Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial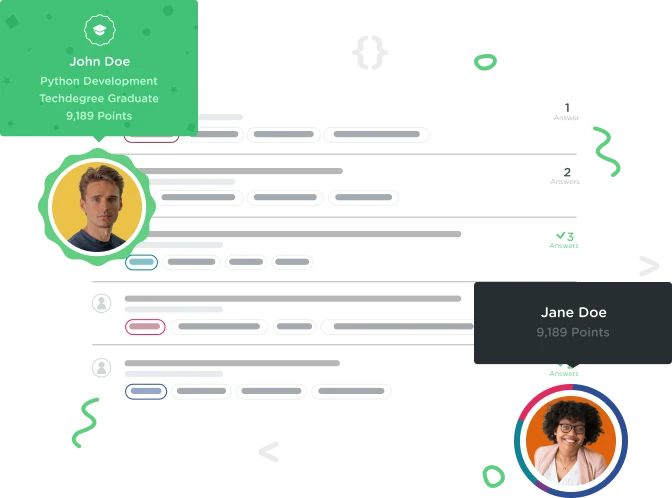

Nafeez Quraishi
12,416 Pointslast_first giving undefined method slice error
I wrote the method slightly differently than what is shown on the tutorial and i am getting undefined method error. Could someone please point what is wrong with the below way-
def last_first
last_first = last_name
last_first += ", "
last_first += first_name
last_first += " "
if !middle_name.nil?
middle_name = middle_name.slice(0, 1)
last_first += middle_name
last_first += "."
end
end
Error i'm getting is:
AddressBook/Contact.rb:37:in `last_first': undefined method `slice' for nil:NilClass (NoMethodError)
2 Answers
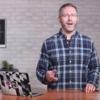
Jay McGavren
Treehouse TeacherIf it's saying undefined method 'slice' for nil:NilClass
, that means middle_name
is getting set to nil
. I think this is happening because your variable names are getting confused with your method names.
Below is my updated version of your code, with comments added.
class Contact
# I don't have your full code, so I created this
# simplified version. Hopefully this is correct.
attr_accessor :last_name, :first_name, :middle_name
def last_first
# It's not a good idea to use a variable with the same
# name as a method. Sometimes it will work, but it risks
# accidentally calling a method when you're just trying
# to assign to the variable.
# I renamed the variable.
full_name = last_name
full_name += ", "
full_name += first_name
full_name += " "
if !middle_name.nil?
# Here, I'm pretty sure your code IS calling the
# middle_name= method and overwriting it with just
# the initial. I changed the variable name here, too,
# and that seems to have fixed it.
middle_initial = middle_name.slice(0, 1)
full_name += middle_initial
full_name += "."
end
end
end
contact = Contact.new
contact.last_name = "Last"
contact.first_name = "First"
contact.middle_name = "Middle"
puts contact.last_first # => Last, First M.

Nafeez Quraishi
12,416 PointsThanks a lot Jay !