Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial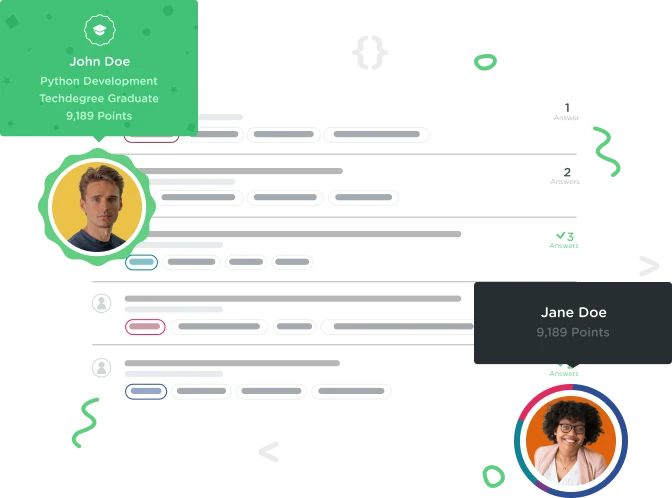

Vic Mercier
3,276 PointsLast child and first child (look in the video dom last child and first child for a better understanding of the challeng)
For example, notice when I click on the Up button of the top list item, nothing happens, because they can't go up any further than the top, right? So using first element, child, you could remove the Up button here. So the app doesn't have a nonfunctional button.
I don't know how to do that.
const removeItemInput = document.querySelector("input.removeInput");
const toggleList = document.getElementById("togglelist");
const listDiv = document.querySelector(".list");
const listDivUl = document.querySelector("ul");
const myInput = document.querySelector("input.description");
const myP = document.querySelector("p.description");
const button = document.querySelector("button.description");
const addItemInput = document.querySelector("input.addItemInput");
const addItemButton = document.querySelector("button.addItemButton");
const removeButton = document.querySelector("button.removeItemButton");
const lis = listDivUl.children;
function attachListItemsButton(li){
//Up button
let up = document.createElement("button");
up.className = "up";
up.textContent = "up";
li.appendChild(up);
//Down button
let down = document.createElement("button");
down.className = "down";
down.textContent = "down";
li.appendChild(down);
//Remove button
let remove = document.createElement("button");
remove.className = "remove";
remove.textContent = "remove";
li.appendChild(remove);
}
for(let i = 0; i < lis.length; i += 1){
attachListItemsButton(lis[i]);
}
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON"&& event.target.className == "remove"){
let li = event.target.parentNode;
let ul = li.parentNode;
ul.removeChild(li);
}
});
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON" && event.target.className == "up"){
let li = event.target.parentNode;
let previousLi = li.previousElementSibling;
let ul = li.parentNode;
if(previousLi){
ul.insertBefore(li, previousLi);
}
}
});
listDivUl.addEventListener("click", (event) => {
if(event.target.tagName == "BUTTON" && event.target.className == "down"){
let li = event.target.parentNode;
let nextLi = li.nextElementSibling;
let ul = li.parentNode;
if(nextLi){
ul.insertBefore(nextLi, li);
}
}
});
toggleList.addEventListener("click", () =>{
if(listDiv.style.display == "none"){
toggleList.textContent = "Hide list";
listDiv.style.display = "block";
}else{
toggleList.textContent = "Show list";
listDiv.style.display = "none";
}
});
button.addEventListener("click", () => {
myP.textContent = myInput.value+" : " ;
myInput.value = "";
});
addItemButton.addEventListener("click", () => {
let values = [];
let ul = document.getElementsByTagName("ul")[0];
let li = document.createElement("li");
li.textContent = addItemInput.value;
attachListItemsButton(li);
ul.appendChild(li);
addItemInput.value = "";
});
<!DOCTYPE html>
<html>
<head>
<title>List creator</title>
<link rel="stylesheet" href="css/style.css">
</head>
<body>
<h1 id="myHeading">List creator</h1>
<p>Making a web page interactive</p>
<button id = "togglelist">Hide list</button>
<div class = "list">
<p class = "description">Things that are purple:</p>
<button class = "description">Change list description</button>
<input type = "text" class = "description">
<ul>
<li>Orange</li>
<li>Grapes</li>
</ul>
<input type = "text" class ="addItemInput">
<button class = "addItemButton">Add something</button>
<button class = "removeItemButton">Remove an item of the list:</button>
</div>
<script src="app.js"></script>
</body>
</html>
1 Answer
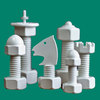
Steven Parker
230,274 PointsYou might want to review the Removing Nodes video.
That video shows you how to remove a node (such as the button) from the page. But be aware that if you remove the "up" button from the top item (and I assume the "down" button from the bottom), you'll also need to recreate them if those items are moved using the remaining button. Plus, you'll also need to restore them if another item is moved up to the top position (or down to the bottom). So to do a complete implementation will require quite a bit of new code.
As an alternative program enhancement, I would recommend just setting the button's "disabled" property to true, which will change the visual appearance in a way most users will recognize as meaning "not active". Then you can just clear that property (by setting it to false) when the item is moved, or another item is moved to the edge position.

Vic Mercier
3,276 PointsWhat is the remaining button

Vic Mercier
3,276 PointsIs there a way to check if the buttons have been moved?
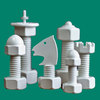
Steven Parker
230,274 PointsIf you remove the "up" button, the remaining button will be "down".
You could store the original position to be able to tell if an item was moved, but it might be easier to take care of any move-related issues when the movement is actually done in the handler.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsThis sounds a lot like your previous question.