Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial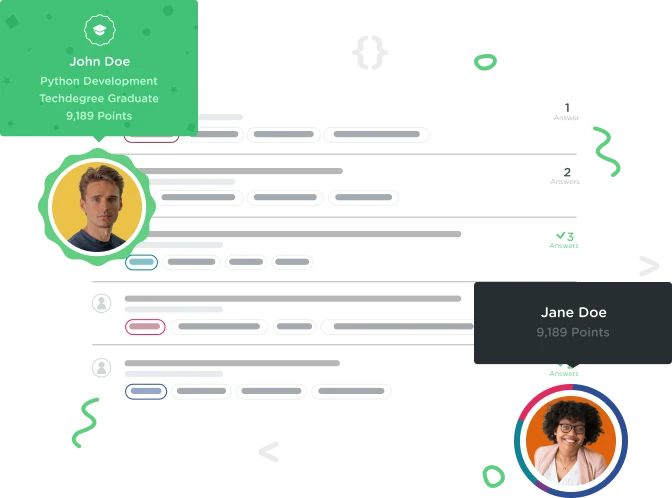
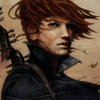
Benjamin Earle
6,204 PointsJust noticed a bug and dont know how to fix it!
Whenever I go to the edit friends page, and then go back, the dialog for choosing the media action (take photo, take viedeo, choose photo, choose video) opens. Here's my main activity:
package com.benjamin.snapshot;
import android.app.AlertDialog;
import android.content.DialogInterface;
import android.content.Intent;
import android.net.Uri;
import android.os.Bundle;
import android.os.Environment;
import android.provider.MediaStore;
import android.support.v4.app.FragmentPagerAdapter;
import android.support.v4.app.FragmentTransaction;
import android.support.v4.view.ViewPager;
import android.support.v7.app.ActionBar;
import android.support.v7.app.ActionBarActivity;
import android.util.Log;
import android.view.Menu;
import android.view.MenuItem;
import android.view.Window;
import android.widget.Toast;
import com.parse.ParseAnalytics;
import com.parse.ParseUser;
import java.io.File;
import java.text.SimpleDateFormat;
import java.util.Date;
import java.util.Locale;
public class MainActivity extends ActionBarActivity implements ActionBar.TabListener {
public static final String TAG = MainActivity.class.getSimpleName();
public static final int TAKE_PHOTO_REQUEST = 0;
public static final int TAKE_VIDEO_REQUEST = 1;
public static final int PICK_PHOTO_REQUEST = 2;
public static final int PICK_VIDEO_REQUEST = 3;
public static final int MEDIA_TYPE_IAMGE = 4;
public static final int MEDIA_TYPE_VIDEO = 5;
protected Uri mMediaUri;
/**
* The {@link android.support.v4.view.PagerAdapter} that will provide
* fragments for each of the sections. We use a
* {@link FragmentPagerAdapter} derivative, which will keep every
* loaded fragment in memory. If this becomes too memory intensive, it
* may be best to switch to a
* {@link android.support.v4.app.FragmentStatePagerAdapter}.
*/
SectionsPagerAdapter mSectionsPagerAdapter;
protected DialogInterface.OnClickListener mDialogListener = new DialogInterface.OnClickListener(){
@Override
public void onClick(DialogInterface dialog, int which) {
switch(which){
case 0:
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IAMGE);
if(mMediaUri == null){
//display error
Toast.makeText(MainActivity.this, R.string.SDCard_error, Toast.LENGTH_LONG).show();
} else {
}
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
break;
case 1:
break;
case 2:
break;
case 3:
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
if(isExternalStorageAvailable()){
//get Uri
//Get external storage directory
File mediaStorageDir = new File(Environment.getExternalStoragePublicDirectory(Environment.DIRECTORY_PICTURES),
MainActivity.this.getString(R.string.app_name));
//Create subdirectory
if (! mediaStorageDir.exists()){
if(! mediaStorageDir.mkdir()){
Log.e(TAG, "Failed to create directory");
return null;
}
}
//Create file name
//Create file
File mediaFile;
Date now = new Date();
String timeStamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
if (mediaType == MEDIA_TYPE_IAMGE){
mediaFile = new File(path + "IMG_" + timeStamp + ".jpg");
} else if (mediaType == MEDIA_TYPE_VIDEO){
mediaFile = new File(path + "VID_" + timeStamp + ".mp4");
} else {
return null;
}
Log.d(TAG, "File: " + Uri.fromFile(mediaFile));
//Return file's Uri
return Uri.fromFile(mediaFile);
}else {
return null;
}
}
private boolean isExternalStorageAvailable(){
String state = Environment.getExternalStorageState();
if(state.equals(Environment.MEDIA_MOUNTED)){
return true;
} else{
return false;
}
}
};
/**
* The {@link ViewPager} that will host the section contents.
