Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial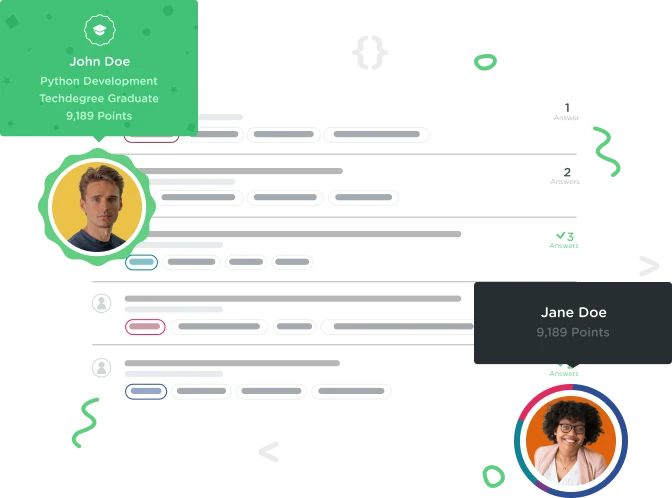

anthonyparker2
4,854 PointsKeep getting code could not compile. Code works fine on my XCode however.
Preview is blank so I can't even see the compiler errors.
class Point {
var x: Int
var y: Int
init(x: Int, y: Int) {
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! I'm a machine!")
}
}
// Enter your code below
class Robot: Machine {
override init(){
super.init()
}
override func move(direction: String) {
switch direction {
case "Up":
self.location.y += 1
case "Down":
self.location.y -= 1
case "Right":
self.location.x += 1
case "Left":
self.location.x -= 1
default: break
}
}
}
2 Answers
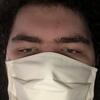
Zack Mowrer
Full Stack JavaScript Techdegree Graduate 62,350 PointsDo 4 Things here to pass this challenge. First, in the move method in the Machine class, put a backslash in the string "Do nothing! I'm a machine!", before the apostrophe in "I'm".
Then, in the method move() for both Machine and Robot, make it so that move() has no external name for the parameter, but has a local name of direction for the parameter. (replace func move(direction: String) with func move(_ direction: String in both classes.)
Next, in the switch statement in the Robot class, you don't have to use self to refer to the location variable. You can substitute self.location.y with just location.y and self.location.x with just location.x (because of inheritance)
Finally, overriding the initializer is completely unnecessary, as you aren't initializing any new values in the subclass. You can just remove that bit of code.
I hope this gets you through the challenge.
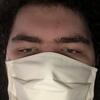
Zack Mowrer
Full Stack JavaScript Techdegree Graduate 62,350 PointsNo Problem. I'm glad I could help.
anthonyparker2
4,854 Pointsanthonyparker2
4,854 PointsThanks very much! I didn't think I needed the override, just saw it in an example that worked.
Thanks again!