Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial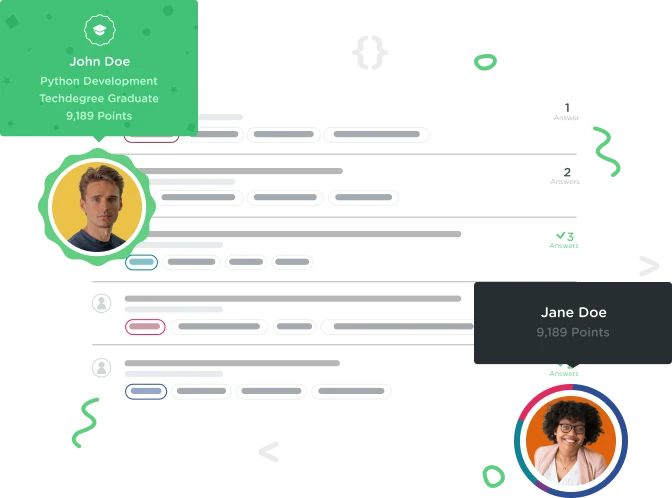

John Weland
42,478 PointsJSON Data not displaying one of its array items...
I actually decided to revamp the Daily Forecast using the RecyclerView and it works. I added in a temperatureMin value too. unfortunately it shows up as '0' in the app even when walking through in debug mode and looking at the parcelables and then stepping over the days return shows 0 for the value yet in my raw json via my forecast link I am clearing getting values for temperatureMin. If you don't mind taking a look I am sure its something simple I am missing. I appreciate it.
DayAdapter.java
package johnweland.me.stormy.adapters;
import android.content.Context;
import android.net.ConnectivityManager;
import android.support.v7.widget.RecyclerView;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.BaseAdapter;
import android.widget.ImageView;
import android.widget.TextView;
import android.widget.Toast;
import org.w3c.dom.Text;
import johnweland.me.stormy.R;
import johnweland.me.stormy.weather.Day;
/**
* Created by jweland on 5/27/2015.
*/
public class DayAdapter extends RecyclerView.Adapter<DayAdapter.DayViewHolder> {
private Day[] mDays;
private Context mContext;
public DayAdapter (Context context, Day[] days){
mContext = context;
mDays = days;
}
@Override
public DayViewHolder onCreateViewHolder(ViewGroup parent, int viewType) {
View view = LayoutInflater.from(parent.getContext())
.inflate(R.layout.daily_list_item, parent, false);
DayViewHolder viewHolder = new DayViewHolder(view);
return viewHolder;
}
@Override
public void onBindViewHolder(DayViewHolder holder, int position) {
holder.bindDay(mDays[position]);
}
@Override
public int getItemCount() {
return mDays.length;
}
public class DayViewHolder extends RecyclerView.ViewHolder
implements View.OnClickListener {
public TextView mSummaryLabel;
public TextView mTemperatureMaxLabel;
public TextView mTemperatureMinLabel;
public TextView mDayNameLabel;
public ImageView mIconImageView;
public DayViewHolder(View itemView) {
super(itemView);
mTemperatureMaxLabel = (TextView) itemView.findViewById(R.id.temperatureMaxLabel);
mTemperatureMinLabel = (TextView) itemView.findViewById(R.id.temperatureMinLabel);
mSummaryLabel = (TextView) itemView.findViewById(R.id.summaryLabel);
mDayNameLabel = (TextView) itemView.findViewById(R.id.dayNameLabel);
mIconImageView = (ImageView) itemView.findViewById(R.id.iconImageView);
itemView.setOnClickListener(this);
}
public void bindDay(Day day) {
mDayNameLabel.setText(day.getDayOfTheWeek());
mTemperatureMaxLabel.setText(day.getTemperatureMax() + "");
mTemperatureMinLabel.setText(day.getTemperatureMin() + "");
mIconImageView.setImageResource(day.getIconId());
}
@Override
public void onClick(View v) {
String day = mDayNameLabel.getText().toString();
String summary = mSummaryLabel.getText().toString();
String temperatureMax = mTemperatureMaxLabel.getText().toString();
String temperatureMin = mTemperatureMinLabel.getText().toString();
String message = String.format("For %s it will be %s the high is %s with a low of %s",
day,
summary,
temperatureMax,
temperatureMin);
Toast.makeText(mContext, message, Toast.LENGTH_LONG).show();
}
}
}
Day.java
package johnweland.me.stormy.weather;
import android.os.Parcel;
import android.os.Parcelable;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
/**
* Created by jweland on 5/27/2015.
*/
public class Day implements Parcelable{
private long mTime;
private String mSummary;
private double mTemperatureMax;
private double mTemperatureMin;
private String mIcon;
private String mTimezone;
public Day() {}
public long getTime() {
return mTime;
}
public void setTime(long mTime) {
this.mTime = mTime;
}
public String getSummary() {
return mSummary;
}
public void setSummary(String summary) {
mSummary = summary;
}
public int getTemperatureMax() {
return (int) Math.round(mTemperatureMax);
}
public void setTemperatureMax(double temperatureMax) {
mTemperatureMax = temperatureMax;
}
public int getTemperatureMin() { return (int) Math.round(mTemperatureMin); }
public void setTemperatureMin(double temperatureMin) { mTemperatureMin = temperatureMin; }
public String getIcon() {
return mIcon;
}
public int getIconId(){
return Forecast.getIconId(mIcon);
}
public void setIcon(String icon) {
mIcon = icon;
}
public String getTimezone() {
return mTimezone;
}
public void setTimezone(String timezone) {
mTimezone = timezone;
}
public String getDayOfTheWeek() {
SimpleDateFormat formatter = new SimpleDateFormat("EEEE");
formatter.setTimeZone(TimeZone.getTimeZone(mTimezone));
