Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial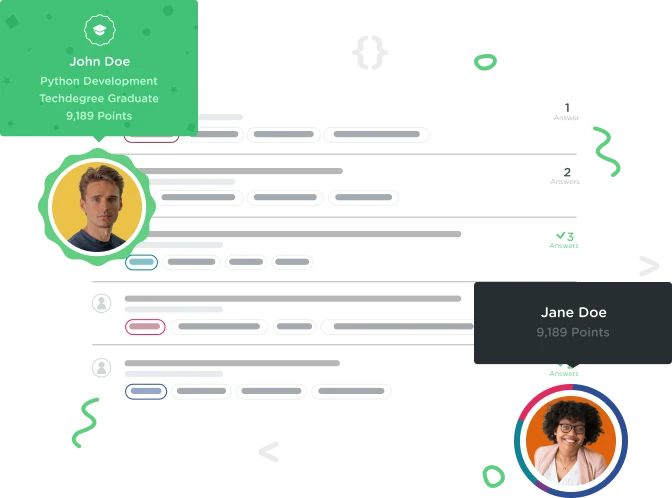

Bashir Orfani
13,170 PointsLet's make all Board instances
Let's make all Board instances iterable so we can loop through their cells attribute. Inside the Board class, define an iter method that yields the cells. If you need help, refer back to the "Emulating Builtins" video.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
class TicTacToe(Board):
def __init__(self, width=3, height=3): # the defaults go here instead
super().__init__(width, height)
3 Answers
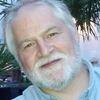
Jeff Muday
Treehouse Moderator 28,722 PointsWhich part of this are you having trouble with?
Part 1 is a question about subclassing the Board Object and calling its super().__init__()
method
Part 2 is a question about implementing an __iter__()
method on the new class.

Kevin LG
5,641 PointsYou can also streamline your code by skipping the for loop, and using yield from self.cells.
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = []
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
def __iter__(self):
yield from self.cells #<------the for loop obviously works,
#but by using yield from you save a line of code to be typed.
class TicTacToe(Board):
def __init__(self):
super().__init__(width = 3, height = 3)

Benyamin Kohanchi
Courses Plus Student 2,342 PointsHey Bashir, this is how you do the second part of the challenge.
Kenneth is asking you to create a method that iterates (using __ iter __) over self.cells in the Class Board. This is what the entire code should look like:
class Board:
def __init__(self, width, height):
self.width = width
self.height = height
self.cells = [] # <---- This is what we are trying to iterate over in Challenge 2
for y in range(self.height):
for x in range(self.width):
self.cells.append((x, y))
def __iter__(self): #<----- Second challenge asked us to define __iter__
for cell in self.cells:
yield cell
class TicTacToe(Board): # <---- First challenge asked to make this class
def __init__(self):
super().__init__(width=3, height=3)
Basically, we defined __ iter __ and made it loop over cells....
def __iter__(self):
for cell in self.cells:
...and then we yielded (or returned what we wanted --> without <--- ending the program) each iterable item in the list of self.cells.
yield cell
If you have any questions, comment them down below and ill try to answer them as soon as I can. Good luck!