Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial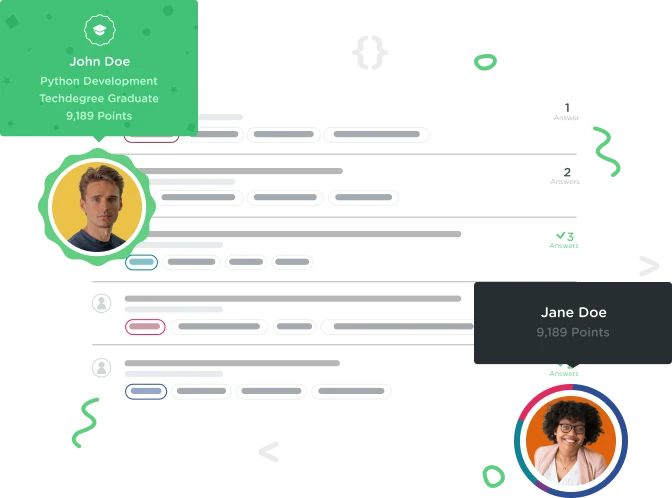

Samme Qandil
1,228 PointsJSON Eception no value for timezone
package io.github.anoobishnoob.stormy;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.TimeZone;
/**
* Created by saqan on 6/25/2018.
*/
public class CurrentWeather {
private String locationLabel;
private String icon;
private long time;
private double temperature;
private double humidity;
private double precipChance;
private String summary;
private String timeZone;
public String getTimeZone() {
return timeZone;
}
public void setTimeZone(String timeZone) {
this.timeZone = timeZone;
}
public String getLocationLabel() {
return locationLabel;
}
public void setLocationLabel(String locationLabel) {
this.locationLabel = locationLabel;
}
public String getIcon() {
return icon;
}
public void setIcon(String icon) {
this.icon = icon;
}
public long getTime() {
return time;
}
public String getFormattedTime(){
SimpleDateFormat formatter = new SimpleDateFormat("h:mm a");
formatter.setTimeZone(TimeZone.getTimeZone(timeZone));
Date dateTime = new Date(time *1000);
return formatter.format(dateTime);
}
public void setTime(long time) {
this.time = time;
}
public double getTemperature() {
return temperature;
}
public void setTemperature(double temperature) {
this.temperature = temperature;
}
public double getHumidity() {
return humidity;
}
public void setHumidity(double humidity) {
this.humidity = humidity;
}
public double getPrecipChance() {
return precipChance;
}
public void setPrecipChance(double precipChance) {
this.precipChance = precipChance;
}
public String getSummary() {
return summary;
}
public void setSummary(String summary) {
this.summary = summary;
}
}
/////////// end of this class//////////////////////
/////////////main activity below////////////////
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException{
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("Timezone");
Log.i(TAG,"From JSON:" + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setLocationLabel("Alcatraz Island, CA");
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("tempature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return currentWeather;
}
I can't get this bug out. Any clue guys?
Here is the Log
E/MainActivity: JSON eception
org.json.JSONException: No value for Timezone
at org.json.JSONObject.get(JSONObject.java:392)
at org.json.JSONObject.getString(JSONObject.java:553)
at io.github.anoobishnoob.stormy.MainActivity.getCurrentDetails(MainActivity.java:88)
at io.github.anoobishnoob.stormy.MainActivity.access$100(MainActivity.java:24)
at io.github.anoobishnoob.stormy.MainActivity$1.onResponse(MainActivity.java:67)
at okhttp3.RealCall$AsyncCall.execute(RealCall.java:153)
at okhttp3.internal.NamedRunnable.run(NamedRunnable.java:32)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1162)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:636)
at java.lang.Thread.run(Thread.java:764)
Mod Note - added Forum markdown for clarity on code. Checkout the Markdown Basics course on Treehouse to learn about Markdown :)
[MOD2 - edited code block to be Java]
2 Answers
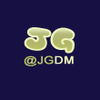
Jonathan Grieve
Treehouse Moderator 91,253 PointsThis might be too simple an answer but in your line where you're setting the string for Timezone try changing
String timezone = forecast.getString("Timezone");
to
String timezone = forecast.getString("timezone");

Samme Qandil
1,228 PointsThanks man :)