Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial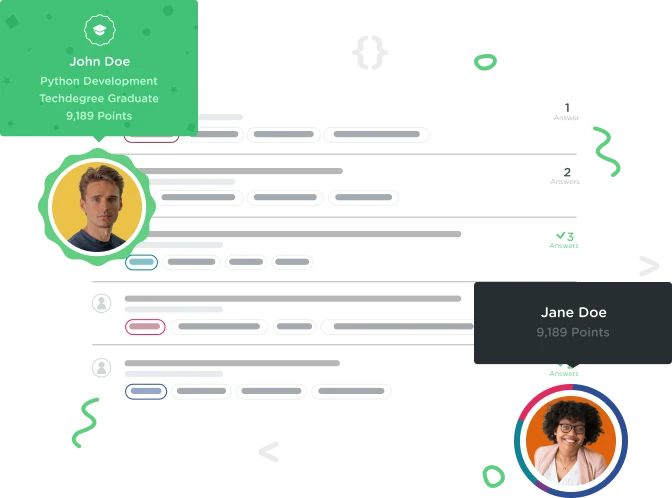

Jeremy Leeper
2,013 PointsJust want to share my work. I'm really shocked that I pulled this off!! https://w.trhou.se/b8dn5gw5qk
I feel that there is definitely another way to display the value between the two requested values, but, I got it to work. I am so proud of myself because I didn't think that I would get it right so quickly. Thanks for this opportunity Treehouse!
1 Answer

Jamison Habermann
11,691 PointsHi there! Great job with your accomplishment! I don't want to discourage you, but I did want to point out a few things in your code.
Your two user prompts are asking for numbers between 0-6 and 3-6, assuming I do actually type in numbers, you still aren't getting numbers you can do math with, you are actually getting strings! Adding two strings together like "1" + "3" will actually give me "13" instead of 4 like you'd expect! This is because string concatenation is happening, instead of math.
Whenever you want to prompt users for numbers, you have to then parse the the prompt with the parseInt (or parseFloat when working with decimals) function like so:
var firstNum = prompt('Give me a number from 0-6.');
firstNum = parseInt(firstNum);
var secNum = prompt('Give me a number from 3-6.');
secNum = parseInt(secNum);
Or you could simplify it to look like this (this is good way to keep your code DRY):
var firstNum = parseInt(prompt('Give me a number from 0-6.'));
var secNum = parseInt(prompt('Give me a number from 3-6.'));
The reason your code appeared to be working before was because you were only working with one of your prompted numbers. You collect the first number, but it isn't present in your equation!
var luckyNum = Math.floor( Math.random() * secNum ) + 4;
If we change the "lucky number generator" equation to the below, it first adds the first prompted number to the second prompted number, then chooses a random number between 0 and the sum of the two numbers, then adds 4. This might create a bit more variety then you may have gotten.
var luckyNum = Math.floor( Math.random() * (firstNum + secNum) ) + 4;
Second thing is I would consider thinking of other ways to name your variables to make them less confusing.
For instance, you have similar naming schemes like firstNum, firstAns, secNum, and secAns. fistNum is actually the first number picked by a user, but someone may confuse that later as the first answer chosen by the user, when in fact you have firstAns as a static, unchanging string.
My suggestion would be to eliminate the firstAns and secAns variables completely by just writing those strings directly into the alerts. Since you don't need to store the strings for any other reason, this is going to be the best way to do this (remember DRY?). I also added some text to the final alert, to show how keeping luckyNum as a variable is useful. When all of these are put together your code should look like this:
var firstNum = prompt('Give me a number from 0-6.');
firstNum = parseInt(firstNum);
alert("Great!");
var secNum = prompt('Give me a number from 3-6.');
secNum = parseInt(secNum);
alert("Awesome! Now let's find out your lucky number of the day!");
var luckyNum = Math.floor( Math.random() * (firstNum + secNum) ) + 4;
alert("Your lucky number is: " + luckyNum);
Jeremy Leeper
2,013 PointsJeremy Leeper
2,013 PointsThank you very much for the feedback! I have a long way to go.