Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial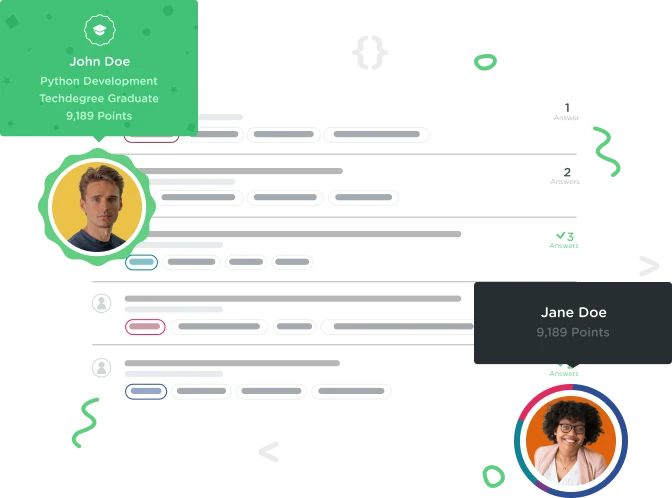
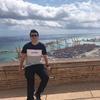
Tadjiev Codes
9,626 PointsJS function average problem
let outputElement = document.getElementById("output");
let numberArrays = [10, 58, 47, 12, 5, 4, 8, -7, 521, -99];
let totalNumber = 10 + 58 + 47 + 12 + 5 + 4 + 8 + -7 + 521 + -99;
outputElement.innerHTML += numberArrays.length;
function findAverage() {
var average = 0;
var sum = 0;
for (var counter = 0; counter < numberArrays.length; counter++) {
sum += numberArrays[counter];
}
average = totalNumber / numberArrays.length;
return average;
}
let averageNum = findAverage();
outputElement.innerHTML += (averageNum);
My result becomes 1055.9 when it's supposed to be only 55.9? Wheres the extra 10 coming from?
3 Answers
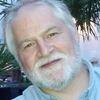
Jeff Muday
Treehouse Moderator 28,717 PointsJavaScript is a great language, but it's automated type conversions can make for some unexpected results.
In this case you are adding a string (from outputElement.innerHTML) and a number (averageNum). JavaScript is pretty tolerant, so string conversion takes precedence and averageNum is converted to a string. so the essentially you concatenate two strings rather than doing a numeric computation. "10" + "55.9" = "1055.9".
If we use the function Number() we can ensure that the string returned from outputElement.innerHTML is used as a number rather than a string. We also should not use "=+" operation so we can do the expected conversion.
I would also note that a better programming practice is to minimize the use of any HTML elements as computing structures-- but the thing I love about programming is there are MANY correct ways to solve a problem.
Good luck with your JavaScript journey! It is a valuable skill to study!
let outputElement = document.getElementById("output");
let numberArrays = [10, 58, 47, 12, 5, 4, 8, -7, 521, -99];
let totalNumber = 10 + 58 + 47 + 12 + 5 + 4 + 8 + -7 + 521 + -99;
// this will ensure you have a numeric computation rather than string concat
outputElement.innerHTML = Number(outputElement.innerHTML) + numberArrays.length;
function findAverage() {
var average = 0;
var sum = 0;
for (var counter = 0; counter < numberArrays.length; counter++) {
sum += numberArrays[counter];
}
average = totalNumber / numberArrays.length;
return average;
}
let averageNum = findAverage();
// this will ensure you have a numeric computation rather than string concat
outputElement.innerHTML = Number(outputElement.innerHTML) + (averageNum);

Paul Messmer
15,023 PointsThe extra 10 I think is coming from line 6 when you add 10 to the outputElement.innerHTML. 10 is the length of the string, then at the bottom you are getting the correct avg but you already have 10 in the variable. I am not to the point of learning about the outputElement.innerHTML but just from how variables act I think this is your problem. I tested this by changing line 6 and line 20 to console.log and I got back the 10 on line 6 then the correct avg on line 20. I hope this helped!
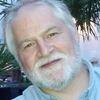
Jeff Muday
Treehouse Moderator 28,717 PointsThe code does not return a NaN in my testing. Are you using the code with a <script> tag in the context of a web page? JavaScript needs to be able to find the element with id="output" and must not contain any strings that aren't numeric.
IMPORTANT, that output area should be blank. If it is not, then you will get NaN if it attempts to convert something that is either non-blank or a string that is not convertible to a number!
See below.
<!DOCTYPE html>
<head>
</head>
<body>
<p>See output below:</p>
<p>
<div id="output">
</div>
</p>
<script>
let outputElement = document.getElementById("output");
let numberArrays = [10, 58, 47, 12, 5, 4, 8, -7, 521, -99];
let totalNumber = 10 + 58 + 47 + 12 + 5 + 4 + 8 + -7 + 521 + -99;
outputElement.innerHTML = Number(outputElement.innerHTML) + numberArrays.length;
function findAverage() {
var average = 0;
var sum = 0;
for (var counter = 0; counter < numberArrays.length; counter++) {
sum += numberArrays[counter];
}
average = totalNumber / numberArrays.length;
return average;
}
let averageNum = findAverage();
outputElement.innerHTML = Number(outputElement.innerHTML) + (averageNum);
</script>
</body>
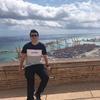
Tadjiev Codes
9,626 PointsThank you) YEs You are right. I was using one div to output multiple things from the arrays I created and once I created a new empty div it gave me the right number.
outputElement.innerHTML = Number(outputElement.innerHTML) + numberArrays.length;
This code was still adding 10 but then the lower final one did work like supposed to be 55.9 average number. Many thanks
outputElement.innerHTML = Number(outputElement.innerHTML) + (averageNum);
Could you please take a look at this question of mine where I'm having a problem as well? https://teamtreehouse.com/community/js-form-validation-day-month-year-onchange-event-according-to-the-month
Thanks in advance)))))))
Tadjiev Codes
9,626 PointsTadjiev Codes
9,626 PointsHi, Thank you for the explanation but it's returning NAN? Is there still sth wrong with the code? And Number is a reserved keyword so there's a calculation?