Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial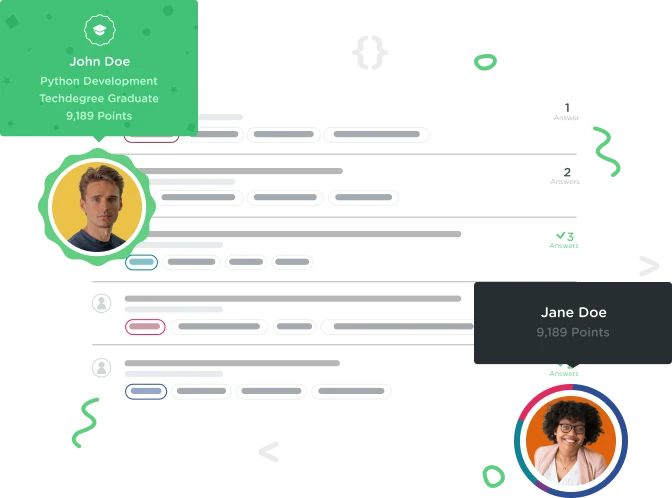
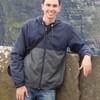
Trevor Johnson
14,427 PointsJS Help With Queues and Arrays
Hey so I am doing this code challenge on Free Code Camp, and I don't understand what this question is asking. I don't feel like its explained well so if someone can help me that would be great.
Directions:
In Computer Science a queue is an abstract Data Structure where items are kept in order. New items can be added at the back of the queue and old items are taken off from the front of the queue.
Write a function nextInLine which takes an array (arr) and a number (item) as arguments. Add the number to the end of the array, then remove the first element of array. The nextInLine function should then return the element that was removed.
function nextInLine(arr, item) {
// Your code here
return item; // Change this line
}
// Test Setup
var testArr = [1,2,3,4,5];
// Display Code
console.log("Before: " + JSON.stringify(testArr));
console.log(nextInLine(testArr, 6)); // Modify this line to test
console.log("After: " + JSON.stringify(testArr));
2 Answers

andren
28,558 PointsBroken down it is asking you to:
Add the
item
parameter to the array (arr
) that is passed in.Remove the first item of the array
Return the item that you removed.
If you want further advice just ask, but that is about as much of a break down I can give you beyond just posting the actual answer.
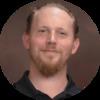
Michael Davis
Courses Plus Student 12,508 PointsIf you're going through FCC (as am I, for the challenges), then I'm going to assume you enjoy figuring out the answers. With that in mind, I offer these two references:
I'm sure you'll get it! If you need more guidance, feel free to reply and we'll definitely help out!
Trevor Johnson
14,427 PointsTrevor Johnson
14,427 PointsWhen it comes to removing the first item of an array I think of the .shift() method and the .push() method to add to the end. Other than that I don't know what to do because that isn't discussed at all on this challenge.
On the return line is it asking me to do something like this?
andren
28,558 Pointsandren
28,558 PointsNo, that would not work. That's not really how you add a value to an array and the task is to only return the removed item, not the entirety of the array + the item added on.
You are on the right track with
shift
andpush
. if you usepush
on the array you can insert theitem
value at the end, this accomplishes the first step of the task.One thing you might not know about the
shift
method is that in addition to removing the first item of an array it also returns said item. This means that you can assign the result of runningarr.shift()
to a variable (or areturn
statement) in order to get a copy of the item that was removed.I feel that should be enough hints. If you are still stuck you can post again and I'll either just post the solution or answer your question if you have other specific questions.
Trevor Johnson
14,427 PointsTrevor Johnson
14,427 PointsI got it
By the way, why does my code always come out orange?
Michael Davis
Courses Plus Student 12,508 PointsMichael Davis
Courses Plus Student 12,508 Pointsadd the name of the syntax you're using after the code marks.
i.e. ```JavaScript
Edit: Edited after receiving valuable pro-tip to escape the back-tick
andren
28,558 Pointsandren
28,558 PointsThat's great.
When using code formatting on this forum you have to type the name of the language you are pasting in after the three backticks that indicate the start of the code block.
So the first line of the code block should look like this:
```JavaScript
If you don't specify a language then everything will be highlighted in orange.
Also Michael Davis pro tip for the future, you can escape the various code formatting options on this forum using \ as an escape character. By using that you can show off the three backticks properly without them being misunderstood by the forum software.