Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial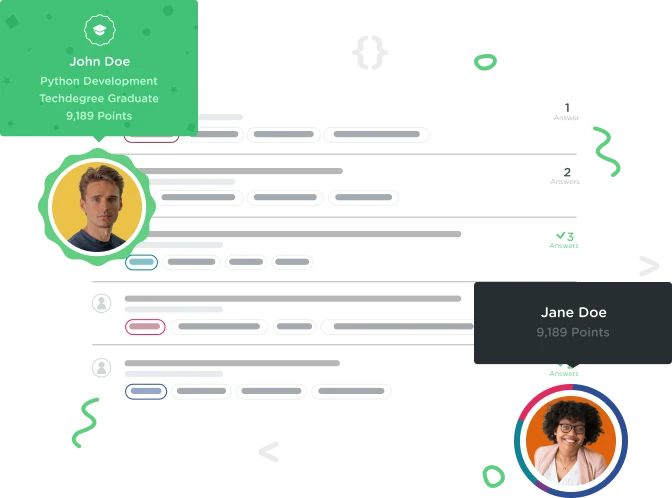

Matt Dobrenen
497 PointsK, now use .format() on the string "Treehouse loves {}" to put your name into the placeholder. Assign this to the variab
K, now use .format() on the string "Treehouse loves {}" to put your name into the placeholder. Assign this to the variable subject (so start with subject =). You do not need to print() anything.
name = 'matt'
subject ='treehouse loves {}' + name.format()
1 Answer
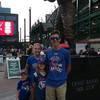
AJ Salmon
5,675 PointsHey Matt! Couple problems here, but they're easy to fix. Firstly, Treehouse challenges are super picky, so you have to do exactly what they say. In this case, you need to make sure 'Treehouse loves {}'
starts with a capital T. Secondly, this challenge requires you to use .format()
on the string "Treehouse loves {}"
, and your name variable should be passed as the argument to .format()
. This should all be assigned to the new variable, subject
. When formatting a string, python will try replacing the curly braces in the string being formatted with whatever argument you pass to it inside the parentheses after .format
. Some examples:
>>> "I have {} dogs".format('3')
'I have 3 dogs'
>>> fav_animal = "chicken"
>>> info = "My favorite animal is a {}".format(fav_animal)
>>> info
'My favorite animal is a chicken'
>>> info2 = "My favorite food is fried {}"
>>> info2.format(fav_animal)
'My favorite food is fried chicken'
Notice in the last example, I didn't actually change the variable info2
into the string 'My favorite food is fried chicken'
. It just showed us what info2
looked like when formatted with fav_animal
. This code would change the string, though:
>>> info2 = "My favorite food is fried {}"
>>> info2 = info2.format(fav_animal)
>>> info2
'My favorite food is fried chicken'
Formatting will become very helpful when writing programs that will display different names or values depending on the conditions. The answer to this challenge will look similar to the second example, about my favorite animal. Hope this helps, and happy coding!