Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial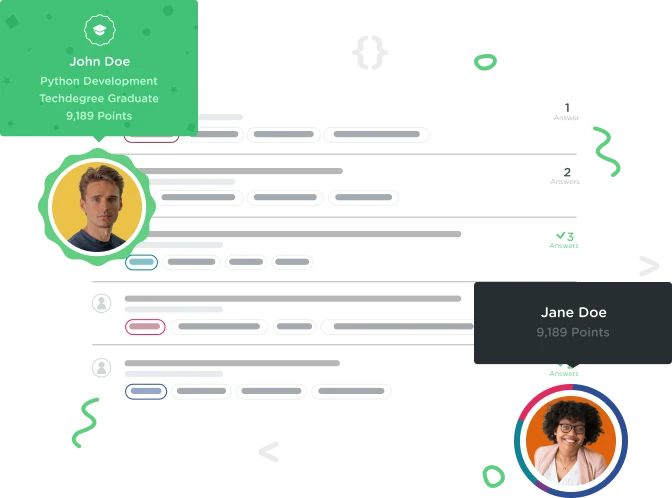

Raj Ku
Courses Plus Student 2,561 PointsLets assume the tableView has more than 1 section
Lets assume the tableView has 3 sections, and we want the items of the array to be distributed on these sections.
The first section will have the first 3 items of the array, the second will have 2 items, and the third will have the remaining items. What methods should we implement in this case?
I'd appreciate if I could find a solution for this answer because i've been struggling a lot and couldn't figure out how.
2 Answers
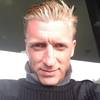
Rasmus Rønholt
Courses Plus Student 17,358 PointsHi Raj
It really does depend on where you get your data from - or rather in which form ... how the sections are defined. But the following is an example of where the data is an array of arrays - one for each section.
var objects:[[AnyObject]] = []
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return objects.count
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
return objects[section].count
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier("Cell", forIndexPath: indexPath)
let data = objects[indexPath.section][indexPath.row]
// do stuff with cell based on data
return cell
}
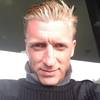
Rasmus Rønholt
Courses Plus Student 17,358 PointsNow if the scenario is exactly like you describe; all items in same array, first section 3 items, then 2 and then the rest:
var objects:[AnyObject] = []
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
return 3
}
override func tableView(tableView: UITableView, numberOfRowsInSection section: Int) -> Int {
switch section {
case 0: return 3
case 1: return 2
case 2: return objects.count - 5
default: return 0
}
}
override func tableView(tableView: UITableView, cellForRowAtIndexPath indexPath: NSIndexPath) -> UITableViewCell {
let cell = tableView.dequeueReusableCellWithIdentifier(“Cell”, forIndexPath: indexPath)
var row = indexPath.row
switch indexPath.section {
case 1: row += 3
case 2: row += 2
default:()
}
let data = objects[row]
// do stuff with cell based on data
return cell
}
You could implement a struct or something to dynamically calculate rows in sections - but this will work.
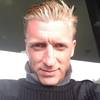
Rasmus Rønholt
Courses Plus Student 17,358 PointsBtw there should be checks to see if there are enough items to fill all sections... you could do
override func numberOfSectionsInTableView(tableView: UITableView) -> Int {
switch objects.count {
case 0...3: return 1
case 4...5: return 2
default: return objects.count
}
}
and similar for the other functions. But then you would have to consider whether or not to display headers for empty sections etc etc.
Anyways let me know if you run in to specific problems. Best of luck :)