Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial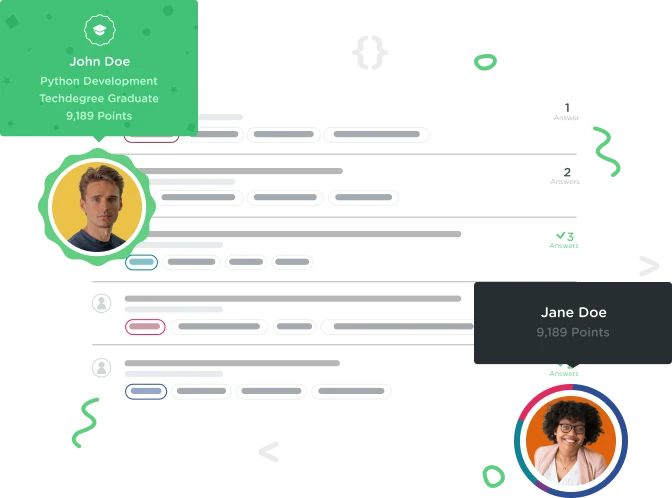
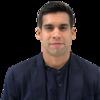
Johnny Garces
Courses Plus Student 8,551 PointsLet variables and block scope
I have an example code that has two let
variables- one on the outer function scope, and one inside a block-scope within the if-statement.
Why does createStudent('Ken') print "James"? Shouldn't it print "Ken"?
(function (){
let student = {name: 'James'};
function createStudent(name){
if(true){
let student = {name: name};
}
return student;
}
console.log(createStudent('Ken'));
console.log(student);
})();
2 Answers

Tray Denney
Courses Plus Student 12,884 PointsThe problem seems to be that you are trying to create a second student variable. You can reassign the variable by removing the let in your if statement.
(function (){
let student = {name: 'James'};
function createStudent(name){
if(true){
student = {name: name};
}
return student;
}
console.log(createStudent('Ken'));
console.log(student);
})();
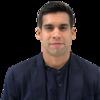
Johnny Garces
Courses Plus Student 8,551 PointsYes, I am trying to create a second student variable that's only scoped to the if-statement. Why then does it get assigned to "James"? thx for ur response btw- still confused with es6 variables. I'm surprised Guil from Treehouse didn't go over block-scope, an important concept in es6.
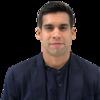
Johnny Garces
Courses Plus Student 8,551 Pointsyup that did the trick :) is it because if you return a value outside of its block-scope, then it will look up its scope-chain and reference the outer-scope's value? (in this case, the outer-scope being the function scope...)?
thanks again!

Tray Denney
Courses Plus Student 12,884 PointsGlad it works now! If you haven't already, check out the MDN docs for Block-Scope variables: https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Statements/let
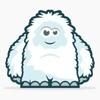
ywang04
6,762 Points(function (){
let student = {name: 'James'};
function createStudent(name){
if(true){
let student = {name: name};
//this student variable with let keyword which has it's own block scope.
}
return student;
//this returned student variable is not the same as above one due to block scope, actually
//it refers to {name: 'James'} using scope chain
}
console.log(createStudent('Ken'));
console.log(student);
})();
To meet your requirement
(function (){
let student = {name: 'James'};
function createStudent(name){
if(true){
let student = {name: name};
return student; //put return statement in "if" block scope
}
}
console.log(createStudent('Ken'));
console.log(student);
})();
Tray Denney
Courses Plus Student 12,884 PointsTray Denney
Courses Plus Student 12,884 PointsIn that case it is because you are returning the wrong student. Try: