id is exclusive and not required for another element or class in the selector, it just that I had that question before, and testing it out here.

andren
28,558 PointsjQuery uses CSS selectors to select elements, to target multiple selectors in CSS you have to separate them with a comma. Like so:
$('button, #submitBtn').click(function(){console.log("button element and id ok")});
If you separate them with a space then you end up with a descendant selector instead. Meaning that you tell jQuery to select elements with the id submitBtn
that are descendants of button
elements.

Chapman Cheng
PHP Development Techdegree Graduate 46,936 PointsThanks for replying, but I'm not looking for descendant selector, I was trying to select "one" element, which is a <button> and contains the id#submitBtn,
<button id="submitBtn">Submit</button>
so does that mean I could only pick one DOM element out of an element?

Chapman Cheng
PHP Development Techdegree Graduate 46,936 PointsThank you andren, I didn't know the space in selector makes the difference (I wish I can 'Best answer' your post)

andren
28,558 PointsI've switched my comment to an answer, so you can now select it as "Best answer" if you wish. And yeah CSS selectors are pretty peculiar about how you type them, since most symbols (including spaces) have a specific meaning.