Date date = new Date(mTime * 1000);
return formatter.format(date);
}
@Override
public int describeContents() {
return 0; // ingore
}
@Override
public void writeToParcel(Parcel dest, int flags) {
dest.writeLong(mTime);
dest.writeDouble(mTemperatureMax);
dest.writeDouble(mTemperatureMin);
dest.writeString(mSummary);
dest.writeString(mIcon);
dest.writeString(mTimezone);
}
private Day (Parcel in) {
mTime = in.readLong();
mTemperatureMax = in.readDouble();
mTemperatureMin = in.readDouble();
mSummary = in.readString();
mIcon = in.readString();
mTimezone = in.readString();
}
public static final Creator<Day> CREATOR = new Creator<Day>() {
@Override
public Day createFromParcel(Parcel source) {
return new Day(source);
}
@Override
public Day[] newArray(int size) {
return new Day[size];
}
};
}
Daily Forecast Activity
package johnweland.me.stormy.ui;
import android.content.Intent;
import android.os.Parcelable;
import android.support.v7.app.ActionBarActivity;
import android.os.Bundle;
import android.support.v7.widget.LinearLayoutManager;
import android.support.v7.widget.RecyclerView;
import android.view.Menu;
import android.view.MenuItem;
import android.widget.ArrayAdapter;
import java.util.Arrays;
import butterknife.ButterKnife;
import butterknife.InjectView;
import johnweland.me.stormy.R;
import johnweland.me.stormy.adapters.DayAdapter;
import johnweland.me.stormy.weather.Day;
public class DailyForecastActivity extends ActionBarActivity {
private Day[] mDays;
@InjectView(R.id.recyclerView) RecyclerView mRecyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_daily_forecast);
ButterKnife.inject(this);
Intent intent = getIntent();
Parcelable[] parcelables = intent.getParcelableArrayExtra(MainActivity.DAILY_FORECAST);
mDays = Arrays.copyOf(parcelables, parcelables.length, Day[].class);
DayAdapter adapter = new DayAdapter(this, mDays);
mRecyclerView.setAdapter(adapter);
RecyclerView.LayoutManager layoutManager = new LinearLayoutManager(this);
mRecyclerView.setLayoutManager(layoutManager);
mRecyclerView.setHasFixedSize(true);
}
}
daily_list_item.xml
<?xml version="1.0" encoding="utf-8"?>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:paddingLeft="64dp"
android:paddingRight="32dp"
android:paddingTop="4dp"
android:paddingBottom="4dp"
tools:background="@drawable/bg_gradient">
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/circleImageView"
android:layout_alignParentTop="true"
android:layout_alignParentLeft="true"
android:layout_alignParentStart="true"
android:src="@drawable/bg_temperature"/>
<ImageView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/iconImageView"
android:layout_centerVertical="true"
android:layout_toRightOf="@+id/circleImageView"
android:layout_toEndOf="@+id/circleImageView"
android:paddingLeft="10dp"
android:src="@drawable/clear_day"/>
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:id="@+id/dayNameLabel"
android:layout_centerVertical="true"
android:layout_toRightOf="@+id/iconImageView"
android:layout_toEndOf="@+id/iconImageView"
android:textColor="#FFFFFFFF"
android:textSize="20sp"
android:paddingLeft="10dp"
tools:text="Wednesday"/>
<LinearLayout
android:orientation="vertical"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_alignTop="@+id/circleImageView"
android:layout_alignBottom="@+id/circleImageView"
android:layout_alignLeft="@+id/circleImageView"
android:layout_alignRight="@+id/circleImageView"
android:layout_centerVertical="true"
android:paddingTop="5dp"
android:paddingBottom="5dp">
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/temperatureMaxLabel"
android:gravity="center"
android:textColor="#f25019"
tools:text="-100"
android:layout_weight="0"
/>
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:id="@+id/temperatureMixLabel"
android:gravity="center"
android:textColor="#f25019"
tools:text="-240"
android:layout_weight="0"
/>
</LinearLayout>
</RelativeLayout>
1 Answer

Sam Wilskey
3,420 PointsWell again I think that the code that you are missing is not in the files that you provided. The issue I believe is that the instance variable mTemperatureMin is not being set in MainActivity. In the function getDailyForecast in MainActivity make sure that you set the min temperature when reading the jsondata.
Example with possible variable names. Make sure that you use the ones you have.
day.setTemperatureMin(jsonDay.getDouble("temperatureMin"));

John Weland
42,478 PointsDude, awesome!
John Weland
42,478 PointsJohn Weland
42,478 PointsSam Wilskey I thought I'd create another topic and tag you in it so if you have a moment and can help I an select the best answer and upvote you.