Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial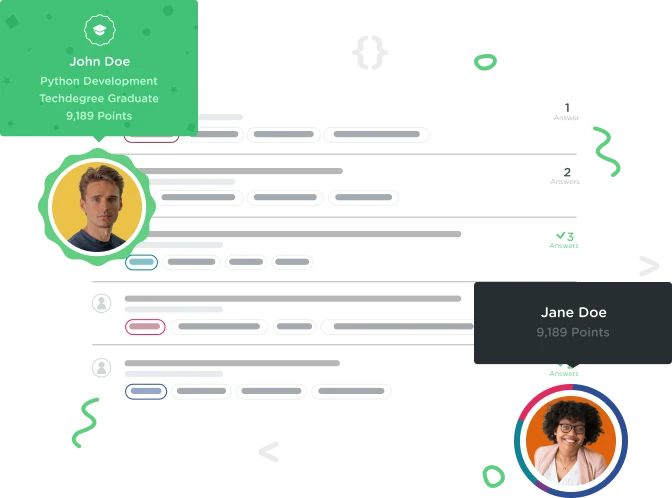

Saif Khan
4,866 PointsJust need some clarification on JavaScript Scope
var world= "World!";
function sayHello () {
var hello = "Hello ";
function inner() {
var extra = " There is more!"
console.log(hello + world + extra);
}
inner();
}
sayHello();
console.log("world outside sayHello(): ", world);
console.log("hello inside sayHello(): ", hello);
//In this function, I was playing around with the console, changed the outside to inside. I don't understand why the var hello won't execute and responds with an error even though I said console.log("hello inside sayHello(): ", hello); ? Isn't the var hello nested inside the function sayHello above?
2 Answers
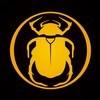
rydavim
18,814 PointsThe variable 'hello' only exists inside the sayHello() function. So once you're back out into the main program where you call console.log, you can't see that variable anymore. From the outside, you can only see world and sayHello().
If you tried putting the console.log call inside the sayHello function, it would print.
var world= "World!";
function sayHello () {
var hello = "Hello ";
function inner() {
var extra = " There is more!"
console.log(hello + world + extra);
}
inner();
// For example, if you put it here...
console.log("hello inside sayHello(): ", hello);
}
sayHello();
console.log("world outside sayHello(): ", world);
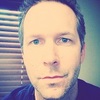
Alex Friant
6,444 Points@ryadavim's answer above is correct, but I want to expand.
I'm not sure, but it looks like you are trying to pass the hello variable to the function, in which you would want to write it something like this:
var hello = "Hello";
var world = " World!";
function sayHello (message) {
var hello = message;
function inner() {
var extra = " There is more!"
console.log(hello + world + extra);
}
inner();
}
This would allow you to pass the global hello variable to the function.
console.log(sayHello(hello));
-->Hello World! There is more!
You could also pass the global world variable to the function.
console.log(sayHello(world));
-->World! World! There is more!
And since the function can now accept variables, you can pass in anything you like.
console.log(sayHello("Howdy"));
-->Howdy World! There is more!
Now what happens when you write this?
console.log(sayHello("Howdy"), hello);
You'll see that the inner hello variable, although it gets assigned the "Howdy" string has no effect on the global outer variable "Hello". They are completely separate variables. One has global scope, and the other has scope only to the sayHello function and all the functions inside it.

Saif Khan
4,866 PointsThank so much Alex, really gave me a great understanding. I think I'm getting it.
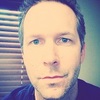
Alex Friant
6,444 PointsCool! Now if you could just help me understand recursive functions (functions that call themselves)! LOL
Cheers!
Saif Khan
4,866 PointsSaif Khan
4,866 PointsThanks so much
So that variable becomes useless if you need to call on it later since it's only defined within a function? What if its defined inside the function and also in the global scope? Is that possible to call on it from outside the function in that scenario? Can a variable be defined twice?
rydavim
18,814 Pointsrydavim
18,814 PointsIf you continue in the JavaScript courses they'll go into this is greater depth, but here are some quick and dirty answers.
If you're going to want to use a variable you declare in a function later in your program, you probably want to return that variable at the end of the function. This lets your program save the value of something you did inside the function, without creating a global variable for it. You'll do this a lot.
You can define something both globally and locally in a function. However, it probably won't behave the way you would expect. You'll run into something called hoisting. Treehouse actually has a whole video on this.
Saif Khan
4,866 PointsSaif Khan
4,866 PointsThanks so much! I see what you are saying. Haha i guess I'm jumping ahead of the videos in my thought process.