*/
ViewPager mViewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
supportRequestWindowFeature(Window.FEATURE_INDETERMINATE_PROGRESS);
setContentView(R.layout.activity_main);
ParseAnalytics.trackAppOpenedInBackground(getIntent());
ParseUser currentUser = ParseUser.getCurrentUser();
if (currentUser == null) {
navigateToLogin();
}
else{
Log.i(TAG, currentUser.getUsername());
}
// Set up the action bar.
final ActionBar actionBar = getSupportActionBar();
actionBar.setNavigationMode(ActionBar.NAVIGATION_MODE_TABS);
// Create the adapter that will return a fragment for each of the three
// primary sections of the activity.
mSectionsPagerAdapter = new SectionsPagerAdapter(this, getSupportFragmentManager());
// Set up the ViewPager with the sections adapter.
mViewPager = (ViewPager) findViewById(R.id.pager);
mViewPager.setAdapter(mSectionsPagerAdapter);
// When swiping between different sections, select the corresponding
// tab. We can also use ActionBar.Tab#select() to do this if we have
// a reference to the Tab.
mViewPager.setOnPageChangeListener(new ViewPager.SimpleOnPageChangeListener() {
@Override
public void onPageSelected(int position) {
actionBar.setSelectedNavigationItem(position);
}
});
// For each of the sections in the app, add a tab to the action bar.
for (int i = 0; i < mSectionsPagerAdapter.getCount(); i++) {
// Create a tab with text corresponding to the page title defined by
// the adapter. Also specify this Activity object, which implements
// the TabListener interface, as the callback (listener) for when
// this tab is selected.
actionBar.addTab(
actionBar.newTab()
.setText(mSectionsPagerAdapter.getPageTitle(i))
.setTabListener(this));
}
}
private void navigateToLogin() {
Intent intent = new Intent(this, LoginActivity.class);
intent.addFlags(Intent.FLAG_ACTIVITY_NEW_TASK);
intent.addFlags(Intent.FLAG_ACTIVITY_CLEAR_TASK);
startActivity(intent);
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
int itemId = item.getItemId();
switch (itemId){
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
case R.id.action_camera:
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
}
return super.onOptionsItemSelected(item);
}
@Override
public void onTabSelected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
// When the given tab is selected, switch to the corresponding page in
// the ViewPager.
mViewPager.setCurrentItem(tab.getPosition());
}
@Override
public void onTabUnselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
@Override
public void onTabReselected(ActionBar.Tab tab, FragmentTransaction fragmentTransaction) {
}
}
Update
I just noticed that its not only when I go to the Edit Friends screen. The real bug is that when I click on one of the options from the menu, it also, for I dont know what reason, runs the code for the next menu item. For example: If I click the camera, it all runs as it should, because it has no menu item after it. If I click Edit Friends, it runs the code for when I click on the camera icon as well as the code for Edit Friends. And finally, if I click on Log Out, it tries to open the Edit Friends screen while logging out, making the app crash. Please, dose someone have an idea of how to fix it?
8 Answers
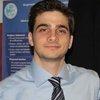
adamabdulghani
7,864 PointsHi Benjamin!
Glad that you found a work around, but i believe that a switch statement is much better to use in this case, because its much cleaner code that nested if elses. anyway in the switch statement you have to add a break statement after each case, unless you want to continue and execute the second case's code. In other words:
switch (itemId){
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
break;
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
break;
case R.id.action_camera:
Toast.makeText(this, "Asdf", Toast.LENGTH_LONG).show();
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
break;
default: //this is good to have, this will be reached if none of the above cases match to the switchId value
Log.i(TAG,"oh oh case "+itemId+"isn't handled here");
break;
}
by adding a break you're getting out of the switch statement and not running the next case. I recommend you read up on the switch statement in the docs i linked.
From the docs: Another point of interest is the break statement. Each break statement terminates the enclosing switch statement. Control flow continues with the first statement following the switch block. The break statements are necessary because without them, statements in switch blocks fall through
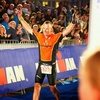
Steve Hunter
57,712 PointsHi Benjamin,
The code here looks OK, although there is a lot of it!!
Generally, though, the menu buttons are either coded in the onOptionsItemSelected
method as you have done. However, the home/up/back commands are handled by the action bar itself. The .xml in the Android Manifest will tell you where the click will lead you to.
Have a look to see if the ".EditFriendsActivity"
has a parent activity set correctly.
Steve.
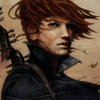
Benjamin Earle
6,204 PointsIt does:
<activity
android:name=".EditFriendsActivity"
android:label="@string/title_activity_edit_friends"
android:parentActivityName=".MainActivity"
android:screenOrientation="portrait" >
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="com.benjamin.snapshot.MainActivity" />
</activity>
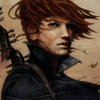
Benjamin Earle
6,204 PointsI cant make it show, but theres a last line, which is the activity closing
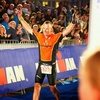
Steve Hunter
57,712 PointsSomething like:
<activity
android:name=".EditFriendsActivity"
android:label="@string/something"
<meta-data
android:name="android.support.PARENT_ACTIVITY"
android:value="uk.co.website.ribbit.InboxActivity" />
</activity>
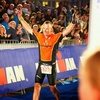
Steve Hunter
57,712 PointsAh right - so it isn't that then.
