Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial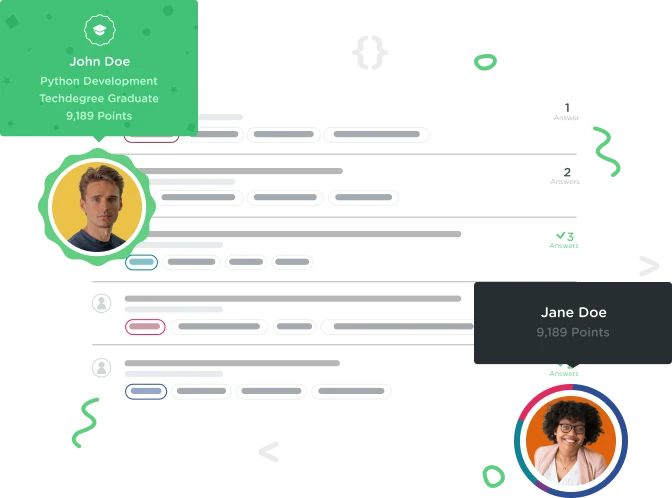
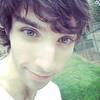
Alphonse Cuccurullo
2,513 PointsJust curious guys. Is it possible to write a class without initializing? Like maybe with the attr_accessor?
Cause i saw a video in which he did this and now im just alittle confused on what initializing is for and the difference between that and the attr_reader, writer and accessors.
3 Answers
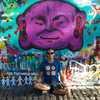
Andrew Stelmach
12,583 PointsYou can of course write a class without initialising it, but it has to be instantiated (initialised) for it to do anything. You haven't been very descriptive in your question so I don't know exactly how to answer it, but a few key points:
You typically write an initialize method (which is run when you instantiate the class with eg SomeClass.new
) when you want to something to happen when the class is instantiated.
A common usage of the initialize method is to assign values to instance variables which are then accessed through attr_reader
. These are typically called 'attributes' (I think).
attr_reader
allows you to do:
variable_assigned_to_an_instance_of_a_class.some_attribute
=> attribute's value
attr_writer
allows you to change an attribute's value.
attr_accessor
rolls attr_reader
and attr_writer
into one.
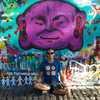
Andrew Stelmach
12,583 PointsRe your first question - less code is usually more :-)
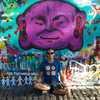
Andrew Stelmach
12,583 PointsHaving attr_accessor
unnecessarily might well be confusing to another developer looking at your code, too, which is bad. Using just one of them when you only need one will probably make your code more semantic and readable and is better practice.
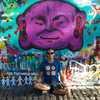
Andrew Stelmach
12,583 PointsYup. First of all, forget about the initialize method for a moment. Erase it from your memory, then read this: http://stackoverflow.com/questions/5046831/why-use-rubys-attr-accessor-attr-reader-and-attr-writer
Remember what an instance variable is - it's a variable that belongs to an instance of a class i.e. it is accessible throughout that specific instance of that specific class and nowhere else.
Have you read that link? If not, do that now.
So in the video he assigns an instance of that class to a variable. Then he uses the method PROVIDED BY attr_writer
to create an instance variable inside the instance of the class.
class AwesomeClass
attr_writer :name
end
an_instance = AwesomeClass.new
an_instance.name = "Andrew"
Then, he uses the method PROVIDED BY attr_reader
to print out the @name
instance variable. eg:
class AwesomeClass
attr_writer :name
attr_reader :name
end
an_instance = AwesomeClass.new
an_instance.name = "Andrew"
an_instance.name
=> "Andrew"
Now, moving on to the initialize method... if you want @name to be created and assigned a value when you instantiate your class, you can do so, for example:
class AwesomeClass
attr_reader :name
def initialize
@name = "Andrew"
end
end
an_instance = AwesomeClass.new
an_instance.name
=> "Andrew"
If you want to be able to change this value, you can add an attr_writer. Since we already have an attr_reader, it makes sense to remove attr_reader and just have attr_accessor:
class AwesomeClass
attr_accessor :name
def initialize
@name = "Nobrot"
end
end
an_instance = AwesomeClass.new
an_instance.name
=> "Nobrot"
an_instance.name = "Andrew"
an_instance.name
=> "Andrew"
Any questions, fire away.
Alphonse Cuccurullo
2,513 PointsAlphonse Cuccurullo
2,513 PointsSo is the attr_reader, and attr_writer really neccessary? I mean if the accessor is both whats the point of the other's?
https://www.youtube.com/watch?v=qXG-UKVgdbM
This is the link in which the guy didn't use the initialize method and top it off he instantiated a object. Can you explain why by any chance?