Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial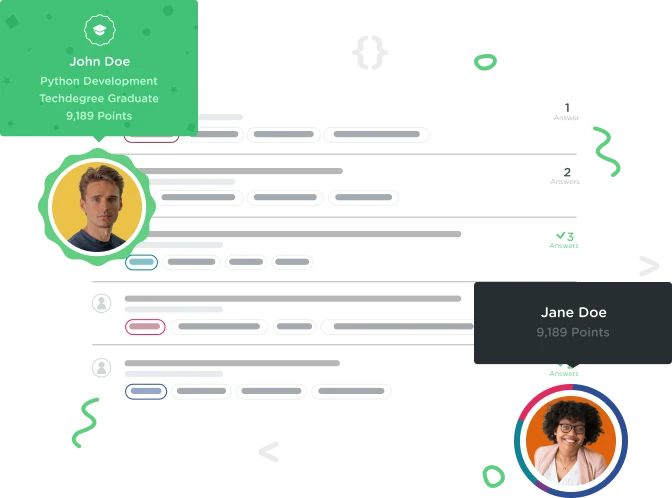

Rafael Singer
Courses Plus Student 13,161 PointsKeep getting error message "Cannot read property '1' of null"
Trying to assign variable listItems to the HTML collection of all list items containing the tag <u1>
// Complete the challenge by writing JavaScript below
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
let listItems = document.getElementsByTagName("ul");
for ( let i = 0; i < colors.length; i++ ) {
listItems[i].style.color = colors[i];
}
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="utf-8">
<title>JavaScript and the DOM</title>
</head>
<body>
<h1>My List</h1>
<ul>
<li>This should be red</li>
<li>This should be orange</li>
<li>This should be yellow</li>
<li>This should be green</li>
<li>This should be blue</li>
<li>This should be indigo</li>
<li>This should be violet</li>
</ul>
<script src="app.js"></script>
</body>
</html>
1 Answer

martinjones1
Front End Web Development Techdegree Graduate 44,824 PointsVery close, but the main reason for this error, is that you are only selecting the UL element.
For the UL element, there is only one of them on the page, if you click the preview/refresh button you will see that all of the list items turn red, the first color in the colors array [0]. When it loops over to the colors array to apply the next color, it will not work since it does not exist.
listItems[1].style.color = colors[1];
Instead you need to be selecting the LI elements as below:
// Complete the challenge by writing JavaScript below
const colors = ["#C2272D", "#F8931F", "#FFFF01", "#009245", "#0193D9", "#0C04ED", "#612F90"];
let listItems = document.getElementsByTagName('li');
for ( let i = 0; i < colors.length; i++ ) {
listItems[i].style.color = colors[i];
}