Weird.
Have you put some Toasts around to see what path your code is taking?
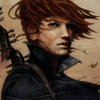
Benjamin Earle
6,204 Pointsummm, Im not sure what you mean
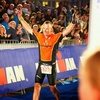
Steve Hunter
57,712 PointsAdd a few pop-up messages around just to see if the code is going directly to the wrong Intent or if it is taking a circuitous route.
I can't see how you can get from EditFriends to the camera picker - I'd be interested to see what it is doing!
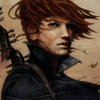
Benjamin Earle
6,204 PointsI just made a quick and sloppy video of what happens visually:
https://www.youtube.com/watch?v=XkLO6RatYqA
I added a toast for, supposedly, the camera icon is tapped on, but when I go to edit friends it apears...
I added this (Just the toast message):
switch (itemId){
case R.id.action_logout:
ParseUser.logOut();
navigateToLogin();
case R.id.action_edit_friends:
Intent intent = new Intent(this, EditFriendsActivity.class);
startActivity(intent);
case R.id.action_camera:
Toast.makeText(this, "Asdf", Toast.LENGTH_LONG).show();
AlertDialog.Builder builder = new AlertDialog.Builder(this);
builder.setItems(R.array.camera_choices, mDialogListener);
AlertDialog dialog = builder.create();
dialog.show();
}
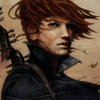
Benjamin Earle
6,204 PointsUpdate
I just noticed that its not only when I go to the Edit Friends screen. The real bug is that when I click on one of the options from the menu, it also, for I dont know what reason, runs the code for the next menu item. For example: If I click the camera, it all runs as it should, because it has no menu item after it. If I click Edit Friends, it runs the code for when I click on the camera icon as well as the code for Edit Friends. And finally, if I click on Log Out, it tries to open the Edit Friends screen AND the camera code while logging out, making the app crash. Please, does someone have an idea of how to fix it?
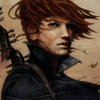
Benjamin Earle
6,204 PointsFor some reason, replacing the switch statement with if and else fixed it... But it works! horray!!

Andre Colares
5,437 PointsHere is the functional code (ANDROID STUDIO 1.4):
protected DialogInterface.OnClickListener mDialogListener =
new DialogInterface.OnClickListener() {
@Override
public void onClick(DialogInterface dialog, int which) {
switch (which){
case 0: //Take Picture
Intent takePhotoIntent = new Intent(MediaStore.ACTION_IMAGE_CAPTURE);
// get path to save images
mMediaUri = getOutputMediaFileUri(MEDIA_TYPE_IMAGE);
if(mMediaUri==null){
//display an error
Toast.makeText(MainActivity.this,
R.string.error_external_storage,
Toast.LENGTH_LONG).show();
}else {
takePhotoIntent.putExtra(MediaStore.EXTRA_OUTPUT, mMediaUri);
startActivityForResult(takePhotoIntent, TAKE_PHOTO_REQUEST);
}
break;
case 1: //Take Video
break;
case 2: //Choose Picture
break;
case 3: //Choose Video
break;
}
}
private Uri getOutputMediaFileUri(int mediaType) {
//To be safe, you should check that the SDCard is mounted
//using Environment.getExternalStorageState() before doing this
if(isExternalStorageAvaible()){
//Get the URI
//1. Get the external storage directory
String appName = MainActivity.this.getString(R.string.app_name);
File mediaStorageDir = new File(
Environment.getExternalStoragePublicDirectory(
Environment.DIRECTORY_PICTURES),
getString(R.string.app_name)
);
// create subdirectory
if ( ! mediaStorageDir.exists() ) {
if ( ! mediaStorageDir.mkdirs() ) {
Log.e(TAG, "Failed to create directory");
return null;
}
}
//3.Create a file name
File mediaFile;
//4. Create the file
Date now = new Date();
String timestamp = new SimpleDateFormat("yyyyMMdd_HHmmss", Locale.US).format(now);
String path = mediaStorageDir.getPath() + File.separator;
switch (mediaType) {
case MEDIA_TYPE_IMAGE:
mediaFile = new File(path + "IMG_" + timestamp + ".jpg");
break;
case MEDIA_TYPE_VIDEO:
mediaFile = new File(path + "VID_" + timestamp + ".mp4");
break;
default:
return null;
}
Log.d(TAG, "File: " + Uri.fromFile(mediaFile));
//5. Return the file's Uri
return Uri.fromFile(mediaFile);
}else {
return null;
}
}
private boolean isExternalStorageAvaible(){
String state = Environment.getExternalStorageState();
return state.equals(Environment.MEDIA_MOUNTED) ? true : false;
}
};