Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial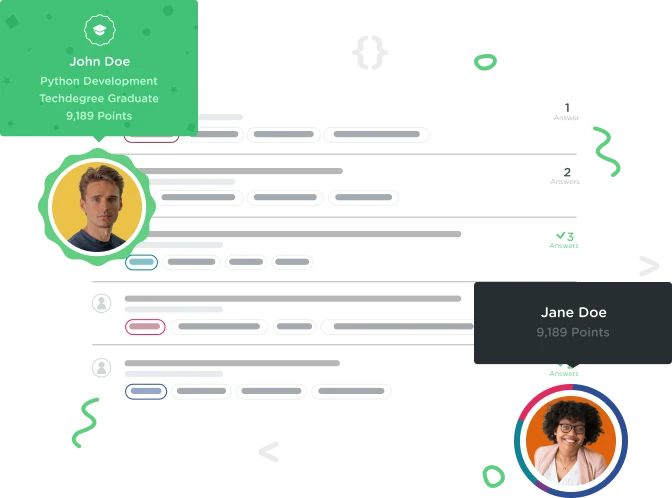
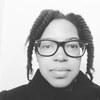
Sharina Jones
Courses Plus Student 18,771 PointsJSON req.body not appearing in Postman
I'm getting the "You sent me a POST request" message, however, the body information doesn't appear in my POST route.
I've verified the info is still in the Postman body.
//app.js
'use strict';
var express = require('express');
var app = express();
const routes = require('./routes');
app.use("/questions", routes);
const jsonParser = require('body-parser').json;
app.use(function(req, res, next) {
req.body;
next();
});
//routes.js
'use strict';
const express = require('express');
const router = express.Router();
// GET /questions
//returns all questions
//next isn't required
router.get("/", function (req, res) {
res.json({response: "You sent me a GET request"});
});
// POST /questions
//route for creating questions
router.post("/", function (req, res) {
res.json({
response: "You sent me a POST request",
body: req.body
});
});
// GET /question/:id
//retrieves a specific question
router.get("/:id", function (req, res, next) {
res.json({
response: "You sent me a GET request for ID " + req.params.id
});
});
module.exports = router;
1 Answer

Brian Anstett
5,831 PointsHey Sharina Jones, It looks like you forgot to attach ('use') your json body parser.
Recall you can use the .use() function in express to mount a specific middleware function. (https://expressjs.com/en/4x/api.html#app.use)
So you need to add something like this
var express = require('express')
var bodyParser = require('body-parser')
var app = express()
app.use(bodyParser.json())
Also note, in express.js v4, you don't even need to use the npm 'body-parser' module to parse incoming request bodies because express has a native function called .json() that can do it for you. https://expressjs.com/en/4x/api.html#express.json
Sharina Jones
Courses Plus Student 18,771 PointsSharina Jones
Courses Plus Student 18,771 PointsThanks Brian Anstett!