Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial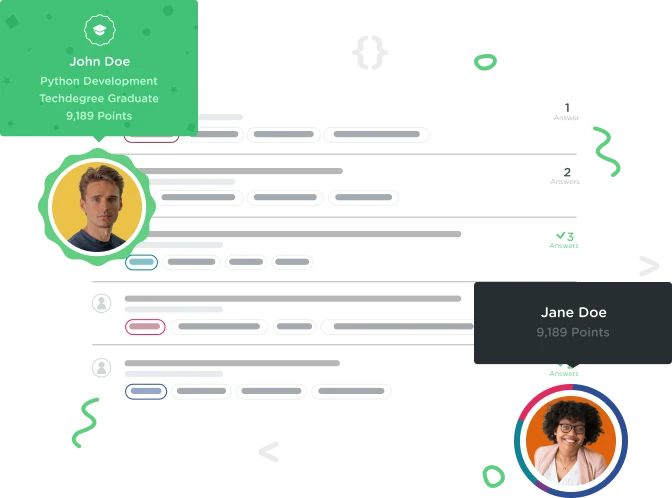
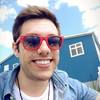
hiroshi
13,701 PointsLast challenge in Ruby Booleans is not working
This challenge is supposed to have you return true or false for the contains? method if the todo_items array contains an element containing the passed in argument. My solution works in irb, but the challenge tells me that the method does not return a boolean value
def contains?(name)
todo_items.include?(name)
end
should return true or false but I get the above error. To prove I wasn't crazy, I also tried:
def contains?(name)
true
end
and it also tells me it does not return a boolean value which is the only thing the method does.
class TodoList
attr_reader :name, :todo_items
def initialize(name)
@name = name
@todo_items = []
end
def add_item(name)
todo_items.push(TodoItem.new(name))
end
def contains?(name)
true
end
def find_index(name)
index = 0
found = false
todo_items.each do |item|
found = true if item.name == name
break if found
index += 1
end
if found
return index
else
return nil
end
end
end
1 Answer
William Li
Courses Plus Student 26,868 PointsHi, Hiroshi, Because todo_items is just an Array containing TodoItem objects, you need to look at the name attribute of each TodoItem object to find out if todo_items include the one you looking for.
if the todo items array contains an item with the name argument.
That's why this code todo_items.include?(name)
doesn't work.
Also, in this code
def contains?(name)
true
end
it also tells me it does not return a boolean value, well, yeah, here I think the grader can be a bit more helpful. It should probably tells you that the code returns a wrong boolean value.
Here's one way to do it
def contains?(name)
todo_items.each do |item|
return true if item.name==name
end
false
end
Or .
def contains?(name)
todo_items.any? {|item| item.name==name}
end
hiroshi
13,701 Pointshiroshi
13,701 PointsOOOOOOHHHHHHHH facepalm I forgot the array was TodoItems and not just strings. Derp.
I got hooked on the error message which didn't seem to fit
Thanks!