Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial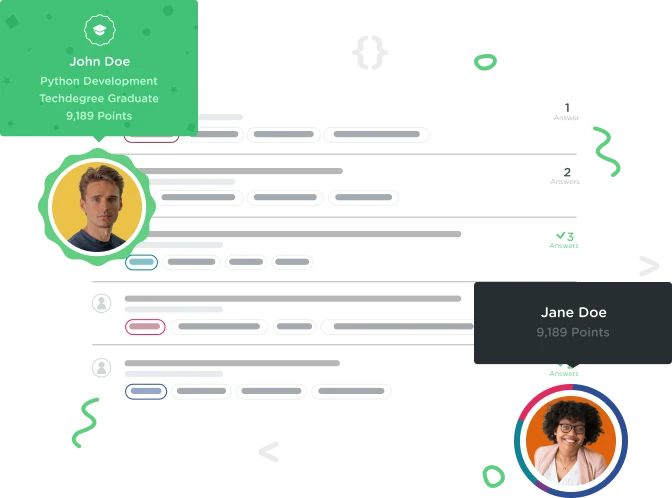
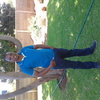
MUZ140063 Glen T. Moyo
5,257 PointsjQuery's fail() method takes a callback function as an argument. The function is run when there's an error in the AJAX r
jQuery's fail() method takes a callback function as an argument. The function is run when there's an error in the AJAX response. Pass an anonymous function as an argument to the method. Don't forget to set a parameter for that function. Use the name jqXHR for the parameter -- this parameter will hold a jQuery XHR object passed by the fail() method to the function
$.get("missing.html", function(data) {
$("#footer").html(data);
}).fail(function (jqXHR);
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<title>AJAX with JavaScript</title>
</head>
<body>
<div id="main">
<h1>AJAX with jQuery</h1>
</div>
<div id="footer"></div>
<script src="jquery.js"></script>
<script src="app.js"></script>
</body>
</html>
4 Answers
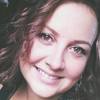
Chelsea Van Dyke-Spitzer
6,939 PointsI kept getting an error, realized that I needed an extra closing parenthesis for it to work. Like this:
.fail(function(jqXHR) {
});
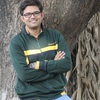
Prashant chaudhari
Courses Plus Student 24,499 Pointscorrect code here.............. $.get("missing.html", function(data) { $("#footer").html(data); }).fail(function (jqXHR){});
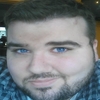
Marcus Parsons
15,719 PointsHey MUZ140063 moyo,
What's happening here is that we need an anonymous callback function to pass into the fail method with a parameter of jqXHR. If you remember, an anonymous function is just a function that has no name attached to it. When they say a parameter of "jqXHR", they mean that that is its argument.
An anonymous callback function often looks like this with an argument of jqXHR:
function (jqXHR) {
//code here
}
I hope that helps! :)
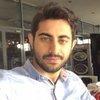
HIDAYATULLAH ARGHANDABI
21,058 Pointssince we pass the call back back function to the fail method. the call back function will be trigged when it fail. and it takes an argument called jqXHR
$.get("missing.html", function(data) {
$("#footer").html(data);
}).fail(function(jqXHR){});