Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial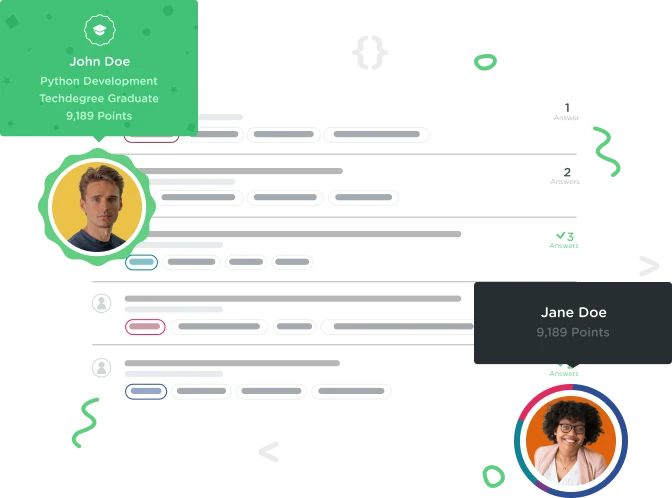
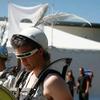
Connor Acott
7,572 PointsKind of a nit-picky question, but I was curious about best practices with variables.
Something I've been wondering for a little now while during JavaScript courses, and I'd appreciate if anyone wouldn't mind clearing this up.
I solved step 3 (the "for" loop) like this:
for ( let i = 0; i < quiz.length; i++ ) {
let guess = prompt(quiz[i][0]);
if ( guess.toLowerCase() === quiz[i][1].toLowerCase() ) {
totalCorrect++;
}
}
When watching Guil's solution video however, I noticed that he declared variables for question
and answer
within his loop first. If I had done the same, my code would have looked like this instead:
for ( let i = 0; i < quiz.length; i++ ) {
let question = quiz[i][0];
let answer = quiz[i][1];
let guess = prompt(question);
if ( guess.toLowerCase() === answer.toLowerCase() ) {
totalCorrect++;
}
}
Is this just a personal preference, or maybe something that Guil is doing to make it easier to follow? Or is it the kind of thing that I should get in the habit of doing too? Even though my code ran fine, I could totally see this being the kind of thing that's encouraged in the professional world to make your code more readable.
If it doesn't matter then I'll stick to doing things the way that's most intuitive for me, but I just want to make sure that I'm not forming bad habits. I've noticed a lot of variables popping up in class that don't seem strictly necessary, so I've been wondering if I missed something about the "right time" to declare a variable. Thank you!
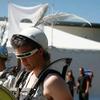
Connor Acott
7,572 PointsAwesome, thanks Ashish Mehra ! Makes sense that adding the variables in this case could help readability, but also wouldn't be strictly necessary. Glad to hear I didn't miss anything after all. I think I keep expecting there to be more "rights" and "wrongs" than there actually are when writing code, and it's nice to see that it's generally more open-ended than that. Appreciate the example of a third option too!
1 Answer
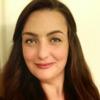
Jennifer Nordell
Treehouse TeacherHi there, Connor Acott! I could be wrong, but I believe you hit the nail on the head when you wrote "make it easier to follow". I have a strong feeling that that's exactly why Guil chose that. I could be wrong, of course.
There are some cases where readability comes into play, but I don't think your solution is unreadable at all, personally. Just my two cents.
Hope this helps!
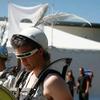
Connor Acott
7,572 PointsCool, thanks Jennifer Nordell ! Makes sense to me. I feel like I might be expecting there to be more "right" or "wrong" answers than there actually are when writing code, when maybe it's more like "readable = good, confusing = bad, end of story." Glad to hear I wasn't missing something!
Ashish Mehra
2,408 PointsAshish Mehra
2,408 PointsHi Connor Acott,
Everyone have different approaches for solving a problem.
The way you approached the solution is also fine for this case because you're not using
quiz[i][0]
andquiz[i][1]
in multiple places.The code solution of Guil have few things I like to mention
The variable question and answer help other developer to understand code more fast because they will be able to identify which one index is question and which one is answer.
If code require more use of question and answer instead of using
quiz[i][0]
orquiz[i][1]
one can simply use variablequestion
andanswer
.My approach for this code will be:
question
andanswer
Hope it helps,
Thanks