Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial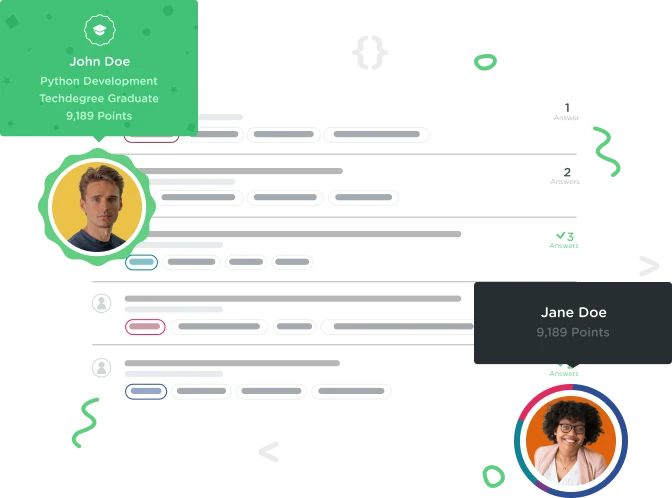
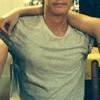
William Hartvedt Skogstad
5,857 PointsLast Enumeration Task Swift 2.0
Challenge Task 2 of 2
To the button enum, add a method named toUIBarButtonItem that returns an instance of UIBarButton item configured properly.
In the buttons.swift file there is a basic implementation of UIBarButtonItem. You can create buttons with three different styles and titles.
Using the associated values as titles for the button, return a button with style UIBarButtonStyle.Done for the Done member of the Button enum. Similarly for the Edit member, return a UIBarButtonItem instance with the style set to UIBarButtonStyle.Plain.
In both cases you can pass nil for target and action. Once you have a method, call it on the value we created in the previous task and assign it to a constant named doneButton.
I really can't grasp what they want me to do, task 1 was piece of cake, but this is way too much for me to handle.. (I'm paying 25$ a month for this, make the tasks more easy on the beginners please...)
import Foundation
enum UIBarButtonStyle {
case Done
case Plain
case Bordered
}
class UIBarButtonItem {
var title: String?
let style: UIBarButtonStyle
var target: AnyObject?
var action: Selector
init(title: String?, style: UIBarButtonStyle, target: AnyObject?, action: Selector) {
self.title = title
self.style = style
self.target = target
self.action = action
}
}
enum Button {
case Done(String)
case Edit(String)
func toUIBarButtonItem() -> UIBarButton {
return UIBarButtonStyle.Done
}
}
let done = Button.Done("Done")
1 Answer
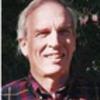
jcorum
71,830 PointsHere you go:
enum Button {
case Done(String)
case Edit(String)
case Bordered(String)
func toUIBarButtonItem() -> UIBarButtonItem {
switch self {
case .Done(let doneValue):
return UIBarButtonItem(title: doneValue, style: UIBarButtonStyle.Done, target: nil, action: nil)
case .Edit(let editValue):
return UIBarButtonItem(title: editValue, style: UIBarButtonStyle.Plain, target: nil, action: nil)
case .Bordered(let borderValue): //not in challenge
return UIBarButtonItem(title: borderValue, style: UIBarButtonStyle.Plain, target: nil, action: nil)
}
}
}
let done = Button.Done("Done")
let doneButton = done.toUIBarButtonItem()
Just to be complete I added a third case to deal with the third Button enum case. The editor won't mind.
William Hartvedt Skogstad
5,857 PointsWilliam Hartvedt Skogstad
5,857 PointsThanks! I will most certainly have to watch the enum videos some more.. :)
Dan Hickey
3,016 PointsDan Hickey
3,016 PointsMy code will not pass the challenge, not sure what I am doing wrong?
enum Button { case Done(title: String) case Edit(title: String)
func toUIBarButtonItem() -> UIBarButtonItem { switch self { case .Done(let value): return UIBarButtonItem( title: value, style: .done, target: nil, action: nil) case .Edit(let value): return UIBarButtonItem( title: value, style: .plain, target: nil, action: nil) } } } let done = Button.Done(title: "Save") let doneButton = done.toUIBarButtonItem()