Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial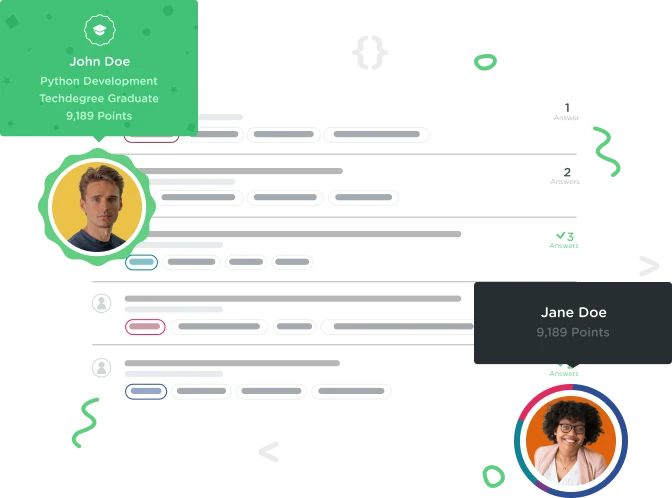
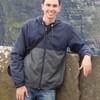
Trevor Johnson
14,427 PointsJS Find the Longest Word in a String
Hi, so I have this challenge on FCC, and I am looking for some help in figuring this out, not a direct answer unless I ask. If someone could give me some advice on my code, and help me understand what I should do I would appreciate it.
Directions: Return the length of the longest word in the provided sentence. Your response should be a number.
function findLongestWord(str) {
var length;
var stringArray = [];
var string = str;
stringArray = string.split(' ');
stringArray.sort();
for (i = 1; i < stringArray.length; i++){
length = stringArray[i].length;
}
return length;
// return stringArray;
}
findLongestWord("The quick brown fox jumped over the lazy dog");

Jason Anello
Courses Plus Student 94,610 PointsHi Trevor,
Were you intending to sort the words by length? As you have it now, the default sort isn't going to help you and will only waste time because it sorts based on unicode point order. You would need to use a compare function to sort by length.
If you did do that then you wouldn't need to loop at all because the longest word would be at the end or the beginning depending on the sort order.
However, I recommend that you take the approach hinted at in the answers and not do any sorting because those approaches will be faster than sorting.
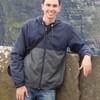
Trevor Johnson
14,427 PointsMy loop seems to be checking the length of the first word in the array instead of looping through the whole thing and only adding to the length variable if the array item is longer than the variable. Am I returning too early?
function findLongestWord(str) {
var length;
var stringArray = [];
var string = str;
stringArray = string.split(' ');
for (i = 0; i < stringArray.length; i++) {
length = 0;
if (stringArray[i].length > length) {
length += stringArray[i].length;
}
return length;
}
}
findLongestWord("The quick brown fox jumped over the lazy dog");

andren
28,558 PointsTrevor Johnson: Yes, you are returning to early. Your return statement is placed within your for loop, which means that the function will always return after one loop.
That issues can be solved by simply moving it out of the loop. But it is far from the only issue in your current code. Your length variable should not be declared with it's initial value within the loop, but outside it. Declaring it within the loop means that it will be set to that value each time the loop runs, which means that it won't be useful for keeping track of the previous item's length.
There is another issue in your code but since you want to work on this yourself I'll limit my hints for the time being.
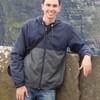
Trevor Johnson
14,427 PointsThanks for your help guys, I solved it. I didn't know that placing that variable within the loop would reset it to zero each time.
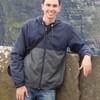
Trevor Johnson
14,427 PointsDo any of you happen to know a more efficient way for doing this challenge than I did it? Something that would use less code?

Jason Anello
Courses Plus Student 94,610 PointsEdit: renamed variable using camelcase.
In terms of efficiency, I don't know of anything. This solution is going to run in linear time. You have to go through the array and look at every single word.
If you had sorted it then it would probably run on average in n log(n) time.
There's a graph on the following big O wikipedia page https://en.wikipedia.org/wiki/Big_O_notation
If you look at the graph with the different time complexities, you'll see that n log(n)
grows much faster than n
In terms of shorter code, you could handle the variable declarations part a little differently.
I would probably write it like this:
var maxLength = 0; // you can declare and initialize the max length in one step. I also renamed it to better indicate what it's storing.
var words = str.split(' '); // same thing here. the words can be declared and initialized in one step.
// then do the loop

andren
28,558 PointsTrevor Johnson: More efficient and less code is not always the same thing, your solution is actually pretty efficient performance wise. But as far as code length is concerned the shortest way I can think of to code this function is this:
function findLongestWord(str) {
return str.split(' ').sort((a, b) => b.length - a.length)[0].length;
}
The more you try to shorten code the less readable it tends to become, especially to a beginner since it often involves using somewhat advanced functions and features. But in essence I'm just combining various operations into one line.
I first split the string then I call the sort
method on that split string, then pass a function (arrow function) to the sort
method which tells it how I want it to sort the array. The condition I provide will result in the array being sorted from longest word to shortest. Then I pull out the first item from the array and call the length
method on that.
2 Answers

andren
28,558 PointsWith a task like this it's hard to give any significant advice without pretty much giving the solution away. But one thing I will say is that your loop is setup incorrectly. i
should be set equal to 0 at the beginning of the loop not 1. Indexes are 0 based so starting it at 1 will lead to you skipping the first word.
Other than that I'll say that for a task like this you don't really need a variable to store the length of the current item, but one for storing the length of the longest item. Which should be set only if the item has a length greater than the length of one of the previous items. So a conditional statement will be required. That's the only hints I think I can give without making the solution too obvious.
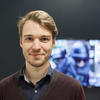
Lean Rasmussen
11,060 PointsThere is a 2 or 3 liners for doing this. but for your way of thinking about this. You could say that your variable length holds the length of the longest word. let it be 0 to start with. then you loop though you can have a conditional saying, if the length of the current word is longer that length store it in a variable, and update the varriable

Jason Anello
Courses Plus Student 94,610 Pointschanged comment to answer
andren
28,558 Pointsandren
28,558 PointsLean Rasmussen The poster explicitly states that they don't want a direct answer (solution) only a hint as to what to do. Your answer definitively provides more than that.
Also the challenge is to return the length of the longest word, not the longest word itself.
Edit: Thank you for editing your answer.