Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial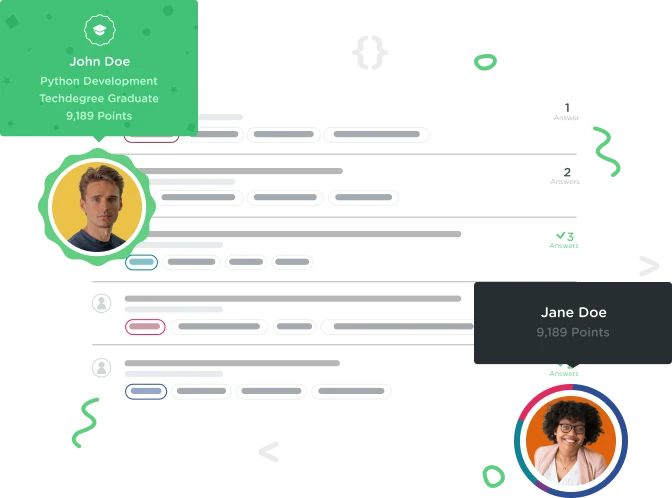

James Wright
Front End Web Development Techdegree Student 10,106 PointsJS For loop stops iterating
In my example I am trying to loop through a collection of HTML elements and check to see if they match a button click on screen. But the loop only seems to check the first html element and does not run the function again if another button is clicked.
keyboard.onclick = function(event) {
event.target.className += " chosen";
let letterFound = event.target.innerHTML;
checkLetter(letterFound);
}
const checkLetter = (a) => {
let letterCheck = document.querySelectorAll('.letter');
for (i = 0; i < letterCheck.length; i++) {
if (a === letterCheck[i].innerHTML) {
letterCheck[i].className += " show";
let letterMatch = letterCheck[i];
return letterMatch
} else {
return null;
}
}
}
2 Answers
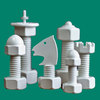
Steven Parker
230,274 PointsI still needed to guess at the HTML, but to keep looking, do not return on a match but just establish the result value.
//Check Letter
const checkLetter = a => {
let letterCheck = document.querySelectorAll(".letter");
let result = null; // preset the result to null
for (i = 0; i < letterCheck.length; i++) {
if (a === letterCheck[i].innerHTML) {
letterCheck[i].classList.add("show"); // Note: better way to add a class
result = a; // found at least one, set result to letter
//return letterMatch; // do NOT return here, keep looking
}
}
return result; // finally, return the result
};
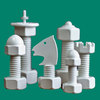
Steven Parker
230,274 PointsThere are no obvious issues in the code shown here, but there are plenty of opportunities for problems in the code that is not shown. For example:
- the assignment of "keyboard" is not shown, it's not clear what it represents
- none of the HTML is shown, so the interaction with the script is not clear
- the CSS is not shown, so the effect of adding a class is unknown
- the purpose for the return value from "checkLetter" is unclear, it is not used by the event handler

James Wright
Front End Web Development Techdegree Student 10,106 PointsHI Steven,
Thanks for your input.
I've added the full JS below. Made some changes since earlier but similar issues.
When a user clicks a button on screen (child element of keyboard), the letter is looped over a node list in the checkLetter function.
It mostly works fine, but the loop stops when it finds a match. I assume this is to do with my return letterMatch. However there are duplicate letters in the list. How would I loop the whole list and return the matched value?
Thanks :)
const keyboard = document.getElementById('qwerty');
const phrase = document.getElementById('phrase');
let missed = 0;
const phrases = ['Iron Man', 'The Hulk', 'Black Widdow', 'Dr Strange', 'Thanos'];
const getRandomPhrase = (a) => {
let randomPhrase = a[Math.floor(Math.random() * phrases.length)];
let randomPhraseLower = randomPhrase.toLowerCase();
return randomPhraseLower.split("");
};
keyboard.onclick = function(event) {
event.target.className += " chosen";
let letterFound = event.target.innerHTML;
checkLetter(letterFound);
console.log(checkLetter(letterFound));
if (checkLetter(letterFound) === null) {
missed++;
}
checkWin();
}
const checkWin = () => {
let letterCheck = document.querySelectorAll('.letter').length;
let letterCorrect = document.querySelectorAll('.show').length;
if (letterCheck == letterCorrect) {
console.log("You Win!");
} else if (missed >= 5) {
console.log("you Lose");
}
}
const addPhraseToDisplay = (a) => {
var i = 0;
const letters = a;
const letter = letters[i];
const ul = document.querySelector('ul');
for (i = 0; i < letters.length; i++) {
const li = document.createElement('li');
const liLetter = li.appendChild(document.createTextNode(letters[i]));
if (li.textContent === " ") {
li.className += "";
} else {
li.className += "letter";
}
ul.appendChild(li);
}
}
//Check Letter
const checkLetter = (a) => {
let letterCheck = document.querySelectorAll('.letter');
let i = 0
for (i = 0; i < letterCheck.length; i++) {
if (a === letterCheck[i].innerHTML) {
letterCheck[i].className += " show";
let letterMatch = letterCheck[i];
return letterMatch;
}
}
return null;
}
const phraseArray = getRandomPhrase(phrases);
addPhraseToDisplay(phraseArray);
console.log(phraseArray);```
James Wright
Front End Web Development Techdegree Student 10,106 PointsJames Wright
Front End Web Development Techdegree Student 10,106 PointsThank you so much Steven! That did the trick :)