Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial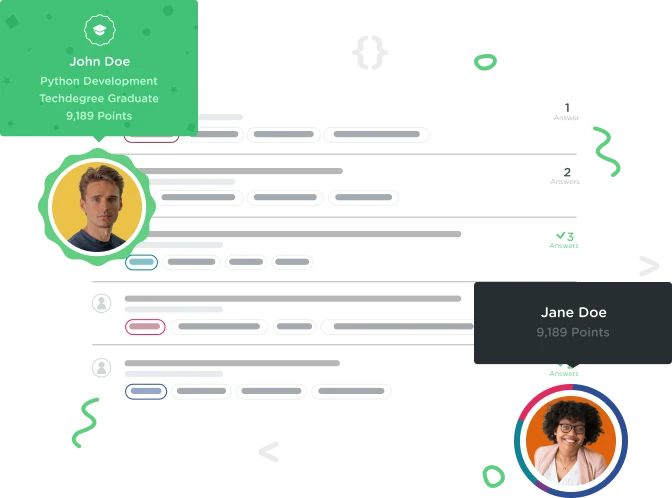

Greg Cruz
1,240 PointsKeep getting "java.util.NoSuchElementException (Look around Scanner.java line 862)" whenever I try and use the scanner
So, as far as I can tell, this code should be running. However, when I use the prompt "scanner.next();" it brings up the title error. I don't understand what's wrong. Can someone please shed some light on this?
FYI, the actual Error reads: "java.util.NoSuchElementException at java.util.Scanner.throwFor(Scanner.java:862) at java.util.Scanner.next(Scanner.java:1371) at ConferenceRegistrationAssistant.getLineNumberFor(ConferenceRegistrationAssistant.java:13) at JavaTester.run(JavaTester.java:91) at JavaTester.main(JavaTester.java:43)"
import java.util.Scanner;
public class ConferenceRegistrationAssistant {
/**
* Assists in guiding people to the proper line based on their last name.
*
* @param lastName The person's last name
* @return The line number based on the first letter of lastName
*/
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
Scanner scanner = new Scanner(System.in);
System.out.print("Please enter your last name. ");
lastName = scanner.next();
if (lastName.charAt(0) < 'N');{
lineNumber = 1;
}
if (lastName.charAt(0) >= 'N');{
lineNumber = 2;
}
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
}
public class Example {
public static void main(String[] args) {
/*
IMPORTANT: You can compare characters using <, >. <=, >= and == just like numbers
*/
if ('C' < 'D') {
System.out.println("C comes before D");
}
if ('B' > 'A') {
System.out.println("B comes after A");
}
if ('E' >= 'E') {
System.out.println("E is equal to or comes after E");
}
// This code is here for demonstration purposes only...
ConferenceRegistrationAssistant assistant = new ConferenceRegistrationAssistant();
/*
Remember that there are 2 lines.
Line #1 is for A-M
Line #2 is for N-Z
*/
int lineNumber = 0;
/*
This should set lineNumber to 2 because
The last name is Zimmerman which starts with a Z.
Therefore it is between N-Z
*/
lineNumber = assistant.getLineNumberFor("Zimmerman");
/*
This method call should set lineNumber to 1, because 'A' from "Anderson" is between A-M.
*/
lineNumber = assistant.getLineNumberFor("Anderson");
/*
Likewise Charlie Brown's 'B' is between 'A' and 'M', so lineNumber should be set to 1
*/
lineNumber = assistant.getLineNumberFor("Brown");
}
}
1 Answer
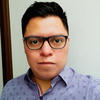
Jerson Otzoy
1,532 PointsHello,
You don't need to use scanner in your method since the values to use are in the Example.java file. And you have semicolons after the if verification, remove them too since those are not needed there. This will fix it.
public int getLineNumberFor(String lastName) {
int lineNumber = 0;
if (lastName.charAt(0) < 'N') {
lineNumber = 1;
}
if (lastName.charAt(0) >= 'N') {
lineNumber = 2;
}
/*
lineNumber should be set based on the first character of the person's last name
Line 1 - A thru M
Line 2 - N thru Z
*/
return lineNumber;
}
Greg Cruz
1,240 PointsGreg Cruz
1,240 PointsHuh. I actually tried that before I started messing with the scanner... I must have had a syntax error or something. In any case, thank you!