Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial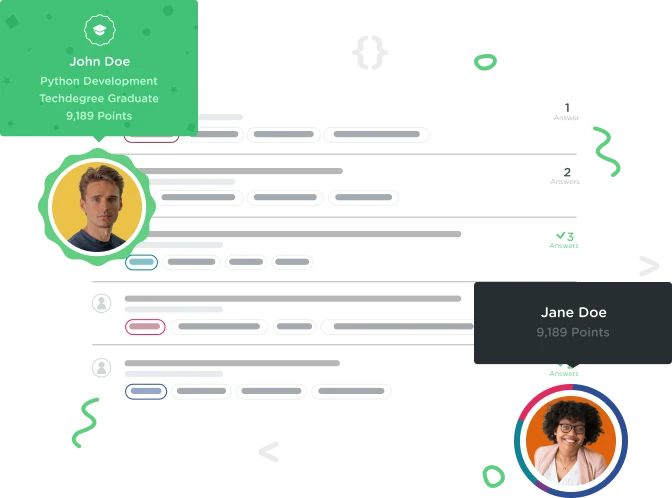
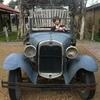
Brendan Moran
14,052 PointsJust for fun: Allowing negative integers and 0 for both numbers, testing for high > low
Something to allow the user to provide any integer they want, positive or negative, as long as the highNumber is greater than the lowNumber. It requires a crazy long conditional statement so that we aren't limited to just the positive integers and so that the program isn't stopped by the falsey zero input on either number. The test for the greater number needs to come first so that we don't get some weirdness in the results. The inverse could have been thrown in to the giant "success test" that follows, but that would have been harder to read, and I wanted to give more specific feedback to the user.
if (lowNumber >= highNumber) {
alert('The second number you provide needs to be greater than the first number. Please try again!');
location.reload();
} else if (lowNumber && highNumber || lowNumber === 0 && highNumber || lowNumber && highNumber === 0) {
// Use Math.random() and the user's numbers to generate a random number
const randomNum = Math.floor(Math.random() * (highNumber - lowNumber + 1)) + lowNumber;
// Create a message displaying the random number
alert(`${randomNum} is a random number between ${lowNumber} and ${highNumber}. Let's do it again!`);
location.reload();
} else {
alert('It appears that one of the values you provided was not a number. You need to type in a number for both prompts. Please try again!');
location.reload();
}