Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial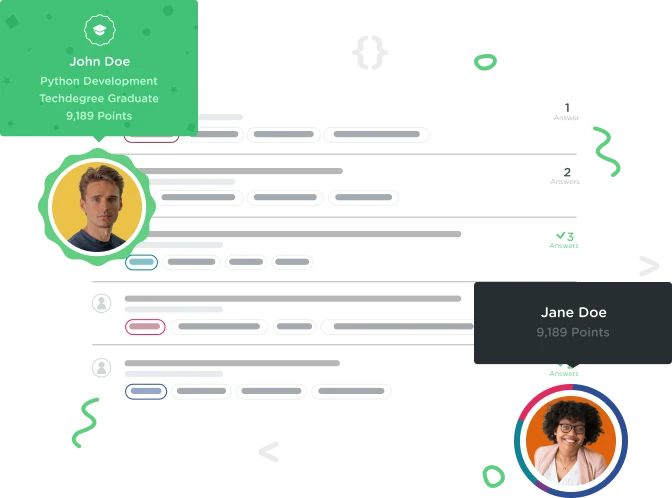

Ra Bha
2,201 PointsKeep getting an error despite my code reflecting the Code Challenge instructions. Not sure what to do here
I feel like my code for this challenge reflects the instructions including a Square subclass of class Polygon. However, I keep getting a Bummer: did you define your Square class...error. I am not sure how to fix this.
namespace Treehouse.CodeChallenges
{
class Polygon
{
public readonly int NumSides;
{
}
public Polygon(int numSides)
{
NumSides = numSides;
}
}
class Square : Polygon
{
public readonly int SideLength;
{
public Square(int sideLength) : base NumSides(4);
{
}
}
}
}
2 Answers
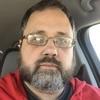
Mark Sebeck
Treehouse Moderator 38,304 PointsHi Ra Bha. You're close but I see a few issues. SideLength is a field so remove the brackets after "public readonly int SideLength; "
Also the format for base is just : base(parameter to pass) so here it should be
public Square(int sideLength) : base (4)
instead of
public Square(int sideLength) : base NumSides(4);
Also don't forget to initialize the SideLength field with the value in the constructor. So you will need something like they have in the Polygon class but with SideLength and sideLength.
Keep at it Ra Bha. Inheritance is not an easy concept. Post your updated code if you are still having issues.

Ra Bha
2,201 PointsThank you Mark, this guidance helped me and I resolved the issue.
A couple followup questions just to make sure my understanding of the logic matches the level of Code Challenge completion:
1) I noticed that the statement with the base keyword does not have a semicolon at the end. Would it be correct to think of that as the rule for using base within code? If not, in which circumstances would you not use a semicolon at the end?
2) I understand now that the base keyword passes the parameters from the subclass to the constructor of the class. I realize that the field for the subclass must be initialized, aka
SideLength = sideLength;
But if the base keyword passes the parameter 4 to the constructor of the parent class Polygon, then why isn't code required to initialize NumSides aka
NumSides = numSides;
?
Thanks, Mark - I really appreciate it.
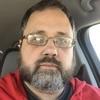
Mark Sebeck
Treehouse Moderator 38,304 PointsYou are welcome Ra Bha. Always happy to help. So glad you were able to resolve your issue.
There is no semicolon after base because it is a constructor definition. Just like there is no semicolon after Polygon is declared. Just a bracket
public Polygon(int numSides)
{
NumSides = numSides;
}
NumSides is being set in the Polygon constructor like you said. That is the advantage of inheritance. Think of it as calling the parent constructor and passing in 4. NumSides will be set to 4 in the Polygon class. I hope that's clear. You kind of almost answered your second question in your question.