Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial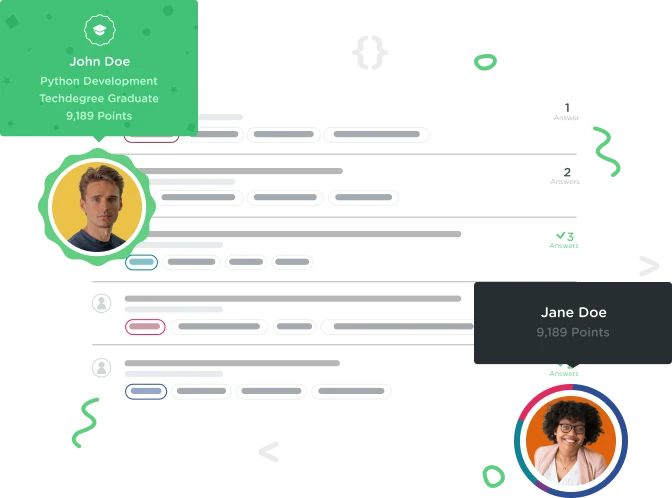

Chris Braun
1,606 PointsLevel construction parameters
Is there a reason why the constructor of Level doesn't take both an array of invaders and an array of towers as parameters? Wouldn't this be clearer/simpler? Is it just to demonstrate how the Towers property of the Level object can be initialized at the same time as the construction of the Level object, or is there a deeper rationale behind it? Thanks.
Adam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsAdam Fields
Full Stack JavaScript Techdegree Graduate 37,838 PointsYou can definitely pass an array of Towers, and also make them private:
Or you can set them after instantiation:
The original example was demonstrating Object Initializers.
Using the initializer syntax makes writing your class easier with less boilerplate (you don't need to write the parameterized constructor with a bunch of assignments). On the other hand, that's not how we do it in Java and JavaScript, so it's one of those C# tricks you have to remember when switching languages.
You should pick one way or the other, though. Setting one property in the constructor, and initializing the other using a an initializer is confusing, in my opinion.