Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial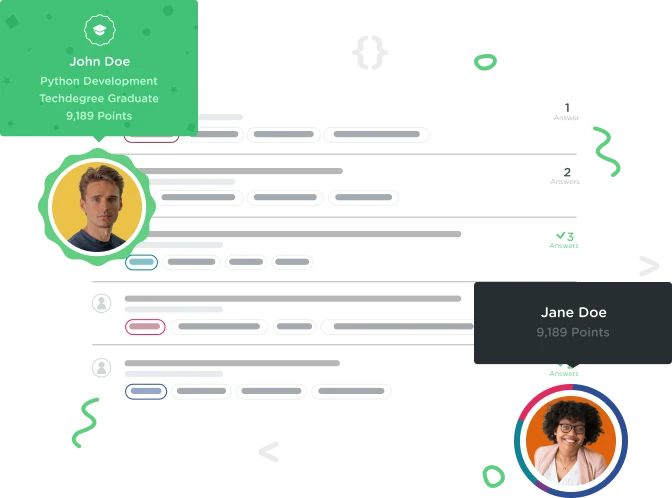
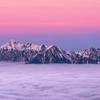
isaacrichardson
14,313 PointsLet's use __str__ to turn Python code into Morse code! OK, not really, but we can turn class instances into a representa
Let's use str to turn Python code into Morse code! OK, not really, but we can turn class instances into a representation of their Morse code counterparts. I want you to add a str method to the Letter class that loops through the pattern attribute of an instance and returns "dot" for every "." (period) and "dash" for every "" (underscore). Join them with a hyphen. I've included an S class as an example (I'll generate the others when I test your code) and it's __str_ output should be "dot-dot-dot".
The code is working, what's wrong with it
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __str__(self):
morse = []
for i in self.pattern:
if i == '.':
morse.append("dot")
if i == "_":
morse.append("dash")
return "-".join(morse)
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
12 Answers
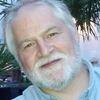
Jeff Muday
Treehouse Moderator 28,716 PointsI pasted in just the str() method you wrote and worked perfectly. Nice job!
I would try re-doing the challenge. It's hard to say where miscommunication between browser, editor, and server goes wrong without examining the logs (which I don't have access to). It can sometimes be as trivial as substitution of space and tab characters or unexpected "kerning" of fonts where indents visually look fine, but Python rules differently.
I feel confident that it should work if other challenges were working for you before.
def __str__(self):
morse = []
for i in self.pattern:
if i == '.':
morse.append("dot")
if i == "_":
morse.append("dash")
return "-".join(morse)
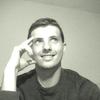
Leonard Foster
14,806 PointsI feel lost in this course, anyone else feel it could be a little more clear?
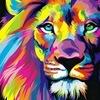
Anthony Parker
1,454 PointsYes, very unclear on the learning objective.

Mitchell Beck
5,836 PointsGenerally, Treehouse courses are great, this is the first one that I am having trouble understanding!

Roger Perez
8,676 PointsOMG I feel a little better that i'm not the only one that feels that way. I felt like my learning was going great until I got to OOP and it thru me off bad. I'm very curious, how are you all feeling about it now that some time has passed? Do you feel like you have a better understanding. I have been loving learning python so far but this threw me off so much that I feel a drop in motivation. Pushing through it tho! Really looking forward to hearing how you guys feel on it now.

RWS Admin
19,205 PointsI am with you all. I am really struggling with this course. I've been watching some youtube tutorials on python and that's been helping a bit. Hopefully they redo this course with one of the newer instructors.

Zachary Ponder
6,704 PointsI feel like I NEVER want to take another one of Kenneth Love's courses.

Laurens Salcedo Valdez
3,117 PointsFelt the same way for some time, I suggest for anyone else having this issue to tackle the subject in a different way. Try to divide the subject in different blocks, look at the teacher's note and try to understand why certain challenges are resolved in a certain way. It took me some time to get a hang of it, but as Kenneth said it is a tricky subject.
Don't lose hope! Once you get through this you will feel so much better and you will be able to continue your journey of learning Python.

Aliaksandr Khvastovich
7,864 Pointsyep, it is like how did I get here from functions...

Flamie Mushawatu
4,135 PointsHey guys i know this is really old now , but if anyone found an easier way to understand this ,i would really appreciate a hand...😓

Yoon Choi
1,649 PointsI agree, it so confusing. We wish someone else can re-teach the OOP. PLEASE!

Titose Chembezi
3,361 PointsI think OO module should be redone. I was fine with the previous modules
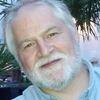
Jeff Muday
Treehouse Moderator 28,716 PointsThe string method "join()" does a joining of list items and separating each with the string supplied and returns a string. This is a little tricky at first if you are coming from a JavaScript or Ruby background where join() works differently.
Here's a simple Python example using mylist and mystring.
mylist = ['How','are','you']
mystring = "$".join(mylist)
After running the code, the variable "mystring" will contain "How$are$you".
Notice that the dollar-sign character is imposed in between each list element of mylist.
In the context of the code challenge
morse_list = ['dot','dash','dot']
morse_string = "-".join(morse_list)
After the code is run, the variable morse_string will contain "dot-dash-dot"

Michal Bednář
3,116 PointsThis OOP module is terrible. Please remake it with somebody else. All the courses in this track with previous two teachers were amazing but this is just terrible.

devin leyba
7,209 PointsI agree, needs to be explained better
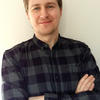
Arturas Budreika
Python Development Techdegree Student 5,464 PointsThis is so bad... I am watching material over and over and still do not get it...

Cheo R
37,150 PointsYou might have a spacing issue, I copied and pasted your exact code and it passed.

anthony pizarro
1,983 Pointswhat does the '-'.join(morse) do?
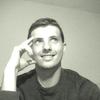
Leonard Foster
14,806 Points@anthony pizarro: joins a list of strings together with a "-" between. ie ["dot", "dot", "dot"] becomes "dot-dot-dot"
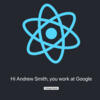
drew s
Python Development Techdegree Graduate 19,491 PointsI agree with all of you.. I was enjoying each course in techdegree for Python and when I reached OOP course I started to lose interest. I thought I was the only one who's struggling in the course.

noel acio
1,470 Pointsglad to read your comments and how you guys feel about OO,.