Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial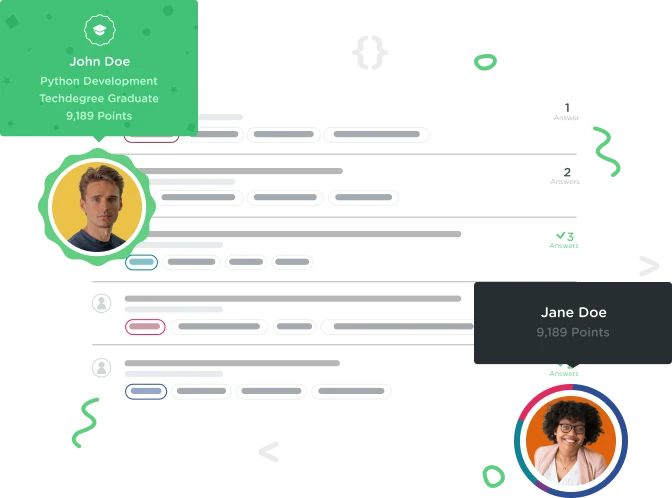

Schott Taylor
Courses Plus Student 7,125 PointsKeep getting an error when i check work on this? What is wrong?
Question Details are very detailed
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double) -> Double {
return width += points
height += points
}
}
class RoundButton: Button {
var cornerRadius: Double = 5.0
override func incrementBy(_ points: 7.0) -> Double {
return width += points
height += points
}
}
2 Answers
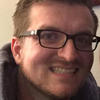
Michael Bates
13,344 PointsHi there!! There are several issues you have going on at a glance. I had trouble with this one not too long ago, so I will help out as best as I can.
The major issue I had was in the func statement. I had the exact same line of syntax in the function statement like you have. It turns out that you do not need the -> Double. The same thing applies to the override func statement.
Next, you don't need to declare return statements. It assumed that it is returned, if I remember correctly.
Lastly, you need to declare the override func statement before the var cornerRadius statement.
Give this code a go:
class Button {
var width: Double
var height: Double
init(width:Double, height:Double){
self.width = width
self.height = height
}
func incrementBy(points: Double){
width += points
height += points
}
}
class RoundButton: Button {
override func incrementBy(_ points: Double = 7.0) {
}
var cornerRadius: Double = 5.0
}
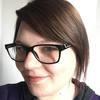
Emily Kolar
2,787 PointsActually, you'll want to keep your return statements. I don't really know what language the person above might be thinking of, perhaps a variant, but with regular Swift, you'll want to be fairly explicit with returns.
However, keep in mind that the functions will exit immediately upon those return statements, and all code underneath your return lines will not be executed. Return literally means "return from this function now", so you have to return as the very last step.
If your intention there is to return two values, you'll have to make it an object, an array, or something else with multiple pieces allowed. (A double is just one solitary decimal number)