Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial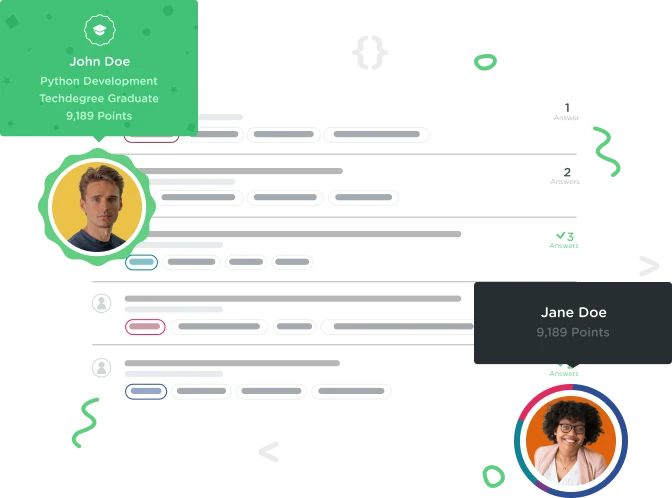
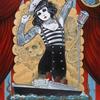
Saira Bottemuller
Courses Plus Student 1,749 PointsJS SyntaxError, having trouble figuring this out.
Hello everyone! :) As always, thanks for taking the time to help out a fellow Treehouser. Here's my quandary. I've been using an altered version of Dave's suggested method in my Random Number Guessing Game, and I've been having a lot of fun with it. Up til now, it's worked great. Now however, I am at the point where I am adding in conditional statements for multiple outcomes, and I knew I'd done something wrong because when I refreshed it in my browser, it no longer worked - no prompt popped up. Each time this has happened, it's been some type of syntax error on my part that I've been able to spot and resolve. This time's been a little more challenging.
I checked the JS Console in my browser, and it lists there's "Uncaught SyntaxError: Unexpected end of input............. script.js:37". So I know this means it's a syntax error on Line 37 (the last line), and the program doesn't know what I'm asking it to do here. The only character on line 37 is my closing curlybrace to end my "else" statement. I have tried tweaking this, removing it, even altering my <p> </p>
paragraph tags, in case I had the syntax in those wrong (see how they start in one set of quotes, and end in another set, within the same document.write
statement? I'm also unsure if that's okay, or if they need to be opened and closed in the same part of each statement ------ but wouldn't that mess up the paragraph effect and break it into a NEW paragraph? Oh my goodness. I guess I have two separate issues, that may possibly be related...or not. So in a nutshell,
1) As far as my <p> </p>
paragraph tags in my final document.write
statement, is my syntax okay there, or can it be improved? This is one of the parts of Dave's program tutorial that I altered to my own taste, but in doing so, I know I run the risk of making mistakes.
2) For the reason my program won't run, if it's not due to the paragraph tags being incorrect or something, what have I done wrong here? Is there a way to drill deeper into the message the JS Console is giving me about the syntax error?
Code is below. Thank you again for your help and feedback, you guys are a huge part of what makes Treehouse so great and so useful. I have never had a better learning experience than I have with Treehouse, and a lot of that is due to the prompt, friendly and knowledgeable help of users and teachers in the forums. You guys rock!
Thanks,
Saira
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6) + 1;
var guess = prompt( "I am thinking of a number between 1 and 6. What is it?");
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if ( parseInt(guess) < randomNumber ) {
var guessMore = prompt("Try again. The number I am thinking of is more than " + guess + ".");
if (parseInt(guessMore) === randomNumber) {
correctGuess = true;
}
} else if ( parseInt(guess) > randomNumber ) {
var guessLess = prompt("Try again. The number I am thinking of is less than " + guess + ".");
if (parseInt(guessLess) === randomNumber) {
correctGuess = true;
}
if ( correctGuess ) {
document.write("<p>You guessed the number, wow!</p>");
} else {
document.write("<p>Sorry, that wasn't quite right. The number was actually " + randomNumber + ". Better luck next time!</p>");
}
4 Answers
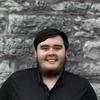
Michael Hulet
47,913 PointsThe "Unexpected end of input" error usually means you're missing a curly brace or something like that somewhere, which is the case here. It happens towards the end of your code, where you forgot to close an if
statement. Here's a fixed version (probably):
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6) + 1;
var guess = prompt( "I am thinking of a number between 1 and 6. What is it?");
if (parseInt(guess) === randomNumber ) {
correctGuess = true;
} else if ( parseInt(guess) < randomNumber ) {
var guessMore = prompt("Try again. The number I am thinking of is more than " + guess + ".");
if (parseInt(guessMore) === randomNumber) {
correctGuess = true;
}
} else if ( parseInt(guess) > randomNumber ) {
var guessLess = prompt("Try again. The number I am thinking of is less than " + guess + ".");
if (parseInt(guessLess) === randomNumber) {
correctGuess = true;
//The curly brace directly below this comment didn't exist in the original code you posted
}
}
if ( correctGuess ) {
document.write("<p>You guessed the number, wow!</p>");
} else {
