Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial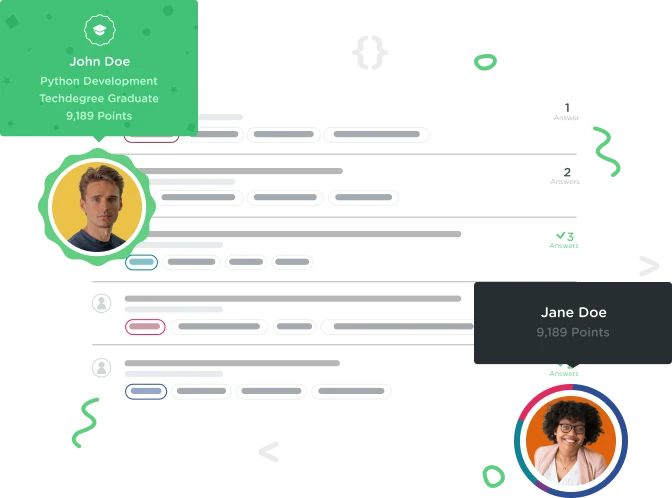
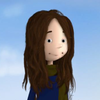
Maximiliane Quel
Courses Plus Student 55,489 PointsLightbox won't pull in video with stored variable
I built a lightbox that shows videos instead of photos in the overlay. I am using an unordered list of images inside anchors as triggers for the overlay.
<body>
<div id="page-container">
<div id="content-container" class="group">
<section id="main">
<h1>Playback Time!</h1>
<p>this is a simple test page to demonstrate a sidebar video playing in overlay mode</p>
</section>
<section id="sidebar">
<p class="sectionTitle">Videos</p>
<!-- VIDEO TRIGGERS -->
<ul id="videoList">
<li>
<a href="https://www.youtube.com/watch?v=CLRPH3nFf8w">
<img src="http://i1381.photobucket.com/albums/ah236/mxquel/92419208-c7b4-4591-a3bd-95ea9b732924_zps473abb42.png" alt="Thumbnail of a Video" />
</a>
<p>description or title of the video</p>
</li>
<li>
<a href="https://www.youtube.com/watch?v=cmSbXsFE3l8">
<img src="http://i1381.photobucket.com/albums/ah236/mxquel/92419208-c7b4-4591-a3bd-95ea9b732924_zps473abb42.png" alt="Thumbnail of a Video" />
</a>
<p>description or title of the video</p>
</li>
</ul>
</section>
</div>
</div>
</body>
The href of the anchor is the same link that I want to use as a file-url in the jwplayer settings so I am saving it into a variable. I am having trouble replacing the hardcoded link for a video with the stored variable of the link.
//creates the overlay
var $overlay = $('<div id="overlay"></div>');
var $player = $('<div id="target" class="jwp"></div>');
//adds player to the overlay
$overlay.append($player);
//adds the overlay to the page
$('body').append($overlay);
// Captures the click even on a video link
$('#videoList a').click(function(event){
event.preventDefault();
var triggerLink = $(this).attr('href');
// Show the overlay
$($overlay).show();
});
//sets up the video player
var jwp;
var videoLink = 'https://www.youtube.com/watch?v=CLRPH3nFf8w';
var videoTitle = 'Keone & Mariel Madrid :: "Cups (When I\'m Gone)" by Anna Kendrick :: Urban Dance Camp';
jwp = jwplayer('target').setup({
file: videoLink,
title: videoTitle,
displaytitle: 'false',
width: '60%',
controls: 'true',
aspectratio: '16:9',
autostart: 'false',
stretching: 'fill'
});
var quality = jwplayer('target').setCurrentQuality(5);
As long as the link is hardcoded it is fine however when I change the code to have triggerLink = videoLink it doesn't work. Where the video was playing nicely in the overlay I only get the blank overlay.
I don't understand why. When I log videoLink in the console it is exactly the same as the hardcoded link.
I would really appreciate if someone could help me identify the issue
2 Answers
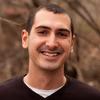
tihomirvelev
14,109 PointsThe problem is because of the scope where the variables are defined.
You want you var videoLink to be assigned with the value of triggerLink but because triggerLink is inside a function therefore is local to that function and you can't assigned it to the var videoLink (which is outside that function).
All the code below the comment //sets up the video player has to go inside the function, right below var triggerLink.
Also don't forget to make your own jwplayer account and add the media library to your html code. Look here for information: http://support.jwplayer.com/customer/portal/articles/1406725-youtube-video-embed.
I've tested the things and they worked on my machine, although it's obvious that you have more work to the code before it looks and performs good. :)
Good luck!
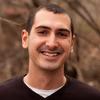
tihomirvelev
14,109 PointsThis happened with me too but I was lazy to search why. :)
Now I found this topic: http://support.jwplayer.com/customer/portal/questions/8085212-error-cannot-read-property-replace-of-undefined
It seems that is a bug with the latest version of JW Player. One of the comments suggest to return to the previous version and this fixes the problem but you'll have to test that on your own.
Good luck!
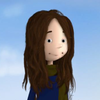
Maximiliane Quel
Courses Plus Student 55,489 Pointsaha I never considered that this could be a bug. thanks!
Maximiliane Quel
Courses Plus Student 55,489 PointsMaximiliane Quel
Courses Plus Student 55,489 PointsThanks. That's great!
I now set up the player in a function at the top so I can pass in the video link and title as an argument when I call it inside the click event.
Thanks for the link. I have set up my account so that's all good.
Yes still a few things to do before it is all done :0).
Thanks again for your help!
Maximiliane Quel
Courses Plus Student 55,489 PointsMaximiliane Quel
Courses Plus Student 55,489 PointsSorry but I have a follow up question. If you don't mind. Why does the code blow not insert the video when I click on the other link? what am I missing?
I can click on either link and it loads just fine. when I click the closing button it does close. but when I click on any of the links again the overlay shows up with the button but no player and the button is dead.
here is a codepen
I don't really understand what I am doing wrong. I would really appreciate if you had a look.