Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial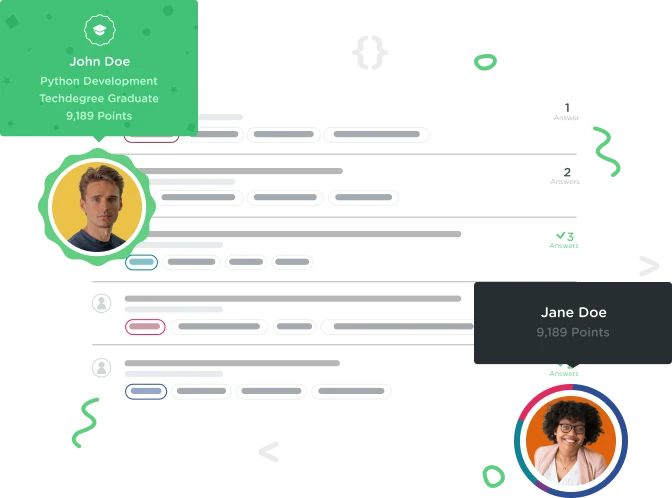

Josh Keenan
19,652 PointsLaptop booking system (tkinter)
Hey Pythonistas, I'm developing a laptop booking system for my college (whodathunkit?!) in Python using tkinter and sqlite. I have hit a problem, I'm using a frame and I can't make it visible (columns and rows need to be visible!) and all my research into it is having no help as I can't find any useful information (incorrect information is everywhere!).
Basically I want to have a table that's empty! I can't make the lines in between the rows and columns visible!
Edit: tagging Chris Freeman for help

Josh Keenan
19,652 Pointsimport tkinter
from tkinter import messagebox
class Body:
def __init__(self, master):
self.rows = 7
self.columns = 5
self.master = master
self.mainframe = tkinter.Frame(self.master, bg="white") # building the grid
self.mainframe.pack(fill=tkinter.BOTH, expand=True) # packing the grid for use
self.build_grid() # calling the config function
self.build_banners()
def build_grid(self): # method for configuring the grid,
self.mainframe.columnconfigure(0, weight=1) # setting up amount of rows and columns
self.mainframe.columnconfigure(1, weight=1)
self.mainframe.columnconfigure(2, weight=1)
self.mainframe.columnconfigure(3, weight=1)
self.mainframe.columnconfigure(4, weight=1)
self.mainframe.columnconfigure(5, weight=1)
self.mainframe.columnconfigure(6, weight=1)
self.mainframe.rowconfigure(0, weight=1)
self.mainframe.rowconfigure(1, weight=1)
self.mainframe.rowconfigure(2, weight=1)
self.mainframe.rowconfigure(3, weight=1)
self.mainframe.rowconfigure(4, weight=1)
# ignore this method
def build_banner(self):
banner = tkinter.Label(
self.mainframe,
bg="red",
text="{}{}".format(self.row, self.column),
fg="white",
font=("Helvetica", 24)
)
banner.grid(
row=0,column=0,
sticky="ew",
padx=10, pady=10
)
banner.pack(fill=tkinter.X)
# problem method
def build_banners(self):
self.row = 0
self.column = 0
self.count = 0
for row in range (0, self.columns * 7):
banner = tkinter.Label(
self.mainframe,
bg="red",
text="Laptop booking",
fg="white",
font=("Helvetica", 24)
)
banner.grid(
row=self.row,column=self.column,
sticky="ew",
padx=5, pady=5
)
banner.pack(fill=tkinter.BOTH, expand=True)
print (self.count)
self.count+=1
self.row+=1
if self.count % 7 == 0:
self.count = 0
self.row = 0
self.column += 1
if __name__ == "__main__":
root = tkinter.Tk()
Body(root)
root.mainloop()
Problem with the grid method in the build_banners method. Trying to put one of the same banner in each position in the grid
3 Answers
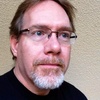
Chris Freeman
Treehouse Moderator 68,423 PointsJosh, Sorry for the delayed response. I needed to go learn tkinter
.
I'm not sure what you are trying to do with the build_banners
method. Can you describe what the end goal is?
It seems that you might be overcomplicating your row and column values by using attributes.
I hacked your code to verify I can populate all grid positions:
class Body:
def __init__(self, master):
self.rows = 7
self.columns = 5
self.master = master
self.mainframe = tkinter.Frame(
self.master, bg="white") # building the grid
# packing the grid for use
self.mainframe.pack(fill=tkinter.BOTH, expand=True)
# calling the config function
self.build_grid()
# self.build_banners() # <-- Comment Out call to self.build_banners
# Added mock fill method
def mock_fill(self, row, col, text):
temp = tkinter.Label(
self.mainframe,
bg='grey',
text=text,
fg='black',
font=('Helvetica', 10)
)
temp.grid(
row=row, column=col,
sticky='ew', # East West
padx=10, pady=10
)
# method for configuring the grid,
def build_grid(self):
# setting up amount of rows and columns
self.mainframe.columnconfigure(0, weight=1)
self.mainframe.columnconfigure(1, weight=1)
self.mainframe.columnconfigure(2, weight=1)
self.mainframe.columnconfigure(3, weight=1)
self.mainframe.columnconfigure(4, weight=1)
self.mainframe.columnconfigure(5, weight=1)
self.mainframe.columnconfigure(6, weight=1)
self.mainframe.rowconfigure(0, weight=1)
self.mainframe.rowconfigure(1, weight=1)
self.mainframe.rowconfigure(2, weight=1)
self.mainframe.rowconfigure(3, weight=1)
self.mainframe.rowconfigure(4, weight=1)
# Added loops to fill all positions
for col in range(7):
for row in range(5):
self.mock_fill(row, col, "r{}c{}".format(row, col))
Output
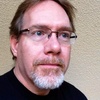
Chris Freeman
Treehouse Moderator 68,423 PointsInspired by this StackOverflow answer, you could think of the picture above as having very wide white
grid lines. If I were to set the background to black
then use thinner padding, it might create the look you want.

Josh Keenan
19,652 PointsHey Chris, Thanks a lot, essentially I plan to create a 5 x 7 grid filled with buttons.
Each button will link to a specific time on a specific day and the user will be able to book laptops (up to 30) , for one hour. They simply collect them at the appropriate time and return them one hour later, considering adding an alert/timer system to the laptops in question to let users know when to return them.
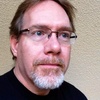
Chris Freeman
Treehouse Moderator 68,423 PointsWere you able to work out the issue with addressing the various grid positions?

Josh Keenan
19,652 PointsHey Chris, thanks that worked great, now just converting them all to buttons and that will be the real challenge!
Chris Freeman
Treehouse Moderator 68,423 PointsChris Freeman
Treehouse Moderator 68,423 PointsCan you post some code snippets or a github link?