Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial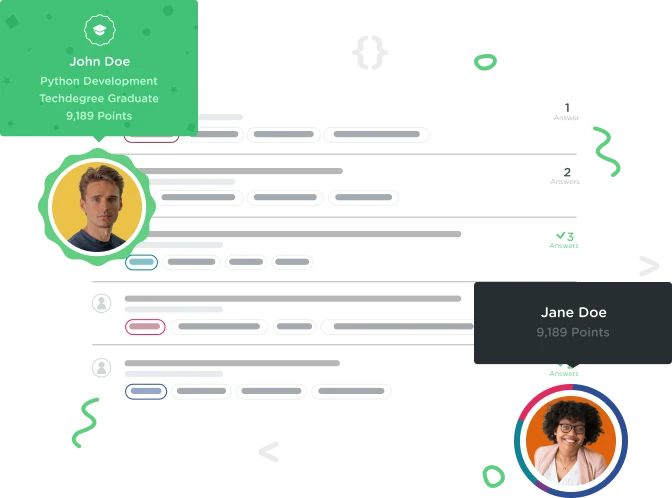

Christopher Wommack
3,983 PointsLetter_game.py
import random
words = [
'apple',
'banana',
'orange',
'cocconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon',
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == 'q':
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter")
continue
elif not guess.isalpha():
print("You can only guess letters")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word):
print("You win The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it My secret word was {}".format(secret_word))
I'm getting an invalid syntax on while true and I tried to follow the video with kenneth
4 Answers
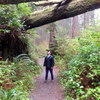
Garrett Guevara
11,331 PointsHi Christopher,
The two things I noticed were that you didn't close the square brackets in your words array. You also forgot to close a parenthesis for len(list(secret_word)). I corrected them and was able to run it. I hope this helps!
import random
words = [
'apple',
'banana',
'orange',
'cocconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == 'q':
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter")
continue
elif not guess.isalpha():
print("You can only guess letters")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it My secret word was {}".format(secret_word))
Happy coding!
Garrett
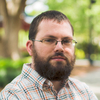
Kenneth Love
Treehouse Guest TeacherIf you keep going through the course, we correct a bug that's in this version. Specifically, this bit of logic:
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
What happens if a word has a repeated letter? good_guesses
isn't going to get multiple copies of the letter so the length of that list and the list made from the chosen word won't ever sync up.
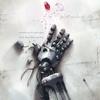
Daniel Ruggiero
1,224 PointsThanks, I was having this problem too

Christopher Wommack
3,983 PointsI have got my program to work to a point I can play the game but it won't tell me when I have won and I can't get a proper exit besides at start
import random
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == 'q':
break
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
else:
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You\'ve already guessed that letter")
continue
elif not guess.isalpha():
print("You can only guess letters")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win The secret word was {}".format(secret_word))
exit()
else:
bad_guesses.append(guess)
else:
print("You didn't get it My secret word was {}".format(secret_word))
break

Christopher Wommack
3,983 PointsI still can't get it to say I won