Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial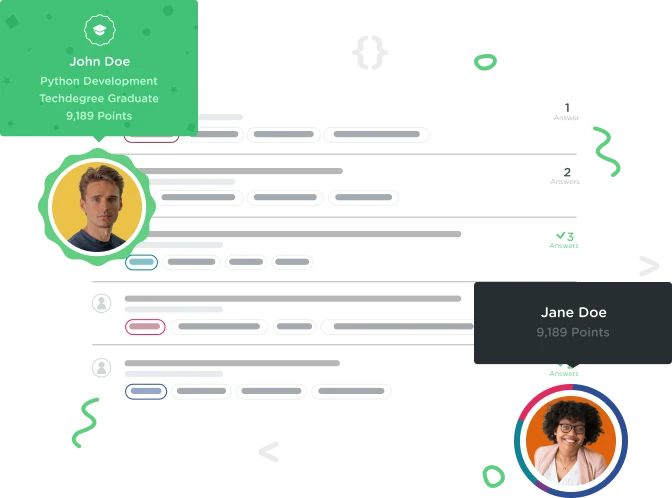
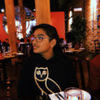
Haardik Gupta
Full Stack JavaScript Techdegree Student 5,459 Pointsjs help
In this challenge you'll write a validator for a hexadecimal string used to set a CSS color. Hexadecimal values for css colors start with a pound sign, or hash (#), then six characters that can either be a numeral or a letter between A and F, inclusive. Two examples of these strings would be #FF4569 and #578E9A.
For this step of the challenge, write a regular expression that will match any hexadecimal string and store it in a variable named hexRegEx. The regex should be case-insensitive. Assume that the string will always be 7 characters.
// Type inside this function
function isValidHex(text) {
}
const hex = document.getElementById("hex");
const body = document.getElementsByTagName("body")[0];
hex.addEventListener("input", e => {
const text = e.target.value;
const valid = isValidHex(text);
if (valid) {
body.style.backgroundColor = "rgb(176, 208, 168)";
} else {
body.style.backgroundColor = "rgb(189, 86, 86)";
}
});
<!DOCTYPE html>
<html>
<head>
<title>DOM Manipulation</title>
</head>
<link rel="stylesheet" href="style.css" />
<body>
<div id="content">
<p>Enter a valid hex value below to make the screen turn green.</p>
<input type="text" id="hex">
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
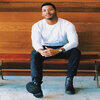
Elias Starks
Full Stack JavaScript Techdegree Graduate 13,605 PointsHey should Look something like this /^#[a-fA-F0-9]{6}$/;
Zimri Leijen
11,835 PointsZimri Leijen
11,835 PointsWhat have you tried so far?
This seems to be the challenge itself, what exactly are you getting stuck on?