Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial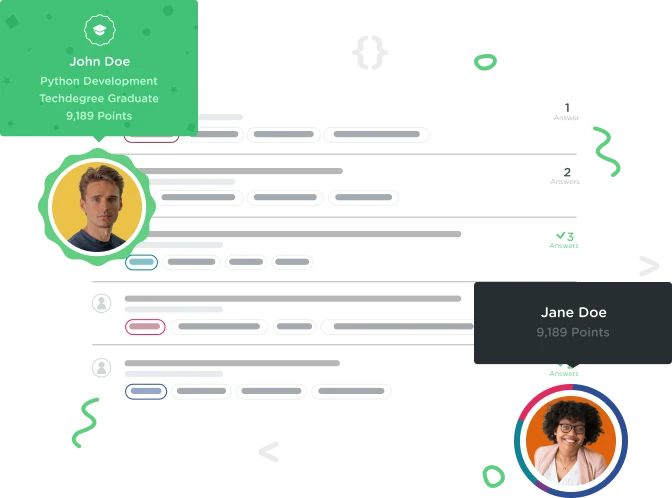
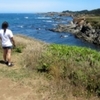
Nancy Melucci
Courses Plus Student 36,143 PointsLambda takes 1 positional argument, reduce takes 2
I feel like I understand lambda but I am not able to make it play nice with reduce in this challenge. I am trying to make the lambda argument 1 and the list lambda 2 and I've tried about a dozen re-arrangements of the parens with no luck. What am I missing? Or am I giving myself too much credit here : (
I love these tutorials but I honestly wish the feedback were more detailed. Thanks in advance.
longest = reduce((lambda string: [len(string) > len(string) for string in strings]), strings)
from functools import reduce
strings = [
"Do not take life too seriously. You will never get out of it alive.",
"My fake plants died because I did not pretend to water them.",
"A day without sunshine is like, you know, night.",
"Get your facts first, then you can distort them as you please.",
"My grandmother started walking five miles a day when she was sixty. She's ninety-seven know and we don't know where she is.",
"Life is hard. After all, it kills you.",
"All my life, I always wanted to be somebody. Now I see that I should have been more specific.",
"Everyone's like, 'overnight sensation.' It's not overnight. It's years of hard work.",
]
3 Answers
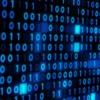
Alexander Davison
65,469 PointsYou are writing a lambda correctly, but the reduce
function is expecting you to be able to take in two arguments instead of one.
Here's a use of reduce
with lambda:
from functools import reduce
print(reduce(lambda x, y: x + y, [1, 2, 3, 4, 5]))
The output will print 15 (which is 1+2+3+4+5).
Here's how the program above will work:
# First, the output list will be:
[1, 2, 3, 4, 5]
# Next, the program takes the first two elements and calls our lambda function against those two (which will add them):
[3, 2, 3, 4, 5]
# It will repeat the same action again:
[5, 3, 4, 5]
# And again...
[9, 4, 5]
# And again...
[13, 5]
# Then the answer is created.
18
As you see, the reduce
function boils down a list into one value by calling a function against the first two elements, then replacing the first two elements with that result.
Here's a way to do it without using FP (Functional Python):
strings = [
"Do not take life too seriously. You will never get out of it alive.",
"My fake plants died because I did not pretend to water them.",
"A day without sunshine is like, you know, night.",
"Get your facts first, then you can distort them as you please.",
"My grandmother started walking five miles a day when she was sixty. She's ninety-seven know and we don't know where she is.",
"Life is hard. After all, it kills you.",
"All my life, I always wanted to be somebody. Now I see that I should have been more specific.",
"Everyone's like, 'overnight sensation.' It's not overnight. It's years of hard work.",
]
longest = ''
for string in strings:
if len(string) > len(longest):
longest = string
I hope these hints help you out
Good luck! ~Alex
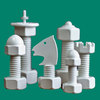
Steven Parker
231,268 Points
The function used by reduce must take two arguments.
In this case, the function is your lambda, which you have defined as: "lambda string: ....
". But a lambda used for reduce would need to take two arguments, something like this: "lambda s1, s2:
".
Then, in the body of the lambda you need to perform a process that will use both arguments and return a single result. You won't need a list comprehension here because reduce takes care of calling your function repeatedly with new pairs of arguments until the source list is exhausted.
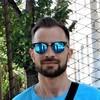
Oszkár Fehér
Treehouse Project ReviewerThis tip helped me also, unbelievable how easy is when you realize how you have to make it, i was trying with list comprehension too much. thanks Steven
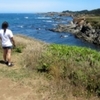
Nancy Melucci
Courses Plus Student 36,143 PointsI didn't realize that the lambda can take an if statement as an argument. Once I got that I was able to pass the challenge. Thanks!