Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial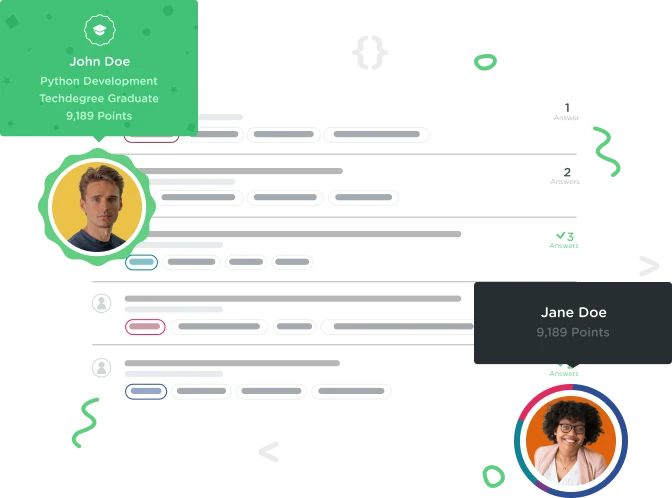

William Jaggers
8,109 PointsJSON.parse error - unexpected token (Javascript)
I am trying to access some JSON data using Javascript. My example is very similar to the employees example in the AJAX unit for Javascript. But when I try to parse the JSON data, it returns an error. Google tells me that it is because I am returning an object and JSON.parse expects a string, so I should use JSON.stringify inside of the parse. But then I can't access the data as an array. Can anyone shed any light on this? It seemed to work fine to return an object in the lesson example. I will show the code/data below that I was basing my effort on, as well as the code/data that I am trying to use.
Lesson example:
var xhr = new XMLHttpRequest(); xhr.open('GET', '../data/employees.json'); xhr.onreadystatechange = function () { if(xhr.readyState === 4 && xhr.status === 200) { var employees = JSON.parse(xhr.responseText); for (var i=0; i<employees.length; i += 1) { ..... }
JSON data: [ { "name": "Aimee", "inoffice": false },
{ "name": "Zac", "inoffice": false } ]
My example:
var xhr = new XMLHttpRequest(); xhr.open('GET', 'locations.json'); xhr.onreadystatechange = function () { if (xhr.readyState === 4) { var locations = JSON.parse(xhr.responseText); var first = locations[0]; //test ..... }
JSON data: [ { "location": "Woods Chapel", "streetAddr": "917 Woods Chapel Rd", "firstItem": "Mon - Thu 11:00AM - 9:30PM", "secondItem": "Fri & Sat 11:00AM - 10:00PM", "thirdItem": "Sun 11:30AM - 9:00PM"},
{ "location": "Raintree", "streetAddr": "899 SW LeMans Lane", "firstItem": "Mon - Thu 11:00AM - 9:30PM", "secondItem": "Fri & Sat 11:00AM - 10:00PM", "thirdItem": "Sun 11:30AM - 9:00PM"} ] locations.html:4 Uncaught SyntaxError: Unexpected token L in JSON at position 94
4 Answers
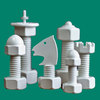
Steven Parker
230,274 PointsThere's an error in the data itself.
Here's the beginning of the responseText:
[
{ "location": "Woods Chapel",
"streetAddr": "917 Woods Chapel Rd",
"cityStateZip: "Lee's Summit, MO 64064",
Note that the key cityStateZip has no closing quote mark. This causes the next quote mark to be interpreted as the end of that string, and the "L" immediately following it is the "unexpected token".
If you look through the objects in the array, each one has the same error on the line with that key.
As a work-around until the server bug is fixed, you might try this:
const locations = JSON.parse(xhr.responseText.replace(/cityStateZip:/g, 'cityStateZip":'));
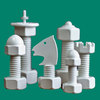
Steven Parker
230,274 PointsYou may need to provide a more complete project.
I tried substituting the xhr.responseText with your JSON data, but I had no trouble travsersing the array returned by JSON.parse. Could you provide a link to a workspace snapshot or a github repo to facilitate an analysis of the issue?
Also, when posting code here, be sure to use the instructions for code formatting in the Markdown Cheatsheet pop-up below the "Add an Answer" area.

William Jaggers
8,109 PointsHi Steven,
Thanks for looking over my problem! I will for sure check out the Markdown Cheatsheet, as I know my code isn't appearing the way I entered it. I also wanted to add that i am very new at this, so i may be doing some very noobish things! That said, I have been trying to create a website for my portfolio, taking a site that has problems and trying to fix some of them. This example is using the website for LaFuente, a mexican restaurant, as my model. I have been writing code in SublimeText, and haven't started using GitHub yet, so the best place to look at the whole project might be on the web, if that is acceptable. I don't know if you can see the JSON data that way, but it is the same (just a little longer) as what I posted initially. I do see the JSON data in the xhr.responseText before i try to parse it, but I can see that I am still getting the same error. Please check out the site at www.bill.jaggers.cloud/LaFuente/lafuente.html, and click on the locations nav button. This is the page I am trying to build. I tested it and it should work for you; but if not please let me know and i will try to find a better way to do it. I think you can inspect (in Chrome) the page, and view the JS source to see the problem happening.
Thanks again!

William Jaggers
8,109 PointsNot the first time my eyes have failed me. I looked hard at the data, thinking there could be something wrong there, but I just couldn't see it. Wish there was a way to see what is happening in more detail at execution time. Anyway, thanks very much for spending your time to help me out. I'm very grateful. I'll try and pay it forward if I ever get any good at this stuff!