Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial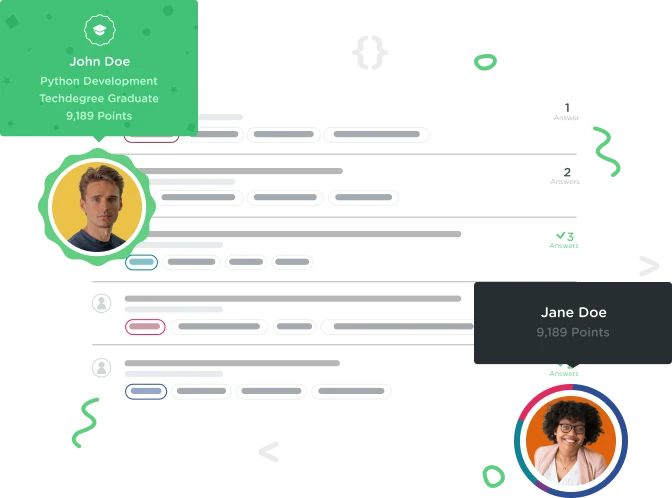

Jason Whitaker
12,252 Pointsletter_game.py just runs in a loop. doesn't quit.
import random
# make a list of words
words = [
'apple',
'banana',
'orange',
'coconut',
'strawberry',
'lime',
'grapefruit',
'lemon',
'kumquat',
'blueberry',
'melon'
]
while True:
start = input("Press enter/return to start, or enter Q to quit")
if start.lower() == 'q':
break
# pick a random word
secret_word = random.choice(words)
bad_guesses = []
good_guesses = []
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
# draw guessed letters, spaces and strikes
for letter in secret_word:
if letter in good_guesses:
print(letter, end='')
else:
print('_', end='')
print('')
print('Strikes: {}/7'.format(len(bad_guesses)))
print('')
# take a guess
guess = input("Guess a letter: ").lower()
if len(guess) != 1:
print("You can only guess a single letter!")
continue
elif guess in bad_guesses or guess in good_guesses:
print("You've already guessed that letter")
continue
elif not guess.isalpha():
print("You can only guess letters!")
continue
if guess in secret_word:
good_guesses.append(guess)
if len(good_guesses) == len(list(secret_word)):
print("You win! The word was {}".format(secret_word))
break
else:
bad_guesses.append(guess)
else:
print("You didn't guess it! My secret word was {}".format(secret_word))
# print out win/lose
3 Answers
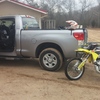
Ryan Ruscett
23,309 PointsThat's because you are doing a
while True:
Let me ask you this. While what is true? As far the computer knows as long as True it is going to keep going. Nothing tells me to quite.
A while loop is used in the instance something will run for x amount of time and I don't know what x is going to be. You have the first while True but you also have:
while len(bad_guesses) < 7 and len(good_guesses) != len(list(secret_word)):
You don't do a while loop for something that you know how many times it's suppose to run. While length of bad_guess is less than seven. How about if len of bad_guess is less than 7. No need to while loop it. You are entering a loop inside of a loop. But not a loop that ever ends. The first loop doesn't end and the second loop runs as long as something needs to happen.
You should make the first while loop depending on a variable. Say "run = True" Now I am going to run until that value get's turned false.
run = True
While run"
blah blah blah
Then change the second while to an if statement. This way it kicks it back to the while loop to run again. where as the value of run is still true keep going.
Does that make sense? If not let me know and I can try and help further or rephrase it.
Thanks!

Jason Whitaker
12,252 PointsI just rewatched the video and double checked my code. It is correct per the video. I runs now and I didn't change a thing on it. There must have been something wrong with Workspaces. Also, if using a while construct is not considered best practice then why is the code presented that way in the video?
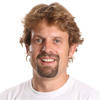
Mark Southcombe
3,361 PointsThe code only works if the word has no repeating letters, it is not correct!
When it compares the good_guesses to secret_word it doesn't take into account that there may be repeating letters.
Basically the code the instructor wrote is floored!! Unless the word you are guessing has no repeating letters.