Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial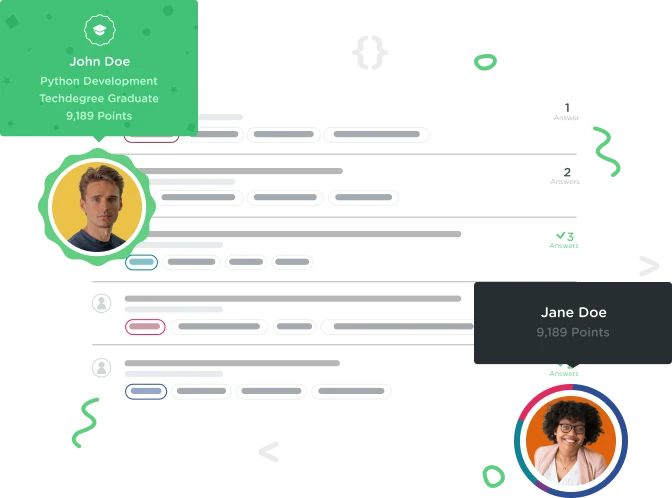

Evan Waterfield
3,240 PointsKeep getting a NoneType error for if answer[0]
import datetime
import random
from questions import Add, Multiply
class Quiz:
questions = []
answers = []
def __init__(self):
question_types = (Add, Multiply)
# generate 10 random questions with numbers from 1 to 10
for _ in range(10):
num1 = random.randint(1,10)
num2 = random.randint(1,10)
question = random.choice(question_types)(num1, num2)
# add these questions to self.questions
self.questions.append(question)
def take_quiz(self):
# log the start time
self.start_time = datetime.datetime.now()
# ask all of the questions
for question in self.questions:
self.answers.append(self.ask(question))
else:
self.end_time = datetime.datetime.now()
# log if they got the questions right
# log the end time
# show a summary
return self.summary()
def ask(self, question):
correct = False
# log the start time
question_start = datetime.datetime.now()
# capture the answer
answer = input(question.text + ' = ')
# check the answer
if answer == str(question.answer):
correct = True
# log the end time
question_end = datetime.datetime.now()
# if the answer is right, send back true
# otherwise, send back false
# send the elasped time, too
def total_correct(self):
# return the total # of correct answers
total = 0
for answer in self.answers:
if answer[0]:
total += 1
return total
def summary(self):
# print how many you got right and the total # of questions. 5/10
print("You got {} out of {} right.".format(
self.total_correct(), len(self.questions)
))
# print the total time for the quiz: 30 seconds!
print("It took you {} seconds total.".format(
(self.end_time-self.start_time).seconds
))
Quiz().take_quiz()
1 Answer

aaronbuckles
3,291 PointsSorry that this is so late, but for people who may see this in the future, the problem is in the ask method. You never returned the answer or elapsed time. At the bottom of the ask method add this
elapsed_time = question_end - question_start
return correct, elapsed_time
Quinton Dobbs
5,149 PointsQuinton Dobbs
5,149 PointsHelped me out.