document.write("<p>Sorry, that wasn't quite right. The number was actually " + randomNumber + ". Better luck next time!</p>");
}
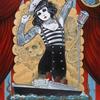
Saira Bottemuller
Courses Plus Student 1,749 PointsMichael you are awesome! Sorry it took a while for me to get back to you, I tested your fixed version in Brackets, and it works beautifully! I can't believe it was something as silly as a missed curlybrace. Do you have any recommended methods for verifying you've closed everything? I guess the method I used didn't work (going over everything several times to spot errors, and then physically counting the number of braces/brackets/parentheses each to verify there are an even number (meaning each one should hypothetically have a "closing partner").... I would love any tips you can give! Simple, silly syntax errors have been my biggest JS hurdle so far. I am trying to form a good habit of writing a SMALL bit of code, testing it, debugging, and then moving on, but in cases like this one, I get so excited about the program and all the ideas flying around in my head that I get carried away, write a big block of code so I don't forget it, and then try to debug it. That's not working out well. What's your strategy? I'm starting to lean toward more documentation/comments, that can be deleted later, to help guide myself through writing the program even if I have to like, leave it alone for a while for some reason.
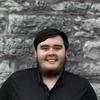
Michael Hulet
47,913 PointsAs Lauri Neuding pointed out below me, a lot of IDEs & Text Editors will insert them for you automatically (It's been a while since I coded in Brackets, so I don't know about it). That's one of the many reasons I love writing apps for iOS in Xcode. If your text editor doesn't insert them automatically, another thing you can do is write the closing bracket yourself before you write any code in between. That way, you can just write as much as you want, and forget about it. Another thing I do is put as little whitespace as possible in my code, but indent every time you write an opening bracket. For example, here's the code above written how I'm describing:
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6) + 1;
var guess = prompt( "I am thinking of a number between 1 and 6. What is it?");
if(parseInt(guess) === randomNumber){
correctGuess = true;
}
else if(parseInt(guess) < randomNumber){
var guessMore = prompt("Try again. The number I am thinking of is more than " + guess + ".");
if (parseInt(guessMore) === randomNumber){
correctGuess = true;
}
}
else if( parseInt(guess) > randomNumber ){
var guessLess = prompt("Try again. The number I am thinking of is less than " + guess + ".");
if(parseInt(guessLess) === randomNumber){
correctGuess = true;
//The curly brace directly below this comment didn't exist in the original code you posted
}
}
if(correctGuess){
document.write("<p>You guessed the number, wow!</p>");
}
else{
document.write("<p>Sorry, that wasn't quite right. The number was actually " + randomNumber + ". Better luck next time!</p>");
}
After reading a strict format like that for a while, the space where there isn't a closing brace looks massive. For example, here's that formatted code again, but without the closing brace that you were originally missing:
var correctGuess = false;
var randomNumber = Math.floor(Math.random() * 6) + 1;
var guess = prompt( "I am thinking of a number between 1 and 6. What is it?");
if(parseInt(guess) === randomNumber){
correctGuess = true;
}
else if(parseInt(guess) < randomNumber){
var guessMore = prompt("Try again. The number I am thinking of is more than " + guess + ".");
if (parseInt(guessMore) === randomNumber){
correctGuess = true;
}
}
else if( parseInt(guess) > randomNumber ){
var guessLess = prompt("Try again. The number I am thinking of is less than " + guess + ".");
if(parseInt(guessLess) === randomNumber){
correctGuess = true;
}
if(correctGuess){
document.write("<p>You guessed the number, wow!</p>");
}
else{
document.write("<p>Sorry, that wasn't quite right. The number was actually " + randomNumber + ". Better luck next time!</p>");
}
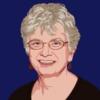
Lauri Neuding
13,525 PointsUsually IDEs will show you matching braces, or anything like parentheses. In Notepad++, for example, highlighting one shows where it is matched. Other IDEs I've used do similar matching. That can really help you out, especially when using nesting.
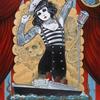
Saira Bottemuller
Courses Plus Student 1,749 PointsThank you guys so much for your help, and the great tips and examples, I will put this to work! :D