Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial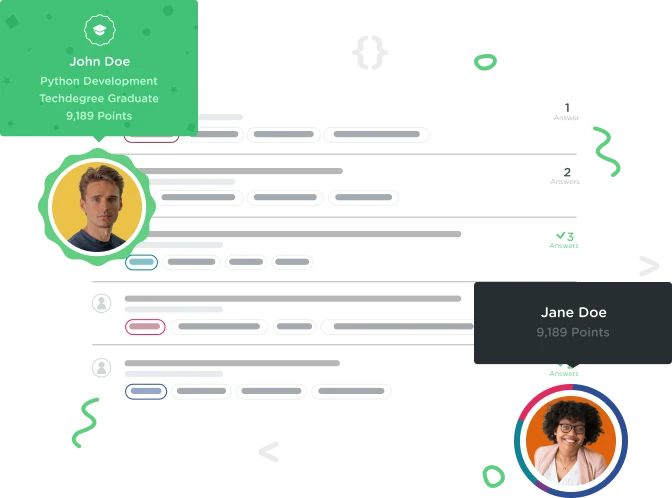
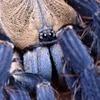
plejsyczejsy
10,348 PointsJust seeking for some advice to improve my code :)
Im working on my skill to keep my code clear and DRY, if someone see any chance here to make something quicker and easier. Please let me know guys :) PS. Is there any chance to do this functions work without: helpful_list, helpful_variable?
def num_teachers(dictionary):
return len(dictionary)
def num_courses(dictionary):
return len(courses(dictionary))
def courses(dictionary):
helpful_list = []
for courses in dictionary.values():
helpful_list.extend(courses)
return helpful_list
def most_courses(dictionary):
helpful_variable = 0
for name, courses in dictionary.items():
if len(courses) > helpful_variable:
helpful_variable = len(courses)
teacher = name
return teacher
def stats(dictionary):
helpful_list = []
for name, courses in dictionary.items():
helpful_list.append([name, len(courses)])
return helpful_list
3 Answers
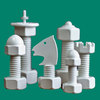
Steven Parker
231,269 PointsGiven what you've studied so far, this code looks very clean and concise. Good job!
And yes, there are ways to do these without creating variables, but those techniques have not been introduced yet. But keep an eye out for list comprehensions in more advanced courses. Here's a "teaser" preview:
def stats(dictionary):
return [[name, len(courses)] for name, courses in dictionary.items()]

Robin Goyal
4,582 PointsIt's great that you're looking for ways to improve your code and it already looks good! I have a few suggestions to make your code slightly more readable and improved in no particular order.
Don't use dictionary as the variable name as it's hard to understand what the contents are. Based on the function names and some of the variables used in the functions, I'm guessing it's a dictionary of teachers and the courses they teach so a better variable name might be
teacher_courses
. There probably would be differing opinions but this is what I would call it.You're right that we might be better off without the helpful_* variables so the best bet is to use better variable names there as well. The courses function is probably a list of all of the courses taught so a better variable name might be
all_courses
. Same for the most_courses function where you could use a variable name likemax_courses
. This one was harder for me to think of one though.For the stats function, the pattern used for creating a list there is a great opportunity to learn about something called list comprehensions. I'll give a basic rundown of list comprehensions. Their syntax is quite simple.
new_list = [<expression> for <vars> in <iterable>]
.
The <expression> are the new elements that you're adding to your new list. <vars> is the variables that are returned from your <iterable>. So we can convert your stats function into a list comprehension. Check out the workshop on list comprehensions to try to convert yours and you could avoid using the helpful_list
variable in your stats function. Here's an example of a list comprehension below though.
>>> # Create a list of squared values (traditional way)
>>> l = [1, 2, 3, 4, 5]
>>> new_l = []
>>> for num in l:
>>> new_l.append(num ** 2)
>>> new_l
[1, 4, 9, 16, 25]
>>>
>>> # Create a list of squared values (list comprehension)
>>> new_l = [num ** 2 for num in l]
>>> new_l
[1, 4, 9, 16, 25]
Lastly, I hope you don't feel discouraged by the different things I once said and everything I have given my opinion on is just a suggestion based on what I have learned. You will learn better practices as you continue on in your programming career. If you have any questions, please ask away! Goodluck :-)!
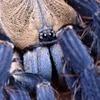
plejsyczejsy
10,348 PointsThank you guys very much! I will keep your advices in my mind.
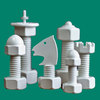
Steven Parker
231,269 Pointsplejsyczejsy — Glad i could help. You can mark a question solved by choosing a "best answer".
And happy coding!